Preface
Like any of the most-used programming languages, Java has its share of detractors, advocates, issues, quirks,1 and a learning curve. The Java Cookbook aims to help the Java developer get up to speed on some of the most important parts of Java development. I focus on the standard APIs and some third-party APIs, but I don’t hesitate to cover language issues as well.
This is the fourth edition of this book, and it has been shaped by many people and by the myriad changes that Java has undergone over its first two decades of popularity. Readers interested in Java’s history can refer to Appendix A.
Java 11 is the current long-term supported version, but Java 12 and 13 are out. Java 14 is in early access and scheduled for final release the very same day as this book’s fourth edition. The new cadence of releases every six months may be great for the Java SE development team at Oracle and for click-driven, Java-related news sites, but it “may cause some extra work” for Java book authors, since books typically have a longer revision cycle than Java now does! Java 9, which came out after the previous edition of this book, was a breaking release, the first release in a very long time to break backwards compatibility, primarily the Java module system. Everything in the book is assumed to work on any JVM that is still being used to develop code. Nobody should be using Java 7 (or anything before it!) for anything, and nobody should be doing new development in Java 8. If you are, it’s time to move on!
The goal of this revision is to keep the book up to date with all this change. While cutting out a lot of older material, I’ve added information on new features such as Modules and the interactive JShell, and I’ve updated a lot of other information along the way.
Who This Book Is For
I’m going to assume that you know the basics of Java. I won’t tell
you how to println
a string, nor how
to write a class that extends another and/or implements an interface.
I presume you’ve taken a Java course such as
Learning Tree’s Introduction or that you’ve studied an
introductory book such as Head First Java, Learning Java,
or Java in a Nutshell (O’Reilly). However, Chapter 1 covers some techniques that you
might not know very well and that are necessary to understand some
of the later material. Feel free to skip around! Both the printed
version of the book and the electronic copy are heavily cross-referenced.
What’s in This Book?
Java has seemed better suited to “development in the large,” or enterprise application development, than to the one-line, one-off script in Perl, Awk, or Python. That’s because it is a compiled, object-oriented language. However, this suitability has changed somewhat with the appearance of JShell (see Recipe 1.4). I illustrate many techniques with shorter Java class examples and even code fragments; some of the simpler ones will be shown using JShell. All of the code examples (other than some one- or two-liners) are in one of my public GitHub repositories, so you can rest assured that every fragment of code you see here has been compiled, and most have been run recently.
Some of the longer examples in this book are tools that I originally wrote
to automate some mundane task or another. For example, a tool called
MkIndex
(in the javasrc repository) reads the top-level directory of the
place where I keep my Java example source code, and it builds a
browser-friendly index.html file for that directory. Another example is XmlForm
, which was used to convert parts of the manuscript from XML into the form needed by another publishing software. XmlForm
also handled—by use of another program,
GetMark
—full and partial code insertions from the javasrc directory
into the book manuscript. XmlForm
is included in the Github repository
I mentioned, as is a later version of GetMark
, though neither of these
was used in building the fourth edition. These days, O’Reilly’s Atlas publishing
software uses
Asciidoctor, which provides the mechanism we use for inserting files and parts of files into the book.
Organization of This Book
Let’s go over the organization of this book. Each chapter consists of a handful of recipes, short sections that describe a problem and its solution, along with a code example. The code in each recipe is intended to be largely self-contained; feel free to borrow bits and pieces of any of it for use in your own projects. The code is distributed with a Berkeley-style copyright, just to discourage wholesale reproduction.
I start off Chapter 1, “Getting Started: Compiling and Running Java”, by describing some methods of compiling your program on different platforms, running them in different environments (browser, command line, windowed desktop), and debugging.
Chapter 2, “Interacting with the Environment”, moves from compiling and running your program to getting it to adapt to the surrounding countryside—the other programs that live in your computer.
The next few chapters deal with basic APIs. Chapter 3, “Strings and Things”, concentrates on one of the most basic but powerful data types in Java, showing you how to assemble, dissect, compare, and rearrange what you might otherwise think of as ordinary text. This chapter also covers the topic of internationalization/localization so that your programs can work as well in Akbar, Afghanistan, Algiers, Amsterdam, and Angleterre as they do in Alberta, Arkansas, and Alabama.
Chapter 4, “Pattern Matching with Regular Expressions”, teaches you how to use the powerful regular expressions technology from Unix in many string-matching and pattern-matching problem domains. Regex processing has been standard in Java for years, but if you don’t know how to use it, you may be reinventing the flat tire.
Chapter 5, “Numbers”, deals both with built-in numeric types such as int
and double
, as well as the corresponding API classes (Integer
, Double
, etc.) and the conversion and testing facilities they offer. There is also brief mention of the “big number” classes. Because Java programmers often need to deal in dates and times, both locally and internationally, Chapter 6, “Dates and Times”, covers this important topic.
The next few chapters cover data processing. As in most languages, arrays in Java are linear, indexed collections of similar objects, as discussed in Chapter 7, “Structuring Data with Java”. This chapter goes on to deal with the many Collections classes: powerful ways of storing quantities of objects in the java.util
package, including use of Java Generics.
Despite some syntactic resemblance to procedural languages such as C, Java
is at heart an Object-Oriented Programming (OOP) language, with some
important Functional Programming (FP) constructs skilfully blended in.
Chapter 8, “Object-Oriented Techniques”, discusses some of the key
notions of OOP as it applies to Java, including the commonly overridden
methods of java.lang.Object
and the important issue of design patterns.
Java is not, and never will be, a pure FP language.
However, it is possible to use some aspects of FP, increasingly so with Java
8 and its support of lambda expressions (a.k.a. closures). This is
discussed in Chapter 9, “Functional Programming Techniques: Functional Interfaces, Streams, and Parallel Collections”.
The next chapter deals with aspects of traditional input and output. Chapter 10, “Input and Output: Reading, Writing, and Directory Tricks”, details the rules for reading and writing files (don’t skip this if you think files are boring; you’ll need some of this information in later chapters). The chapter also shows you everything else about files—such as finding their size and last-modified time—and about reading and modifying directories, creating temporary files, and renaming files on disk.
Big data and data science have become a thing, and Java is right in there. Apache Hadoop, Apache Spark, and much more of the big data infrastructure is written in, and extensible with, Java, as described in Chapter 11, “Data Science and R”. The R programming language is popular with data scientists, statsticians, and other scientists. There are at least two reimplementations of R coded in Java, and Java can also be interfaced directly with the standard R implementation in both directions, so this chapter covers R as well.
Because Java was originally promulgated as the programming language for the internet, it’s only fair to spend some time on networking in Java. Chapter 12, “Network Clients”, covers the basics of network programming from the client side, focusing on sockets. Today so many applications need to access a web service, primarily RESTful web services, that this seemed to be necessary. I’ll then move to the server side in Chapter 13, “Server-Side Java”, wherein you’ll learn some server-side programming techniques.
One simple text-based representation for data interchange is JSON, the JavaScript object notation. Chapter 14, “Processing JSON Data”, describes the format and some of the many APIs that have emerged to deal with it.
Chapter 15, “Packages and Packaging”, shows how to create packages of classes that work together. This chapter also talks about deploying (a.k.a. distributing and installing) your software.
Chapter 16, “Threaded Java”, tells you how to write classes that appear to do more than one thing at a time and let you take advantage of powerful multiprocessor hardware.
Chapter 17, “Reflection, or “A Class Named Class””, lets you in on such secrets as how to write API cross-reference documents mechanically and how web servers are able to load any old Servlet—never having seen that particular class before—and run it.
Sometimes you already have code written and working in another language that can do part of your work for you, or you want to use Java as part of a larger package. Chapter 18, “Using Java with Other Languages”, shows you how to run an external program (compiled or script) and also interact directly with native code in C/C++ or other languages.
There isn’t room in a book this size for everything I’d like to tell you about Java. The Afterword presents some closing thoughts and a link to my online summary of Java APIs that every Java developer should know about.
Finally, Appendix A, “Java Then and Now”, gives the storied history of Java in a release-by-release timeline, so whatever version of Java you learned, you can jump in here and get up to date quickly.
So many topics, and so few pages! Many topics do not recieve 100% coverage; I’ve tried to include the most important or most useful parts of each API. To go beyond, check the official javadoc pages for each package; many of these pages have some brief tutorial information on how the package is to be used.
Besides the parts of Java covered in this book, two other platform editions, Java ME and Java EE, have been standardized. Java Micro Edition (Java ME) is concerned with small devices such as handhelds, cell phones, and fax machines. At the other end of the size scale—large server machines—there’s Eclipse Jakarta EE, replacing the former Java EE, which in the last century was known as J2EE. Jakarta EE is concerned with building large, scalable, distributed applications. APIs that are part of Jakarta EE include Servlets, JavaServer Pages, JavaServer Faces, JavaMail, Enterprise JavaBeans (EJBs), Container and Dependency Injection (CDI), and Transactions. Jakarta EE packages normally begin with “javax” because they are not core packages. This book mentions but a few of these; there is also a Java EE 8 Cookbook by Elder Moraes (O’Reilly) that covers some of the Jakarta EE APIs, as well as an older Java Servlet & JSP Cookbook by Bruce Perry (O’Reilly).
This book doesn’t cover Java Micro Edition, Java ME. At all. But speaking of cell phones and mobile devices, you probably know that Android uses Java as its language. What should be comforting to Java developers is that Android also uses most of the core Java API, except for Swing and AWT, for which it provides Android-specific replacements. The Java developer who wants to learn Android may consider looking at my Android Cookbook (O’Reilly), or the book’s website.
Java Books
A lot of useful information is packed into this book. However, due to the breadth of topics, it is not possible to give book-length treatment to any one topic. Because of this, the book contains references to many websites and other books. In pointing out these references, I’m hoping to serve my target audience: the person who wants to learn more about Java.
O’Reilly publishes, in my opinion, the best selection of Java books on the market. As the API continues to expand, so does the coverage. Check out the complete list of O’Reilly’s collection of Java books; you can buy them at most bookstores, both physical and virtual. You can also read them online through the O’Reilly Online Learning Platform, a paid subscription service. And, of course, most are now available in ebook format; O’Reilly ebooks are DRM free, so you don’t have to worry about their copy-protection scheme locking you into a particular device or system, as you do with certain other publishers.
Though many books are mentioned at appropriate spots in the book, a few deserve special mention here.
First and foremost, David Flanagan and Benjamin Evan’s Java in a Nutshell (O’Reilly) offers a brief overview of the language and API and a detailed reference to the most essential packages. This is handy to keep beside your computer. Head First Java by Bert Bates and Kathy Sierra offers a much more whimsical introduction to the language and is recommended for the less experienced developer.
Java 8 Lambdas (Warburton, O’Reilly) covers the Lambda syntax introduced in Java 8 in support of functional programming and more concise code in general.
Java 9 Modularity: Patterns and Practices for Developing Maintainable Applications by Sander Mak and Paul Bakker (O’Reilly) covers the important changes made in the language in Java 9 for the Java module system.
Java Virtual Machine by Jon Meyer and Troy Downing (O’Reilly) will intrigue the person who wants to know more about what’s under the hood. This book is out of print but can be found used and in libraries.
A definitive (and monumental) description of programming the Swing GUI is Java Swing by Robert Eckstein et al. (O’Reilly).
Java Network Programming and Java I/O, both by Elliotte Harold (O’Reilly), are also useful references.
For Java Database work, Database Programming with JDBC & Java by George Reese (O’Reilly) and Pro JPA 2: Mastering the Java Persistence API by Mike Keith and Merrick Schincariol (Apress) are recommended. Or my forthcoming overview of Java Database.
Although the book you’re now reading doesn’t have much coverage of the Java EE, I’d like to mention two books on that topic:
-
Arun Gupta covers the Enterprise Edition in Java EE 7 Essentials (O’Reilly).
-
Adam Bien’s Real World Java EE Patterns: Rethinking Best Practices offers useful insights in designing and implementing an Enterprise application.
You can find more at the O’Reilly website.
Finally, although it’s not a book, Oracle has a great deal of Java information on the web. This web page used to feature a large diagram showing all the components of Java in a “conceptual diagram.” An early version of this is shown in Figure P-1; each colored box is a clickable link to details on that particular technology.
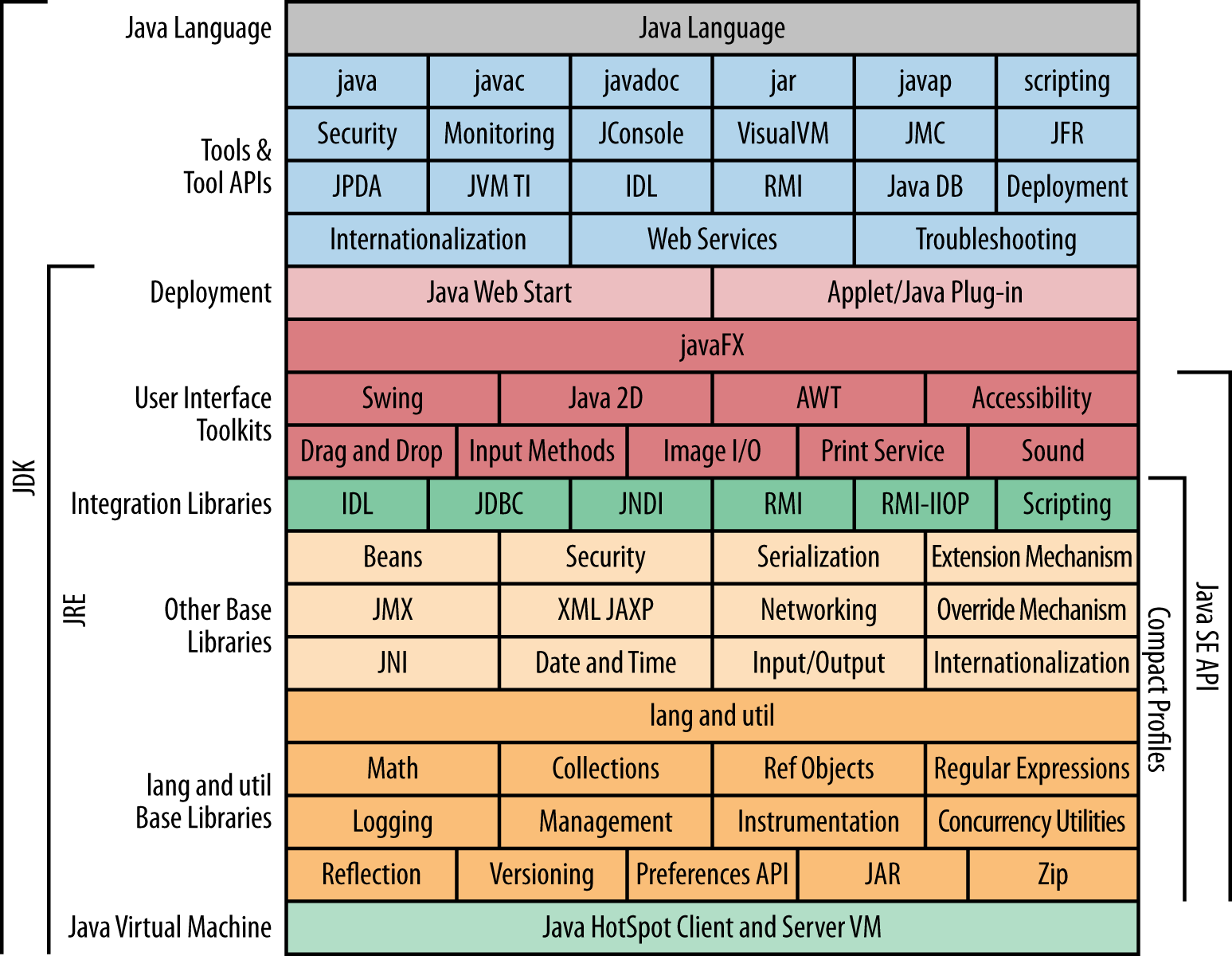
Figure P-1. Java conceptual diagram from Oracle documentation
For better or for worse, newer versions of Java have replaced this with a text page; for Java 13 the page is at https://docs.oracle.com/en/java/javase/13.
General Programming Books
Donald E. Knuth’s The Art of Computer Programming (Addison-Wesley) has been a source of inspiration to generations of computing students since its first publication in 1968. Volume 1 covers Fundamental Algorithms, Volume 2 is Seminumerical Algorithms, Volume 3 is Sorting and Searching, and Volume 4A is Combinatorial Algorithms, Part 1. The remaining volumes in the projected series are not completed. Although his examples are far from Java (he invented the hypothetical assembly language MIX for his examples), many of his discussions of algorithms—of how computers ought to be used to solve real problems—are as relevant today as they were years ago.2
Though its code examples are quite dated now, the book The Elements of Programming Style by Brian Kernighan and P. J. Plauger (McGraw-Hill) set the style (literally) for a generation of programmers with examples from various structured programming languages. Kernighan and Plauger also wrote a pair of books, Software Tools (Addison-Wesley) and Software Tools in Pascal (Addison-Wesley), which demonstrated so much good advice on programming that I used to advise all programmers to read them. However, these three books are dated now; many times I wanted to write a follow-on book in a more modern language. Instead I now defer to The Practice of Programming, Kernighan’s follow-on—cowritten with Rob Pike (Addison-Wesley)—to the Software Tools series. This book continues the Bell Labs tradition of excellence in software textbooks. In previous editions of this book, I had even adapted one bit of code from their book, their CSV parser. Finally, Kernighan recently published UNIX: A History and a Memoir, his take on the story of Unix.
See also The Pragmatic Programmer by Andrew Hunt and David Thomas (Addison-Wesley).
Design Books
Peter Coad’s Java Design (PTR-PH/Yourdon Press) discusses the issues of object-oriented analysis and design specifically for Java. Coad is somewhat critical of Java’s implementation of the observable-observer paradigm and offers his own replacement for it.
One of the most famous books on object-oriented design in recent years is Design Patterns by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides (Addison-Wesley). These authors are often collectively called the “Gang of Four,” resulting in their book sometimes being referred to as the GoF book. One of my colleagues called it “the best book on object-oriented design ever,” and I agree; at the very least, it’s among the best.
Refactoring by Martin Fowler (Addison-Wesley) covers a lot of “coding cleanups” that can be applied to code to improve readability and maintainability. Just as the GoF book introduced new terminology that helps developers and others communicate about how code is to be designed, Fowler’s book provided a vocabulary for discussing how it is to be improved. But this book may be less useful than others; many of the refactorings now appear in the Refactoring Menu of the Eclipse IDE (see Recipe 1.3).
Two important streams of methodology theories are currently in circulation. The first is collectively known as Agile methods, and its best-known members are Scrum and Extreme Programming (XP). XP (the methodology, not that really old flavor of Microsoft’s OS) is presented in a series of small, short, readable texts led by its designer, Kent Beck. The first book in the XP series is Extreme Programming Explained (Addison-Wesley). A good overview of all the Agile methods is Jim Highsmith’s Agile Software Development Ecosystems (Addison-Wesley).
Another group of important books on methodology, covering the more traditional object-oriented design, is the UML series led by “the Three Amigos” (Booch, Jacobson, and Rumbaugh). Their major works are the UML User Guide, UML Process, and others. A smaller and more approachable book in the same series is Martin Fowler’s UML Distilled.
Conventions Used in This Book
This book uses the following conventions.
Programming Conventions
I use the following terminology in this book. A program means any unit of code that can be run: from a five-line main program, to a servlet or web tier component, an EJB, or a full-blown GUI application. Applets were Java programs for use in a web browser; these were popular for a while but barely exist today. A servlet is a Java component built using Jakarta EE APIs for use in a web server, normally via HTTP. EJBs are business-tier components built using Jakarta APIs. An application is any other type of program. A desktop application (a.k.a. client) interacts with the user. A server program deals with a client indirectly, usually via a network connection (usually HTTP/HTTPS these days).
The examples shown are in two varieties. Those that begin with zero or more import statements, a javadoc comment, and a public class
statement are complete examples. Those that begin with a declaration or executable statement, of course, are excerpts. However, the full versions of these excerpts have been compiled and run, and the online source includes the full versions.
Recipes are numbered by chapter and number, so, for example, Recipe 8.1 refers to the first recipe in Chapter 8.
Typesetting Conventions
The following typographic conventions are used in this book:
- Italic
-
Used for commands, filenames, and example URLs. It is also used for emphasis and to define new terms when they first appear in the text.
Constant width
-
Used in code examples to show partial or complete Java source code program listings. It is also used for class names, method names, variable names, and other fragments of Java code.
Constant width bold
-
Used for user input, such as commands that you type on the command line.
Constant width italic
-
Shows text that should be replaced with user-supplied values or by values determined by context.
Tip
This element signifies a tip or suggestion.
Note
This element signifies a general note.
Warning
This icon indicates a warning or caution.
Code Examples
The code samples for this book are on the author’s GitHub. Most are in the repository javasrc, but a few are pulled in from one other repository, darwinsys-api. Details on downloading these are in Recipe 1.6.
Many programs are accompanied by an example showing them in action, run from the command
line. These will usually show a prompt ending in either $
for Unix or >
for
Windows, depending on which computer I was using the day I wrote that example.
If there is text before this prompt character, it can be ignored. It may be a pathname or a hostname, again, depending on the system.
These examples will usually also show the full package name of the class because Java requires this when starting a program from the command line. And because that will remind you which subdirectory of the source repository to find the source code in, I won’t be pointing it out explicitly very often.
We appreciate, but generally do not require, attribution. An attribution usually includes the title, author, publisher, and ISBN. For example: “Java Cookbook by Ian F. Darwin (O’Reilly). Copyright 2020 RejmiNet Group, Inc., 978-1-492-07258-4.”
If you feel your use of code examples falls outside fair use or the permission given above, feel free to contact us at permissions@oreilly.com.
O’Reilly Online Learning
Note
For more than 40 years, O’Reilly Media has provided technology and business training, knowledge, and insight to help companies succeed.
Our unique network of experts and innovators share their knowledge and expertise through books, articles, conferences, and our online learning platform. O’Reilly’s online learning platform gives you on-demand access to live training courses, in-depth learning paths, interactive coding environments, and a vast collection of text and video from O’Reilly and 200+ other publishers. For more information, please visit http://oreilly.com.
Comments and Questions
As mentioned earlier, I’ve tested all the code on at least one of the reference platforms, and most on several. Still, there may be platform dependencies, or even bugs, in my code or in some important Java implementation. Please report any errors you find, as well as your suggestions for future editions, by writing to:
- O’Reilly Media, Inc.
- 1005 Gravenstein Highway North
- Sebastopol, CA 95472
- 800-998-9938 (in the United States or Canada)
- 707-827-0515 (international or local)
- 707-829-0104 (fax)
There is a web page for this book where we list errata, examples, and any additional information. It can be accessed at http://shop.oreilly.com/product/0636920304371.do.
Email bookquestions@oreilly.com to comment or ask technical questions about this book.
For more information about our books, courses, conferences, and news, see our website at http://www.oreilly.com.
Find us on LinkedIn: https://linkedin.com/company/oreilly-media
Watch us on YouTube: http://www.youtube.com/oreillymedia
The O’Reilly site lists errata. You’ll also find the source code for all the Java code examples to download; please don’t waste your time typing them again! For specific instructions, see Recipe 1.6.
Acknowledgments
I wrote in the Afterword to the first edition that “writing this book has been a humbling experience.” I should add that maintaining it has been humbling, too. While many have been lavish with their praise—one very kind reviewer called it “arguably the best book ever written on the Java programming language”—I have been humbled by the number of errors and omissions in earlier editions. I have endeavored to correct these.
My life has been touched many times by the flow of the fates bringing me into contact with the right person to show me the right thing at the right time. Steve Munro, with whom I’ve long since lost touch, introduced me to computers when we were in the same class in high school—in particular an IBM 360/30 at the Toronto Board of Education that was bigger than a living room, had 32 or 64K (not M or G!) of memory, and had perhaps the power of a PC/XT. The late Herb Kugel took me under his wing at the University of Toronto while I was learning about the larger IBM mainframes that came later. Terry Wood and Dennis Smith at the University of Toronto introduced me to mini- and micro-computers before there was an IBM PC. On evenings and weekends, the Toronto Business Club of Toastmasters International and Al Lambert’s Canada SCUBA School allowed me to develop my public speaking and teaching abilities. Several people at the University of Toronto, but especially Geoffrey Collyer, taught me the features and benefits of the Unix operating system at a time when I was ready to learn it.
Thanks to the many Learning Tree instructors and students who showed me ways of improving my presentations. I still teach for “The Tree” and recommend their courses for the busy developer who wants to zero in on one topic in detail over four days.
Closer to this project, Tim O’Reilly believed in my “little Lint book” when it was just a sample chapter from a proposed longer work, enabling my early entry into the rarefied circle of O’Reilly authors. Years later, Mike Loukides encouraged me to keep trying to find a Java book idea that both he and I could work with. And he stuck by me when I kept falling behind the deadlines. Mike also read the entire manuscript and made many sensible comments, some of which brought flights of fancy down to earth. Jessamyn Read turned many faxed and emailed scratchings of dubious legibility into the quality illustrations you see in this book. And many, many other talented people at O’Reilly helped put this book into the form in which you now see it.
The code examples are now dynamically included (so updates get done faster) rather than pasted in. My son (and functional programming developer) Benjamin Darwin helped meet the deadline by converting almost the entire code base to O’Reilly’s newest “include” mechanism and by resolving a couple of other non-Java presentation issues. He also helped make Chapter 9 clearer and more functional.
At O’Reilly
For this fourth edition of the book, Suzanne McQuade was the editorial overseer, and Corbin Collins the principal editor. Corbin was especially meticulous in checking the manuscript. Meghan Blanchette, Sarah Schneider, Adam Witwer, Melanie Yarbrough, and the many production people listed on the Copyright page all played a part in getting the third edition ready for you to read. Thanks to Mike Loukides, Deb Cameron, and Marlowe Shaeffer for editorial and production work on the second edition.
Technical Reviewers
For the fourth edition I was blessed to have two very thorough technical reviewers, Sander Mak and Daniel Hinojosa. Many issues that I hadn’t considered during the main revision were called out by these two, leading to extensive rewrites and changes in the last few weeks before the O’Reilly production team took over. Thanks so much to both of you!
My reviewer for the third edition, Alex Stangl, read the third edition manuscript and went far above the call of duty, making innumerable helpful suggestions, even finding typos that had been present in previous editions! Helpful suggestions on particular sections were made by Benjamin Darwin, Mark Finkov, and Igor Savin. For anyone I’ve forgotten to mention, I thank you all!
Bil Lewis and Mike Slinn made helpful comments on multiple drafts of the first edition. Ron Hitchens and Marc Loy carefully read the entire final draft of the first edition. I am grateful to Mike Loukides for his encouragement and support throughout the process. Editor Sue Miller helped shepherd the manuscript through the somewhat energetic final phases of production. Sarah Slocombe read the XML chapter in its entirety and made many lucid suggestions; unfortunately, time did not permit me to include all of them in the first edition.
Jonathan Knudsen, Andy Oram, and David Flanagan commented on book’s outline when it was little more than a list of chapters and recipes, and they were able to see the kind of book it could become and suggest ways to make it better.
Each of these people made this book better in many ways, particularly by suggesting additional recipes or revising existing ones. Thanks to one and all! The faults that remain are my own.
Readers
My sincere thanks to all the readers who found errata and suggested improvements. Every new edition is better for the efforts of folks like you who take the time and trouble to report that which needs reporting!
Special mention must be made of one of the book’s German translators,3 Gisbert Selke, who read the first edition cover to cover during its translation and clarified my English. Gisbert did it all over again for the second edition and provided many code refactorings, which have made this a far better book than it would be otherwise. Going beyond the call of duty, Gisbert even contributed one recipe (Recipe 18.5) and revised some of the other recipes in the same chapter. Thank you, Gisbert!
The second edition also benefited from comments by Jim Burgess, who read large parts of the book. Comments on individual chapters were received from Jonathan Fuerth, the late Kim Fowler, Marc Loy, and Mike McCloskey. My wife, Betty, and my then-teenaged children each proofread several chapters as well.
The following people contributed significant bug reports or suggested improvements: Rex Bosma, Rod Buchanan, John Chamberlain, Keith Goldman, Gilles-Philippe Gregoire, B. S. Hughes, Jeff Johnston, Rob Konigsberg, Tom Murtagh, Jonathan O’Connor, Mark Petrovic, Steve Reisman, Bruce X. Smith, and Patrick Wohlwend.
Etc.
My dear wife, Betty Cerar, still knows more about the caffeinated beverage that I drink while programming than the programming language I use, but her passion for clear expression and correct grammar has benefited so much of my writing during our life together.
No book on Java would be complete without a note of thanks to James Gosling for inventing Java (he also invented the first Unix Emacs, the sc spreadsheet, and the NeWS window system). Thanks also to his employer Sun Microsystems (before they were taken over by Oracle) for releasing not only the Java language but an incredible array of Java tools and API libraries freely available over the internet.
Willi Powell of Apple Canada provided macOS access for the first edition, back in the early days of macOS.
To each and every one of you, my sincere thanks.
Book Production Software
I used a variety of tools and operating systems in preparing, compiling, and testing this book. The developers of OpenBSD, “the proactively secure Unix-like system,” deserve thanks for making a stable and secure Unix clone that is also closer to traditional Unix than other freeware systems. I used the vi editor (vi on OpenBSD and vim on Windows) while inputting the original manuscript in XML, and I used Adobe FrameMaker (a wonderful GUI-based documentation tool that Adobe bought and subsequently destroyed) to format the documents. I do not know if I can ever forgive Adobe for destroying what was arguably the world’s best documentation system and for making the internet such a dangerous place by keeping the bug-infested Flash alive long past its best-before century. I do know I will never use a documentation system from Adobe for anything.
Because of this, the crowd-sourced Android Cookbook that I edited was not prepared with FrameMaker, but instead XML DocBook (generated from wiki markup on a Java-powered website that I wrote for the purpose) and a number of custom tools provided by O’Reilly’s tools group.
The third and fourth editions of this Java Cookbook were formatted in Asciidoctor and brought to life on the publishing toolchain of O’Reilly’s Atlas.
1 For the quirks, see the Java Puzzlers books by Joshua Bloch and Neal Gafter (Addison-Wesley).
2 With possible exceptions for algorithm decisions that are less relevant today given the massive changes in computing power now available.
3 Earlier editions are or have been available in English, German, French, Polish, Russian, Korean, Traditional Chinese, and Simplified Chinese. My thanks to all the translators for their efforts in making the book available to a wider audience.
Get Java Cookbook, 4th Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.