Preface
The Internet of Things (IoT) is a complex, interconnected system-of-systems composed of different hardware devices, software applications, data standards, communications paradigms, and cloud services. Knowing where to start with your own IoT project can be a bit daunting. Programming the Internet of Things is designed to get you started on your IoT development journey and shows you, the developer, how to make the IoT work.
If you decide to stay with me through this book, you’ll learn how to write, test, and deploy the software needed to build your own basic, end-to-end IoT capability.
Who Is This Book For?
Programming the Internet of Things is, at its core, a book about building IoT solutions—from device to cloud.
This book was primarily written as a teaching guide for my Connected Devices course at Northeastern University, and for any students interested in learning how to program IoT solutions. While it’s structurally focused on assisting students and practitioners, it can also be helpful for those interesting in learning more about IoT concepts and principles.
Throughout the book, you will find step-by-step guidelines for building your own end-to-end IoT capability, with exercises within each chapter that build on one another to help you cement your knowledge of the IoT. If you’re more interested in the concepts, however, that’s perfectly fine! You can learn about the what and why but move quickly through the how and skip the exercises, if you’d prefer.
As an educator and a consultant, I’ve structured the content so it can be used as a road map for teaching introductory IoT programming courses, with the intent of stepping through key concepts and gradually building a base of knowledge in this important area. Whether you’re a college-level instructor or a student looking to develop your skills in the IoT, I hope you’ll find this book helpful in building your course and knowledge.
Lastly, I leave most of the specific instructions and details to the existing specifications and open source APIs. While some parts of the book might serve as a high-level reference, a majority of the content here focuses on helping you leverage such information to build the solution you need. We’re fortunate to have access to well-written protocol specifications and a vibrant open source community, and I’m grateful to those who have championed these efforts.
To the Programmer
If you’re embarking on your own IoT learning journey as a practitioner, I assume you’re mostly interested in expanding your skill set. Perhaps you’ve witnessed the growth of IoT opportunities and want to be part of this important technology evolution. Over the years, I’ve found the step-by-step, “build from the ground up” approach to implementing integrated and open IoT solutions to be most helpful in understanding the complexities of this area, and so I follow this model throughout the book.
The programming examples you’ll encounter here are constructed from my own journey in learning about the IoT, and many have evolved from lab module assignments in the graduate-level Connected Devices course I teach as part of Northeastern University’s Cyber Physical Systems program.
Each chapter and exercise set builds on the one before, so if you are just starting out with the IoT and are unfamiliar with how an end-to-end IoT system comes together through software, I’d recommend walking through each as-is, working through each exercise in the order given. It’s best to consider application customizations only after you’ve mastered each chapter.
If you’re an experienced programmer and are using this book as a reference guide, you may find you can skip over some of the basic development environment setup procedures and simply skim through some of the programming exercises; however, I do recommend you work through the requirements specified in each, even if you choose to use your own design.
To the Instructor
The contents that underpin this book have been used successfully in my graduate-level Connected Devices course for the past few years. This book is the formalization of these lecture notes, presentations, examples, and lab exercises and is structured in much the same way as my course.
I originally designed the class as an introduction to the IoT, but with student input and suggestions from my teaching assistants, it quickly morphed into a project-oriented software-development course. It’s now one of the final required classes for students wrapping up their master’s degrees in the Cyber Physical Systems program. The goal of the course is to establish a strong baseline of IoT knowledge to help students move from academia into industry, bringing foundational IoT skills and knowledge into their respective organizations.
This book is structured so it can be used as a reference guide or even as a major component of a complete curriculum, to help you with your own IoT-related course. The content is focused on constructing an end-to-end, open, and integrated IoT solution, from device to cloud, using a “learn as you build” approach to teaching. Since each chapter builds on the preceding one, you can use this book to guide your students in building their own platform from the ground up.
You can remain up to date with the online exercises by reviewing the Programming the IoT Kanban board.1 Other relevant content useful for teaching and explaining some of the concepts in this book can be found on the book’s website.
To the Technology Manager or Executive
The contents of this book should help you better understand the integration challenges inherent to any IoT project and should provide insight into the skill sets your technology team(s) will need to succeed across your IoT initiatives.
If you’re part of this group, my assumption is that you’re mostly concerned with understanding this technology area as a whole—its integration challenges, dev teams’ setup requirements and needs, team skill sets, business user and stakeholder concerns about the IoT, and the change-management challenges you may encounter as you embark on your organization’s IoT journey.
For technology executives and managers, you don’t need to implement the exercises yourself, but it will be helpful to read through the entire book so you understand the challenges your team will likely encounter.
For business stakeholders interested mostly in understanding what the IoT entails, I recommend reading—at a minimum—the overview section at the start of each chapter and then focusing on the final chapter, which discusses a handful of practical cases, scenarios, and implementation suggestions.
What Do I Need to Know?
Although the exercises in this book assume that you have some experience writing software applications in Python and Java, most do not require sophisticated programming skills or a formal computer science background. Most exercises do, however, require that you’re comfortable developing code in an integrated development environment (IDE); reading, writing, and executing unit tests; running applications from the command line; and configuring Linux-based systems via a shell-based command line.
If you intend to complete the optional exercises listed in the Kanban board or at the end of some of the chapters, you’ll need to be comfortable building Python and Java applications from scratch with little to no guidance.
All exercises are preceded by a target state design diagram for the specific task at hand that details how any new logical components you build should work with the existing components you’ve already developed. Most are simple block diagrams that show the basic relationships among the components of the application.
Note
Many of the diagrams are not designed to fit into a specific documentation methodology and show only a high-level view of the components and their basic interactions. Some diagrams do require additional specificity, and in such cases I include one or more Unified Modeling Language (UML)–based class diagrams to clarify the intention of the design.2
How Is This Book Arranged?
This book will take you through building an end-to-end, full stack, and integrated IoT solution using various open source libraries and software components that you’ll build step by step. Each component will be part of the larger system, and you’ll see how the components interconnect with and map into an end-state architecture as you work through each chapter’s exercises.
In each chapter, I’ll provide a brief introduction to that chapter’s topic along with some helpful background material, which will include some pertinent definitions. I’ll also summarize why the topic is important and what you can expect to learn, and that will be followed by programming exercises relevant to the topic. Many chapters end with additional exercises you can choose to implement as well, further cementing your knowledge of the chapter’s topic.
I have grouped like chapters together and have used this scheme to establish the four parts of the book: Part I, “Getting Started”; Part II, “Connecting to the Physical World”; Part III, “Connecting to Other Things”; and Part IV, “Connecting to the Cloud”. I’ll discuss each part and chapter in a bit more detail here and then provide some background on the IoT itself.
Each part or chapter begins with a haiku that attempts to capture the essence of what you’ll learn and some of the challenges you’ll likely encounter. How did this come to be? In my early days as a software developer, one of the teams I worked with had a policy: if you committed code that caused the nightly build to break, you had to write a haiku related to the issue and email it to everyone on the team. While you probably won’t encounter many broken nightly builds as you work through the exercises in this book, you should feel free to write your own haiku as you make and learn from your mistakes!
Part I, “Getting Started”
In this section, we build our initial foundation for IoT development. You’ll start by creating a development and testing environment and then wrap up the section by writing two simple applications to validate that your environment is working properly.
-
Chapter 1, “Getting Started” is the longest chapter in the book. It lays the foundation for your end-to-end solution and will help you establish a baseline of IoT knowledge. It will also guide you in setting up your workstation and development environment so you can be productive as quickly as possible. In this chapter, I cover some basic IoT terms, create a simple problem statement, define core architectural concepts, and establish an initial design approach that I’ll reference as the framework for each subsequent exercise.
Note
The IoT consists of a plethora of heterogeneous devices and systems, and there are many tools and utilities available to support development and system automation. I use open source tools and utilities throughout the book. These represent a small subset of what is available to you and shouldn’t be taken as carte blanche recommendations; you may have your own preferences. My goals are simply to keep the content well-bounded and inform a generalized development and automation approach that will help you implement the exercises successfully.
-
Chapter 2, “Initial Edge Tier Applications” covers setting up your development environment and your approach to capturing requirements and then moves into coding. Here you’ll create your first two IoT applications—one in Python and the other in Java. These are quite simple but set the stage for subsequent chapters. Even if you’re an experienced developer, it’s important to work through the exercises as given so we’re working from the same baseline going forward.
Part II, “Connecting to the Physical World”
This section covers a fundamental characteristic of the IoT—integration with the physical world. While the exercises focus on simulation and hardware emulation, the principles you’ll learn—how to read sensor data and trigger an actuator (virtually)—will be helpful should you decide to use real hardware in the future.
-
Chapter 3, “Data Simulation” explores ways you can collect data from the physical world (sensing) and trigger actions based on that data (actuation) using simulation. You’ll start by building a set of simple simulators, which you’ll continue using throughout each subsequent exercise. While very basic in design, these simulators will help you better understand the principles of collecting data and using that data to trigger actuation events.
-
Chapter 4, “Data Emulation” expands the simulation functionality you developed in Chapter 3 to emulate sensor and actuator behavior. This chapter remains centered in the virtual world through the use of an open source hardware emulator you can run on your development workstation.
-
Chapter 5, “Data Management” discusses telemetry and data formatting, including ways to structure your data so both humans and machines can store, transmit, and understand it easily. This will serve as the foundation for your interoperability with other “things.”
Part III, “Connecting to Other Things”
This is where the rubber meets the road. Part III focuses on integration across devices: to be truly integrated, you’ll need a way to get your telemetry and other information from one place to another. You’ll learn about and utilize application layer protocols designed for IoT ecosystems. I’ll assume your networking layer is already in place and working, although I’ll discuss a few wireless protocols along the way.
-
Chapter 6, “MQTT Integration–Overview and Python Client” introduces publish/subscribe protocols—specifically, Message Queuing Telemetry Transport (MQTT), which is commonly used in IoT applications. I’ll walk through a select set of specification details and explain how you can begin building out a simple abstraction layer that allows you to easily interface with common open source libraries, beginning with Python.
-
Chapter 7, “MQTT Integration–Java Client” continues to build on your knowledge of MQTT by digging into an open source library that allows you to connect your Java applications to an MQTT server. You’ll use this protocol in the exercises and tests you’ll run at the end of the chapter to integrate with the Python code you developed in Chapter 6.
-
Chapter 8, “CoAP Server Implementation” focuses on request/response protocols—specifically, the Constrained Application Protocol (CoAP), also commonly used in IoT applications. The big difference here is that you’ll start with Java and build a CoAP server using another open source library. Optional exercises are also provided to build a CoAP server in Python.
-
Chapter 9, “CoAP Client Integration” continues with CoAP but focuses on building the client code you’ll use to connect to your newly developed Java server. This client code, written in both Python and Java, will enable you to support device-to-device communications using CoAP as the protocol.
-
Chapter 10, “Edge Integration” centers on integration, enabling you to connect your two applications to each other using either MQTT or CoAP. I’ll include exercises for each protocol, which will help you decide which one may be most relevant for your solution. Much like Chapter 9, this chapter will require implementation work in both Python and Java.
Part IV, “Connecting to the Cloud”
Finally, at the “top” of the integration stack, you’ll learn how to connect all your IoT device infrastructure to the cloud by using your gateway application as a go-between for your cloud functionality and all your devices.
This section covers basic cloud connectivity principles and touches on various cloud services that can store, analyze, and manage your IoT environment. You’ll build the same simple cloud application across each platform.
-
Chapter 11, “Integrating with Various Cloud Services” discusses the key concepts of connecting your IoT solution into the cloud and explores various cloud-integration exercises you can implement using the MQTT protocol. Since there are many books and tutorials available for these platforms, I’ll simply review these capabilities rather than going into detail on building anything specific. The exercises help you choose which cloud platform you’d like to use in your own implementation.
-
Chapter 12, “Taming the IoT” examines the key enablers of an IoT solution and maps these into a few simple IoT use cases that I’ve found particularly helpful to preparing my Connected Devices course. I’ll cover the overall problem statement, expected outcome, and high-level notional design approach.
My hope is that this book’s approach will allow you to understand and create an integrated IoT system, end to end.
Some Background on the IoT
Here’s a brief summary of how the IoT got to this point.
Computing took a big step forward with the invention of the transistor in the 1950s, followed in the 1960s by Gordon Moore’s paper describing the doubling of transistors packed in the same physical space (later updated in the 1970s).3
With modern computing came modern networking, and the beginnings of the internet, with the invention of the ARPAnet in 1969.4 This led in the 1970s to new ways to chunk or packetize data using the Network Control Protocol (NCP) and Transmission Control Protocol (TCP) via the Internet Protocol (IP) and leveraging existing wired infrastructure. This was useful for industry, allowing electrical industrial automation to move down the path of centralized management of distributed, connected systems. Supervisory Control and Data Acquisition (SCADA) systems—ancestors of machine-to-machine (M2M) technologies that led to the IoT—emerged from their proprietary roots, and programmable logic controllers (PLCs)—initially invented just prior to the ARPAnet—evolved to take advantage of TCP/IP networking and related equipment standards.5
The 1980s introduced the User Datagram Protocol (UDP)6 and the birth of what many of us experienced as the early modern internet—the World Wide Web (WWW), invented in the late 1980s by Tim Berners-Lee.7
Note
This time period has also been an important enabler for what would eventually be known as the Industrial Internet of Things (IIoT), a subset of the IoT and an important part of the IoT’s evolution.
I’m sure you’ve noticed a common theme: a problem is followed by a technology innovation (often proprietary) to address the challenge, which then becomes standardized or is superseded by one or more standards, leading to wide adoption and further innovation.
This brings us to the era of the IoT. In the 1980s and early 1990s, the first connected devices emerged, including an internet-connected toaster demonstrated by John Romkey and Simon Hackett at Interop 1990.8 In 1991, users of the computer lab near the Trojan Room at the University of Cambridge set up a web camera to monitor the coffeepot— because who wants to make a trip only to find the pot empty?9
More devices followed, of course, and I’d guess even more were built and connected as experiments in college labs, dorms, homes, apartments, and businesses. All the while, computing and networking continued to become more inexpensive, powerful, and of course smaller. Kevin Ashton is widely believed to have coined the phrase Internet of Things in 1999, when he presented on the topic at Proctor & Gamble.10
Fast-forward to 2005, when the Interaction Design Institute Ivrea in Italy gave us the inexpensive, designed-for-novices Arduino single-board computer (SBC),11 opening the door for more people to build their own sensing and automation systems. Add easily accessible storage and the ability to analyze data through services reachable from anywhere on the internet, and you have the underpinnings of an IoT ecosystem: that is, a lot of individually unique things that can be connected to each other to serve a larger purpose.
Yet to view the IoT as a bunch of things that connect the physical world to the internet does not do the IoT justice. I believe the essence of the IoT, and a key driver behind its complexity, is heterogeneity: dissimilarity and wide variation among device types, features and capabilities, purposes, implementation approaches, supported protocols, security, and management techniques.
Complexity Redefined
So, what exactly is the Internet of Things? It’s a complex set of technology ecosystems that connect the physical world to the internet using a variety of edge computing devices and cloud computing services.
For the purposes of this book, we’ll use some slightly simplified definitions. Edge computing devices refers to the embedded electronics, computing systems, and software applications that either interact directly with the physical world through sensors and actuators or provide a gateway, or bridge, for those devices and applications to connect to the internet. Cloud computing services refers to computing systems, software applications, data storage, and other computing services that live within one or more data centers and are always accessible via the internet.
Going forward, I’ll refer to these two areas by their representative architectural tier—that is, Cloud Tier for cloud computing services, and Edge Tier for edge computing devices. Architectural tiers separate the key functionality of an IoT system both physically and logically—meaning, for example, that all sensing and actuation take place in the Edge Tier, and all long-term storage and complex analytics take place in the Cloud Tier.
Note
For those interested in a deeper study of architecture and related standards, there are many organizations actively participating in and publishing content related to these areas. A few to start with are:
-
The European Telecommunications Standards Institute (ETSI), a Europe-based standardization organization
-
The International Telecommunication Union (ITU), the United Nations’ agency for information and communication technologies
-
The Internet Engineering Task Force (IETF), a global internet standards body
-
The Industry IoT Consortium (IIC), an organization of businesses and organizations focused on the IIoT
The IIC has published a variety of useful documents. Of particular interest is the Industrial Internet Reference Architecture, which discusses a framework and common vocabulary for IIoT systems and has heavily influenced my thinking on the topic of IoT architecture.12
Creating Value
The real value in any IoT system is its ability to provide an improved or enhanced outcome via integration of the physical and logical worlds and the collection and analysis of time-series data.
Let’s take a simple example from an appliance-laden residential kitchen. If, for example, I can measure the inside temperature of a refrigerator every minute, I can determine how long the items stored in the refrigerator have been exposed to a given temperature. If I sample the inside temperature only once a day, I don’t have adequate detail to make that determination.
Of course, if other systems can’t understand the data I collect, it’s pretty much useless. Although individually each part may be unique and not dependent on another part, building integratable IoT solutions requires you, as the developer, to think carefully about how their design (and data) for each part of an IoT system might interact with other systems (and other developers).
Another key part of the value chain is scalability. It’s one thing to build a system that supports a handful of inputs but another thing altogether to handle thousands, millions, or even billions of inputs. Scalability—the ability of a system to handle as much or as little information as we want—is what gives the IoT its true power. For example, a scalable cloud system that supports the IoT is one that can handle a single gateway device sending it data, or thousands (or millions or billions) of inputs, without failing.
It’s probably clear by now that in building an integrated IoT system, you will need to deal with significant nuances at each step. You can’t expect plug ’n’ play or even consistent behaviors from the systems that send you data. What’s more, even if you try to write your code generically enough to function the same way from one hardware device to another, it might not always work across every platform.
Note
It’s not possible for one book to cover all specialized platforms, nor is it easy to write consistent, semi-low-level code at the device level that doesn’t need to be optimized for every device. While every device may have differences that we need to account for when we create (and test) our solutions, the code samples I provide in this book are portable (with some minor exceptions) and usable across most systems that can run a Java Virtual Machine or Python 3 interpreter.
Living on the Edge
With all the power and flexibility of the Cloud Tier, an IoT solution generally exhibits the greatest complexity at the edge, which is where most (and often all) of the system’s heterogeneity lives. The two categories of devices this book will focus on are constrained devices and gateway devices.
To grossly oversimplify: constrained devices have some power, communications, and processing limitations, whereas gateway devices generally do not.
Note
I’m leaving out the nomenclature of “smart devices” on purpose, since it’s becoming less clear how to best define “smart” versus “not-so-smart” devices.
One way to view a constrained device is as a low-power (sometimes battery-operated) SBC that either reads data from the environment (such as temperature, pressure, or humidity) or triggers a mechanical action (such as opening or closing a valve).
Note
The IETF provides detailed definitions and terminology for various “Constrained Devices or Nodes” in RFC 7228.13 It is not the intent of this book to alter these definitions in any way—I simply use the terms constrained device and constrained device app to separate the intended functionality of the constrained device from the gateway device, while implying the nature of each type of device (in short, that the former has more technical limitations than the latter).
A gateway device may also be implemented as an SBC but is much more powerful: it can communicate with many different constrained devices and has enough processing power to aggregate the data from these other devices, perform some analytics functions, and determine when (and how) to send any relevant data to the cloud for further storage and processing.
Figure P-1 envisions a notional IoT systems architecture that represents the relationships between these device types within the Edge Tier and the services and other functionality that live within the Cloud Tier.
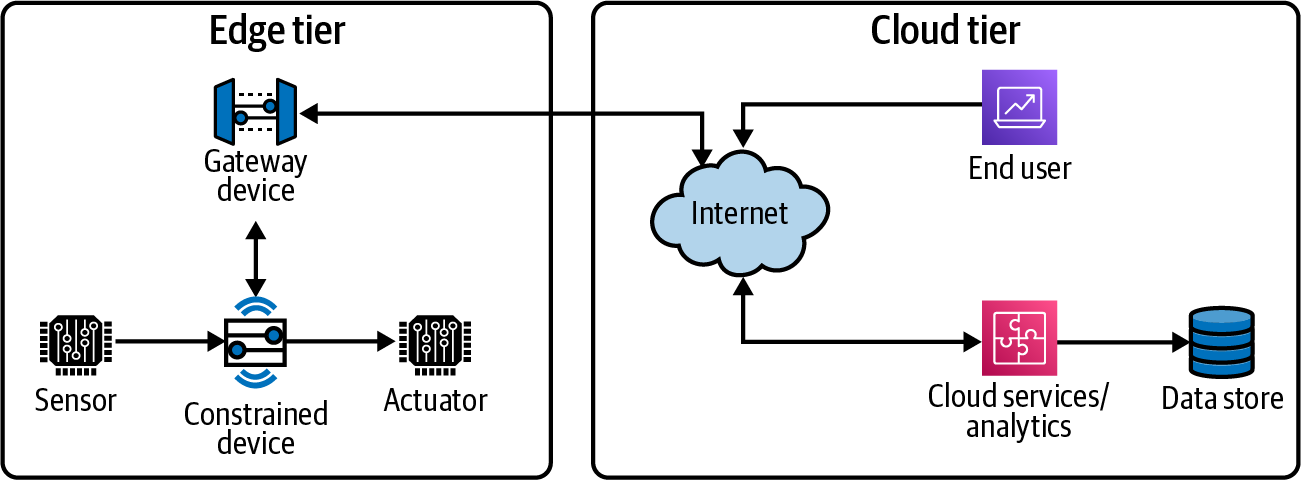
Figure P-1. Notional IoT system architecture
For the purposes of this book, I’ll qualify these devices as follows:
-
Gateway devices focus primarily on processing, interpreting, and integrating edge data and interacting with the cloud. They perform “at the edge” analytics, convert and/or transform protocol stacks, determine how messages should be routed (if at all), and, of course, connect directly to the internet and the various cloud services that make the IoT useful for business stakeholders.
-
Constrained devices handle only sensing and/or actuation and control while processing messages for themselves, passing messages along if the right communications protocol is implemented, and sending messages to a gateway device. In short, their abilities are limited, and they may not be able to connect directly to the internet, relying instead on a gateway device for cloud connectivity.
Can a constrained device connect directly to the internet? Sure, if it contains a TCP/IP stack, has a routable IP address that’s accessible to and from the public internet, and has the appropriate communications hardware to talk to the internet.
For the purposes of this book, however, I’ll narrow the category of constrained devices to these two limitations:
-
They do not support packet routing directly to or from the public internet (although let’s assume they support both TCP/IP and UDP/IP) and must interact with a gateway device to be part of any IoT ecosystem.
-
They do not contain adequate computing resources to intelligently determine complex courses of action based on the data they collect.
I’ll focus on the “constrained device to gateway device to cloud connection” paradigm throughout the book, although there are other viable edge computing models that may be more suitable for your specific use case.
Conclusion
What it all means is this: as we make better computing devices that are smaller, faster, and cheaper, use them to interact with the physical world, and connect them (or their data) to the internet for processing using cloud services, we can derive insights that help deliver better business outcomes.
Thanks for reading!
Conventions Used in This Book
The following typographical conventions are used in this book:
- Italic
-
Indicates new terms, URLs, email addresses, filenames, and file extensions.
Constant width
-
Used for program listings, as well as within paragraphs to refer to program elements such as variable or function names, databases, data types, environment variables, statements, and keywords.
Constant width bold
-
Shows commands or other text that should be typed literally by the user.
Constant width italic
-
Shows text that should be replaced with user-supplied values or by values determined by context.
Tip
This element signifies a tip or suggestion.
Note
This element signifies a general note.
Warning
This element indicates a warning or caution.
Using Code Examples
Supplemental material (code examples and documentation templates) can be found online at https://github.com/programming-the-iot. The code samples in this book are licensed under the MIT License.
If you have a technical question or a problem using the code examples, please send email to bookquestions@oreilly.com.
This book is here to help you get your job done. In general, if example code is offered with this book, you may use it in your programs and documentation. You do not need to contact us for permission unless you’re reproducing a significant portion of the code. For example, writing a program that uses several chunks of code from this book does not require permission. Selling or distributing examples from O’Reilly books does require permission. Answering a question by citing this book and quoting example code does not require permission. Incorporating a significant amount of example code from this book into your product’s documentation does require permission.
We appreciate, but generally do not require, attribution. An attribution usually includes the title, author, publisher, and ISBN. For example: “Programming the Internet of Things by Andy King (O’Reilly). Copyright 2021 Andrew D. King, 978-1-492-08141-8.”
If you feel your use of code examples falls outside fair use or the permission given above, feel free to contact us at permissions@oreilly.com.
O’Reilly Online Learning
Note
For more than 40 years, O’Reilly Media has provided technology and business training, knowledge, and insight to help companies succeed.
Our unique network of experts and innovators share their knowledge and expertise through books, articles, and our online learning platform. O’Reilly’s online learning platform gives you on-demand access to live training courses, in-depth learning paths, interactive coding environments, and a vast collection of text and video from O’Reilly and 200+ other publishers. For more information, visit http://oreilly.com.
How to Contact Us
Please address comments and questions concerning this book to the publisher:
- O’Reilly Media, Inc.
- 1005 Gravenstein Highway North
- Sebastopol, CA 95472
- 800-889-8969 (in the United States or Canada)
- 707-829-7019 (international or local)
- 707-829-0104 (fax)
- support@oreilly.com
- https://www.oreilly.com/about/contact.html
We have a web page for this book, where we list errata, examples, and any additional information. You can access this page at https://oreil.ly/programming-the-IoT.
For news and information about our books and courses, visit http://oreilly.com.
Find us on Facebook: http://facebook.com/oreilly
Follow us on Twitter: http://twitter.com/oreillymedia
Watch us on YouTube: http://youtube.com/oreillymedia
Acknowledgments
I think we’re all on a perpetual path of finding ourselves, whether through career, connection, or missional work. The specific twists and turns God has led me through, and the mentors and companions He’s put in my life, represent the window through which I’ve experienced His love for me. It goes without saying that this book would never have happened if it weren’t for the patience, critiques, encouragement, and expertise of family, friends, and colleagues who were willing to join me on this journey. I’ll attempt to acknowledge everyone who’s influenced me and impacted this writing, but I’m afraid I’ll inadvertently miss some callouts. Please forgive any unintended oversights.
I started programming at the age of 12 or so, after my dad brought home an Atari 400 that a friend had lent him. After digging into BASIC for a bit (and losing all my work after each power cycle), my parents bought me my own Atari 800XL with tape storage (remember that?), which we subsequently upgraded to 5¼” floppy disk storage and eventually enhanced with a blazingly fast 1200 Kbps modem. Thanks, Mom and Dad. Dad...wish you were still here with us.
Fast-forward 25 years, and I (eventually) became a programmer and computer scientist. But how did this project start? My friend Jimmy Song, author of Programming Bitcoin (O’Reilly), connected me to the O’Reilly Media team after I reached out one day and said, “I’m thinking of writing a book...” I truly appreciate Jimmy’s candid advice, feedback, and encouragement throughout this journey.
Of course, the genesis of this book’s lab module content is my Connected Devices course at Northeastern University. As such, I am very fortunate to work with some amazing colleagues in academia, such as Dr. Peter O’Reilly, Director of the Cyber Physical Systems program at Northeastern University’s College of Engineering, who brought me onboard to teach Connected Devices. Dr. Rolando Herrero, also from Northeastern, was one of the earliest reviewers of the book’s content. His expert knowledge of IoT systems and protocols was immensely helpful in shaping the form and function of the material. Working with both Peter and Rolando as friends and colleagues on building an IoT discipline has been one of the highlights of my career, and I’m deeply thankful to both for their mentorship.
To all the Connected Devices graduate students I’ve had the privilege to teach and collaborate with, a huge thank you! The content in this book and the exercises in each chapter have been inspired by your feedback, suggestions, requests, commentary, and tolerance of my silly dad jokes. You are the primary reason this book came to fruition and are why I get to live my passion for teaching (and not as a stand-up comedian).
Many of the teaching assistants for the course provided book commentary and review and helped me address disconnects between the prose and online exercises. A heartfelt thank you to these amazing colleagues: Cheng Shu, Ganeshram Kanakasabai, Harsha Vardhanram Kalyanaraman, Jaydeep Shah, Yogesh Suresh, and Yuxiang Cao. I am glad to have worked with each of them and deeply appreciate their contributions to the course’s success.
Some of my industry friends and colleagues provided detailed feedback and commentary on the pre-final draft. Tim Strunck, an expert in commercial IoT solutions engineering, provided a rich set of feedback on overall flow and technical content—I’m grateful for his friendship and keen insights, along with the amazing Foreword to this book. Steve Resnick, Managing Director at a large consulting firm and author of two books of his own, shared his executive perspective and advice on flow, form, and content, helping me to provide further clarity on key concepts. Ben Pu provided a fresh set of eyes on the overall book, which helped me view it through a lens that enabled me to connect with future readers. I’m thankful to have a network that includes professionals like Tim, Steve, and Ben, and I’m very appreciative of their quick-turn feedback and inputs.
To all my colleagues and friends at O’Reilly Media—wow, what a team! Mike Loukides, VP and Intake Editor, who spent hours on video conferences, emails, and phone calls and helped shape the proposal and get it through the intake process. Melissa Duffield, VP of Content Strategy, who worked with me on the marketing angle and pushed it through the green-lighting process in record time. Cassandra Furtado, who patiently responded to all my initial questions. Chris Faucher, who transformed my manuscript into a book. Virginia Wilson, who helped manage the schedule and tech review process. Arthur Johnson, who, as copyeditor, caught and corrected many prose and formatting consistency foibles. And of course, Sarah Grey, who, as Development Editor, helped me think through the organization of the material and make it real. I have tremendous respect for each of them, and for their professionalism, support, responsiveness, and comradery throughout this project.
A great deal of open source software and open specifications were used in the preparation of this book, its examples, and exercises. I’d like to thank the developers of and contributors to each of these projects.
Most of all, thanks to my wonderful wife, Yoon-Hi, and incredible son, Eliot: you two are the rocks in my life, and I don’t know how I would’ve even started down this path without your encouragement and support. Thank you for all the reviews, wordsmithing conversations (and edits), challenges, support, and belief! You pulled me out of valleys and pushed me up hills. You’re both amazing, and I love you more than I can possibly express.
1 All the exercises in this book are adapted from my Programming the IoT Kanban board and are used with my permission.
2 The Appendix provides a basic UML representation for each exercise. The latest UML specification can be found on the Object Management Group (OMG) website.
3 Encyclopaedia Britannica Online, s.v. “Moore’s law”, by the editors of Encyclopaedia Britannica, last updated December 26, 2019.
4 Encyclopaedia Britannica Online, s.v. “ARPANET”, by Kevin Featherly, last updated March 23, 2021.
5 Simon Duque Antón et al., “Two Decades of SCADA Exploitation: A Brief History”, in 2017 IEEE Conference on Application, Information and Network Security (AINS) (New York: IEEE, 2017), 98–104.
6 John Postel, “User Datagram Protocol”, Internet Standard RFC 768, August 28, 1980.
7 For further reading on Tim Berners-Lee’s WWW proposal, please see https://www.w3.org/History/1989/proposal.html.
8 See an explanation at “The Internet Toaster”.
9 Encyclopaedia Britannica Online, “Know Your Joe: 5 Things You Didn’t Know About Coffee” (2. The Watched Pot), by Alison Eldridge, accessed January 18, 2021.
10 Kevin Ashton, “That ‘Internet of Things’ Thing”, RFID Journal, June 22, 2009.
11 You can read a brief summary of the Arduino’s birth in the Computer History Museum’s Timeline of Computer History.
12 The Industrial Internet of Things, Volume G1: Reference Architecture, Version 1.9 (Needham, MA: Industrial Internet Consortium, 2019).
13 Carsten Bormann, Mehmet Ersue, and Ari Keränen, “Terminology for Constrained-Node Networks”, IETF Informational RFC 7228, May 2014, 8–10.
Get Programming the Internet of Things now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.