Chapter 1. Getting Started with .NET on AWS
This chapter covers the basic scaffolding of essentials on your first day working with AWS and C#. These essentials include using cloud-based development environments such as AWS CloudShell and a traditional Visual Studio development environment that leverages the AWS SDK.
The material in this book sets you up for success by adding many code examples of doing .NET 6 development on AWS. You can view those code examples in the source code repository for the book. Additionally, both traditional development methods like Visual Studio and new cloud native strategies like developing with AWS Cloud9 are covered. There is something for all types of .NET developers.
For scripting fans, there are also examples of using the AWS Command Line Interface and PowerShell for the AWS SDK. At the end of the chapter, there are discussion questions and exercises that you can use to build on the lessons covered. These exercises are an excellent tool for creating a comprehensive portfolio of work that gets you hired. Let’s get started with a brief dive into cloud computing.
What Is Cloud Computing?
Cloud computing is the utility-type delivery of IT resources over the internet with utility-type pricing. Instead of purchasing and maintaining physical data centers and hardware, you can access IT services, such as computing, storage, and databases, on an as-needed basis from a cloud vendor.
Perhaps the best way to describe cloud computing is to take the view of the UC Berkeley Reliable Adaptive Distributed Systems Laboratory or RAD Lab. In their paper “Above the Clouds: A Berkeley View of Cloud Computing”, they mention three new critical aspects of cloud computing: “the illusion of infinite computing resources,” “the elimination of up-front commitment by cloud users,” and “the ability to pay for the use of computing resources on a short-term basis as needed.” Let’s talk about these items in more detail.
Note
Having taught thousands of students and working professionals cloud computing, I (Noah) have strong opinions on learning as quickly as possible. A key performance indicator, or KPI, is how many mistakes you can make per week. In practice, this means trying things out, getting frustrated, then working out the best way to solve what is frustrating you, and then doing that over and over again as quickly as possible.
One of the ways I encourage this in a classroom is by facilitating students to demo progress weekly on what they are working on and build a portfolio of work. Similarly, in this book, I recommend building out a series of GitHub projects that catalog your work and then building out demo videos explaining how your project works. This step accomplishes two things in learning cloud computing: teaching yourself better metacognition skills and building a portfolio of work that makes you more marketable.
- The illusion of infinite computing resources
-
Machine learning is an excellent example of an ideal use case for “near infinite” computing resources. Deep learning requires access to large quantities of storage, disk I/O, and compute. Through elastic compute and storage, data lake capability via Amazon S3 opens up workflows that didn’t exist previously. This new workflow allows users and systems to operate data where it resides versus moving it back and forth to workstations or specialized file servers. Notice in Figure 1-1 that the Amazon S3 storage system can seamlessly handle the scaling of computing, storage, and disk I/O with any number of workers that access the resource. Amazon S3 can do this not only because it contains near infinite elasticity but also due to redundant properties. This concept means that it not only scales but can virtually always be available because of the design around the idea that “things always fail”.
Note
One definition of machine learning is a program that learns from historical data to create predictions. You can use AWS SageMaker Studio to train your own machine learning models without knowing anything about data science with a feature called Amazon SageMaker Autopilot. You can learn more about that service here.
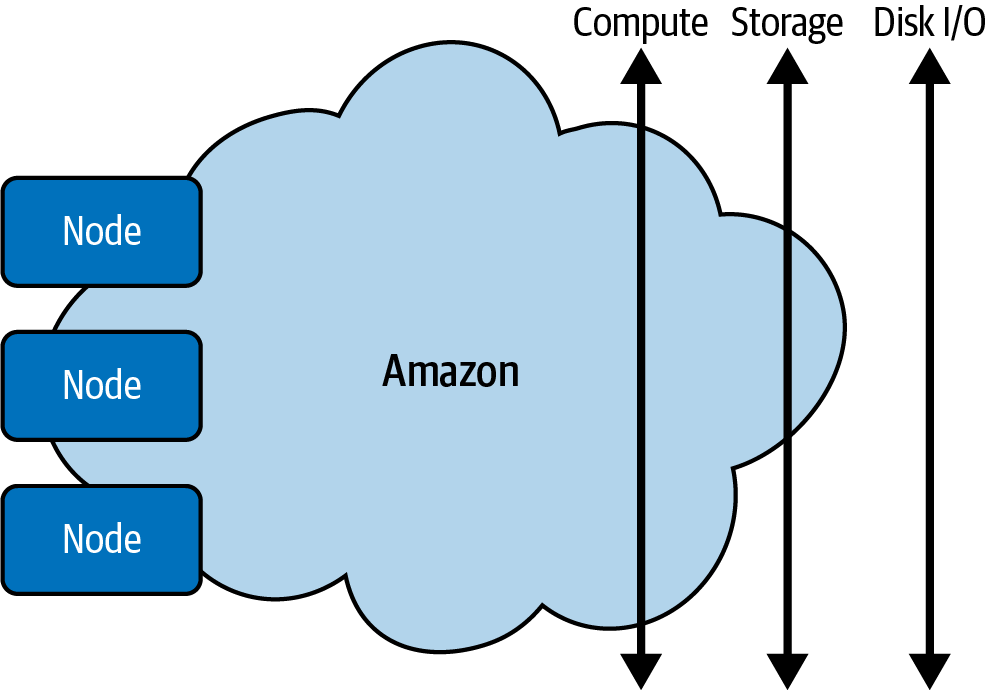
Figure 1-1. Near infinite compute
- The elimination of up-front commitment by cloud users
-
For many start-ups, spending hundreds of thousands of dollars on buying equipment to try out an idea is not feasible. The cloud enables an opportunistic way to develop software by eliminating high fixed costs before cloud computing. Not only that, but you are not locked into a particular technology choice as you are in an on-premise data center.
- The ability to pay for the use of computing resources on a short-term basis as needed
-
By switching to variable costs, companies only pay for what they need. The result is more efficient architectures that can respond to events and scale according to demand. As you can see, the critical cloud benefit is the ability to use elastic capabilities, namely, compute and storage. One such resource is an Elastic File System (EFS) on AWS. According to AWS, EFS is a “simple, serverless, set-and-forget, elastic file system.” It works well with the core elasticity capability of AWS in that it allows a developer to provision or tear down ephemeral resources like spot instances for a task yet interact with persistent storage available to all machines that use it. Spot instances are compute instances you can bid on and obtain for up to 90% cost savings, making them ideal for workflows that can be run anytime or are transient.1
Note
AWS takes cost optimization very seriously and includes it as one of their AWS Well-Architected Framework pillars. According to AWS, “The AWS Well-Architected Framework helps you understand the pros and cons of decisions you make while building systems on AWS.” Spot instances are a considerable part of the cost optimization story because they allow you to bid on unused capacity, achieving, as a result, up to 90% off regular on-demand pricing. For developers learning something, this resource is invaluable because you only “pay for what you need.”
Fully managed networked file systems like EFS are helpful in that they provide a consistent network experience, like a persistent home directory or data share. “Amazon Elastic File System (Amazon EFS) automatically grows and shrinks as you add and remove files with no need for management or provisioning,” according to official AWS documentation.
One of the most troublesome bottlenecks in dealing with large data is needing more compute, disk I/O (input/output), and storage to process a task, like counting the visits to a website for a company with terabytes of data. In particular, the ability to seamlessly use a file system mountable by a cluster of machines (i.e., EFS) and grow to meet the I/O demands of the cluster’s aggregate work is a massive efficiency that did not exist until cloud computing.
Another elastic resource is EC2 virtual computing environments, also known as instances. EC2 instances are a basic level of scalable computing capacity provided by AWS. They have many features, including using Amazon Machine Images (AMIs) that package a complete server install. They are handy for scaling web services, trying out prototypes, or bidding on spare capacity, as with AWS Spot Instances. Spot Instances open up entirely new ways of working because virtual machines can be disposable, i.e., discarded when not needed, and used when the costs are low enough to justify their use.
Types of Cloud Computing
One consideration to be aware of is the types of cloud computing: IaaS (Infrastructure as a Service), PaaS (Platform as a Service), FaaS (Function as a Service), and SaaS (Software as a Service). Let’s discuss each in detail.
- IaaS
-
This option offers the basic building blocks of cloud computing, including networking, storage, and computing. It provides the closest abstraction to traditional IT resources and the most flexibility.
- PaaS
-
This option is a hosted service that takes care of all IT management and allows a developer to focus only on building the business logic. An excellent example of an AWS PaaS is AWS App Runner, which allows for continuous delivery of .NET applications with minimal effort. This type of offering removes the need to manage IT infrastructure. It allows an organization to focus on the deployment and management of applications.
- FaaS
-
FaaS is fascinating as a development paradigm because it allows a developer to work at the speed of thought. AWS Lambda is the canonical example of this model due to its automatic management of the compute resources.2 For a .NET developer, it is perhaps the most efficient way to deploy logic to the cloud since the code only runs when responding to an event.
- SaaS
-
This option provides a complete product available to purchase, freeing up an organization from needing to either host or develop this offering. An excellent example of this type of product is a logging and monitoring analysis service.
- Other (“as a service”)
-
There are newer “as a service” offerings made available by cloud computing. These services are discussed in more detail a little later in the book.
The general intuition is that IaaS means you pay less but have to do more, SaaS means you pay more but do less, and PaaS lies in the middle.3 This concept is similar to walking into a big warehouse store and either buying flour to make pizza, buying a frozen pizza, or buying a cooked slice of pizza at the counter on your way out. Whether we’re talking about pizza or software, you pay less but do more in situations where you have expertise and time to do things yourself. Alternatively, the convenience of higher level service, i.e., a hot slice of pizza, is worth leveraging the experts.
While the raw cost of IaaS may be less, it is also closer to the traditional way of doing things in physical data, which may not always mean it is cheaper. IaaS may not always be more affordable because the total ROI (return on investment) may be higher since you need to pay for highly trained staff to develop and manage applications on top of IaaS. Additionally, some traditional paradigms like running a web service in a virtual machine may cost more money than an event-driven AWS Lambda function that only runs when invoked.
Another way to describe an efficient event-driven service like AWS Lambda is to call it a cloud native service. Cloud native services are new offerings created due to efficiencies made possible by cloud computing and offer paradigms not available in traditional IT infrastructure, such as responding to web events versus running a web server 24/7. In concrete terms, an AWS Lambda function could run for just a few seconds of combined compute time per day. Meanwhile, a traditional web service would run 24/7 regardless of whether it’s receiving thousands of requests per second or just a dozen requests in a day. This concept is similar to the lights in a parking garage: you could waste electricity lighting an empty garage or you could use sensor lights that only turn on when motion triggers them. In this case, IaaS may be a more expensive solution when the ROI of the resources required to build it and the application’s run-time inefficiencies are fully considered in the cost.
Another way to refer to services that require little to no management on your part is managed services. An excellent example of a managed service is Amazon CloudSearch. The managed service in the AWS Cloud makes it simple and cost-effective to set up, manage, and scale a search solution for your website or application. Managed services are the opposite of IaaS, which require experts to set up, run, and maintain them.
Note
AWS uses the term managed services to refer to PaaS, SaaS, and FaaS services. You’ll likely only see the term managed services used in AWS documentation.
Next, let’s dive into how to get started with AWS.
Getting Started with AWS
An ideal place to get started with AWS is to sign up for a free tier account. When you initially create the account note, you need to set both an account name and the email address for the root user as shown in Figure 1-2.
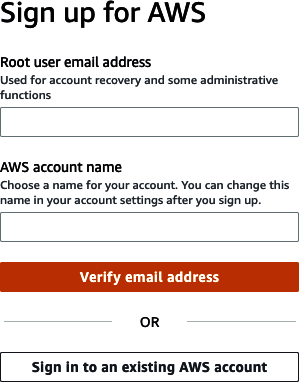
Figure 1-2. Sign up for AWS
Once you create an account, the first thing to do is to lock away your AWS account root user access keys.4 on how to lock your AWS account root user access keys.] You don’t want to use the root user for everyday tasks but instead create an IAM admin user.5
This is because if you expose the account credentials, it is possible to lose control of your AWS account. A fundamental security principle is the principle of least privilege (PLP), which states that you should “start with a minimum set of permissions and grant additional permissions as necessary.” Additionally, the root user account should enable AWS multifactor authentication (MFA) on the AWS root user account to review the step-by-step instructions on how to secure your account with MFA.6
Note
It is worth noting that a standard stumbling block in getting up to speed quickly with cloud computing is the terminology. Fortunately, AWS has a detailed and updated glossary of terms worth bookmarking.
A straightforward way to use AWS is via the AWS web console. Let’s tackle this next.
Using the AWS Management Console
The AWS Management Console, as shown in Figure 1-3, is the central location to control AWS from a web browser.7 The Services tab shows a hierarchical view of every service on the platform. Adjacent to this tab is a search box that allows you to search for a service. We find ourselves using the search box frequently, as it is often the quickest way to navigate to a service. Adjacent to the search box is the AWS CloudShell icon, which appears as a black and white terminal icon. This service is a great way to quickly try out commands on the AWS platform, like listing S3 buckets—containers for object storage—in your account or running a command against a managed natural language processing API like AWS Comprehend.
Note
Conceptually, an S3 bucket is similar to consumer cloud storage like Dropbox, Box, or Google Drive.
The circled N. Virginia tab shows the AWS region currently used. It is common to toggle to different regions to launch virtual machines or try services in another region. Often, but not always, new services first appear in the N. Virginia region, so depending on what region your organization is using, they may need to toggle to N. Virginia to try out a new service and then back again. Likewise, suppose a new service appears in a different region like US West (Oregon). You may find yourself trying that new service out in that region and then needing to toggle back to your primary region.
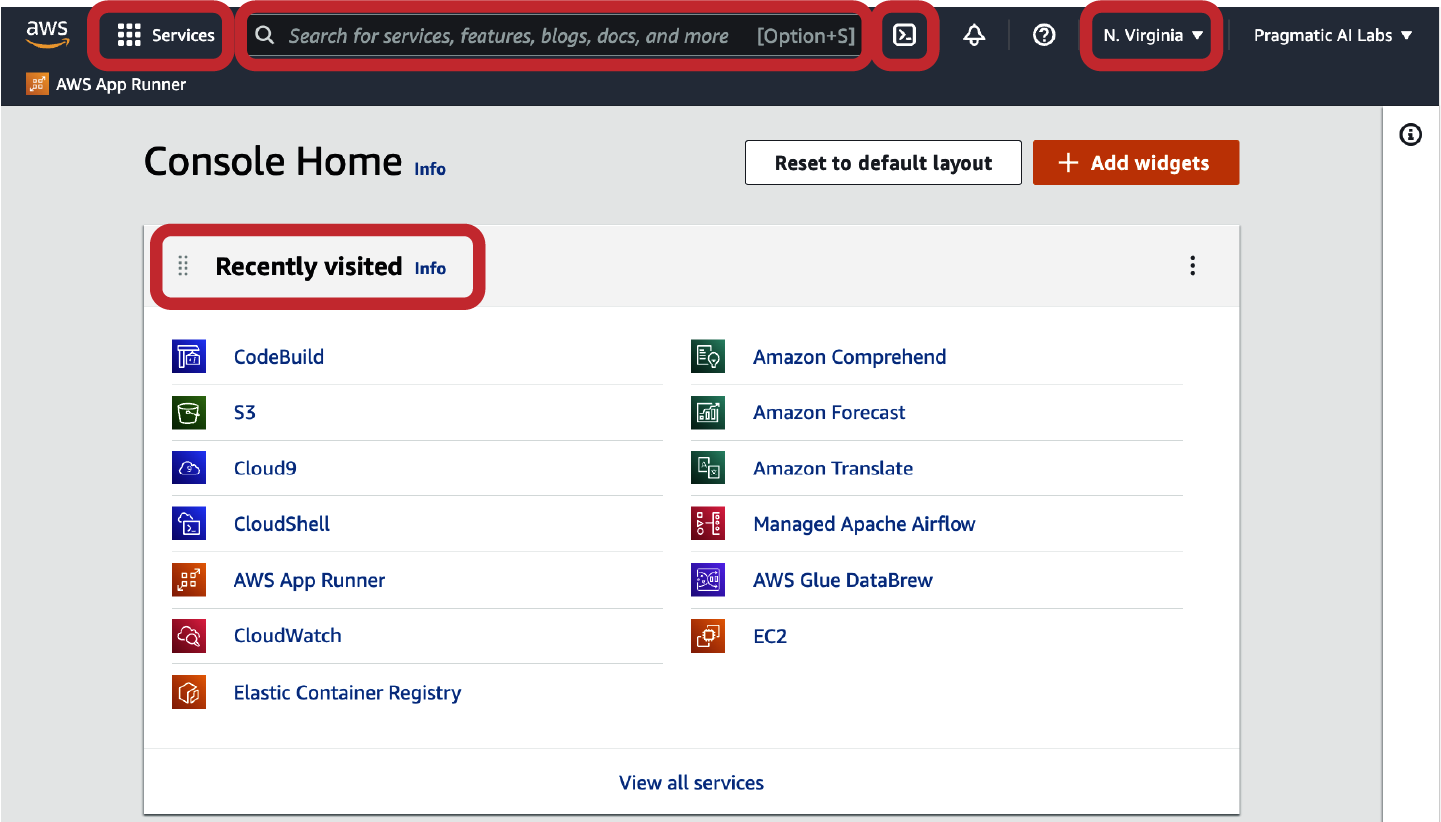
Figure 1-3. Using the AWS Console
Note
The cost of services and transferring data out of AWS may vary between regions.
Finally, the Recently visited tab shows services recently used. After prolonged use of AWS, it is common to have most of the daily services appear in this section, providing a helpful shortcut menu to use AWS. Another thing to note is that you can “star” an item in the console to add it to the Favorites tab, as shown in Figure 1-4. This process can be a convenient way to access services you use often.
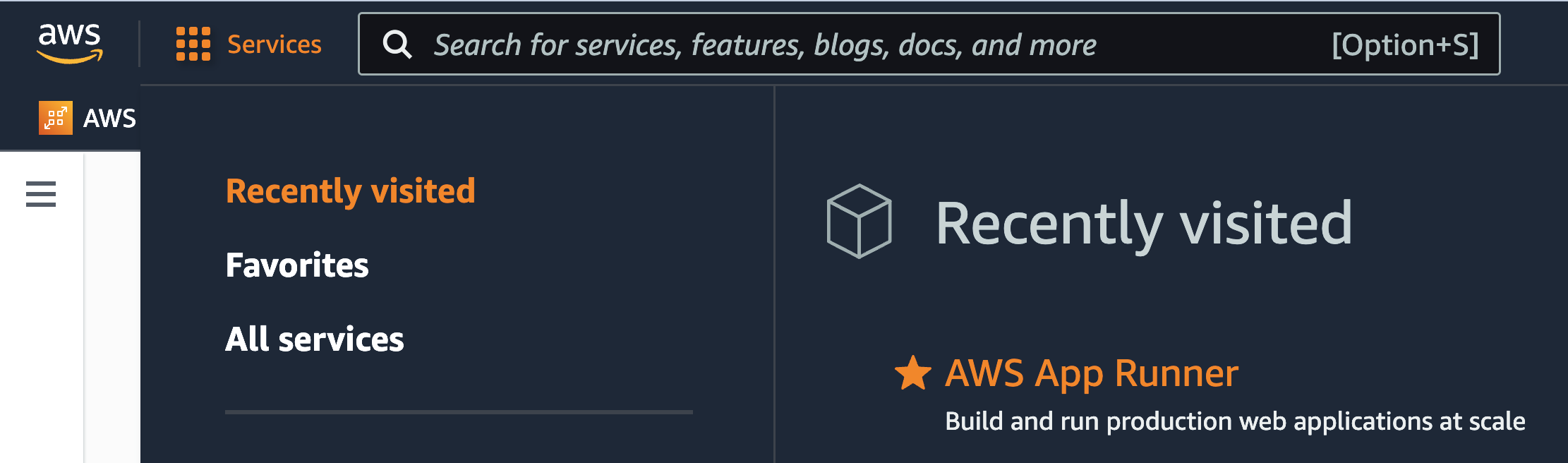
Figure 1-4. Favorites in the AWS Management Console
Next, let’s look at utilizing the AWS communities and documentation resources.
Utilizing the AWS Communities and Documentation
A tremendous variety of documentation is available for learning about the AWS platform in various forms and depth. Let’s discuss these resources next.
The .NET on AWS website
The .NET on AWS website is the central location for information about using .NET with AWS. From here, readers can find service and SDK documentation, AWS toolkits and migration tools, getting started tutorials, developer community links, and other content.
It is wise for a .NET developer to bookmark this website since it has a curated view of helpful resources. One of my favorite sections is the .NET Community tab. This section has a link to many popular developer communities, as shown in Figure 1-5 including Twitter, re:Post, Stack Overflow, Slack, GitHub, and Global AWS User Groups.8
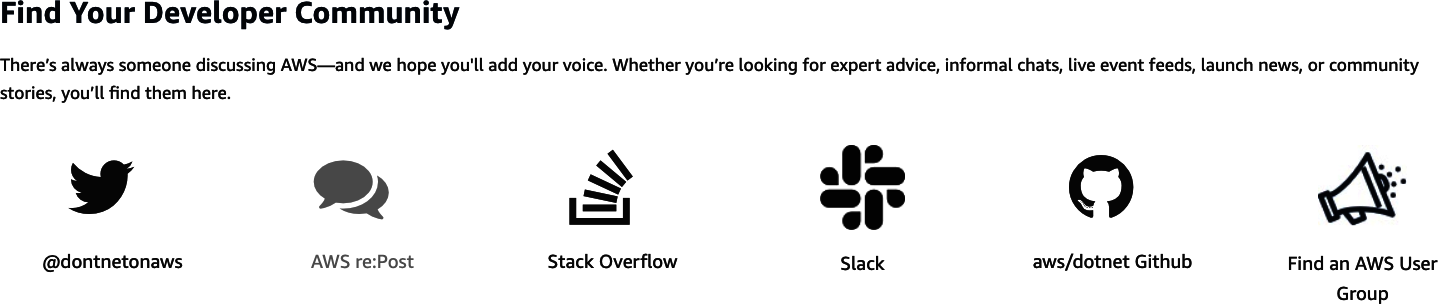
Figure 1-5. Developer communities for .NET
One of the more valuable sections of the community portal is the links to YouTube channels and developer blogs. Finally, there are links to open source .NET projects that AWS invests in, including .NET on AWS and AWS SDK for .NET.
Next, let’s look at the AWS SDK for .NET documentation.
AWS SDK for .NET documentation
You can learn to develop and deploy applications with the AWS SDK for .NET at the official website. This website includes several essential guides, including the Developer Guide, the API Reference Guide, and the SDK Code Examples Guide.
Another essential resource is the necessary development .NET tools on AWS, shown in Figure 1-6. These include AWS Toolkit for Rider, AWS Toolkit for Visual Studio, AWS Toolkit for Visual Studio Code, and AWS Tools for Azure DevOps.

Figure 1-6. Explore .NET tools on AWS
We’ll dig into the AWS SDK later in the chapter. Next, let’s take a look at the AWS service documentation.
AWS service documentation
AWS has so many services that it can be overwhelming at first to understand which service is appropriate for the task at hand. Fortunately, there is a centralized web page, the AWS documentation website, containing guides and API references, tutorials and projects, SDKs and toolkits, as well general resources like FAQs and links to case studies.
One item to call out is that AWS has many SDKs and toolkits, as shown in Figure 1-7, including the AWS CLI. It can be helpful for a .NET developer to see examples in many languages to get ideas for solutions you can build .NET. The AWS Command Line Interface is often the most efficient documentation for a service since it abstracts the concepts at a level that makes it easy to understand what is going on. An excellent example of this concept is the following command to copy a folder recursively into AWS S3 object storage:
aws s3 cp myfolder s3://mybucket/myfolder --recursive
Each whitespace separates the command’s actions, i.e., aws
then s3
for the service, the action s3
, and the rest of the command. There is no quicker way to learn a service on AWS than to invoke it from the command line in many cases.
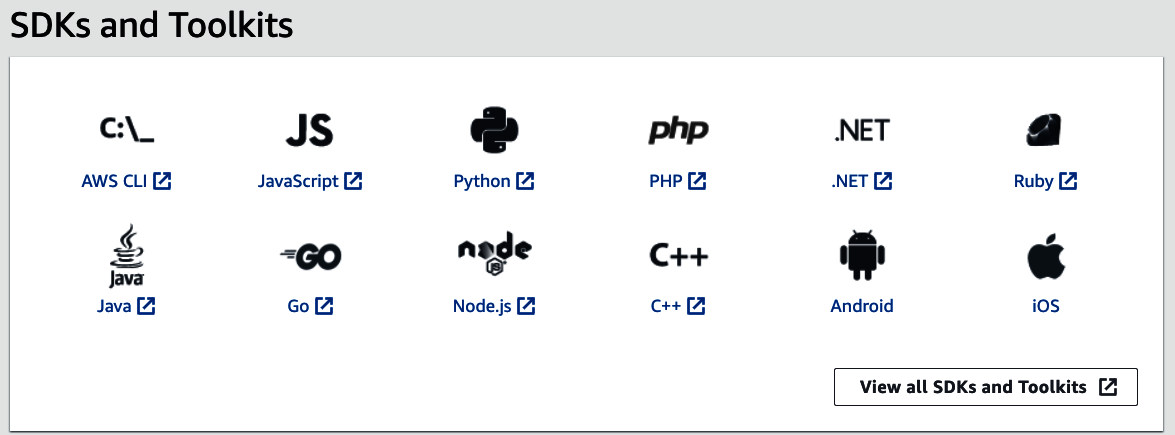
Figure 1-7. Explore AWS SDK and toolkits
Next, let’s talk about installing the AWS Command Line.
Using the AWS Command Line Interface
According to the official AWS documentation, “The AWS Command Line Interface (CLI) is a unified tool to manage your AWS services. With just one tool to download and configure, you can control multiple AWS services from the command line and automate them through scripts.”
There are four essential methods to interact with the AWS Command Line Interface.9 Let’s discuss each briefly.
- AWS CloudShell
-
AWS CloudShell is a cloud native browser-based shell, meaning that it takes advantage of the unique properties of the cloud by preinstalling the AWS CLI for you. You can choose between the Bash shell, PowerShell, or Z shell.
- AWS Cloud9
-
AWS Cloud9 is a cloud native interactive development environment (IDE) that has deep hooks into AWS with unique development tools suited to developing not just for the cloud but inside the cloud itself.
- Linux shells
-
By using the Amazon Linux 2 AMI, you automatically get access to the command-line tools for AWS integration. Alternatively, you can install the AWS CLI on any Linux system.
- Windows command line
-
Managed AWS Windows AMIs come with AWS CLI. Alternatively, you can download and run the 64-bit Windows installer.
Next, let’s discuss the installation notes about each of these methods.
Note
You can watch a more detailed walk-through of using the AWS CloudShell, including interacting with Bash, ZSH, PowerShell, S3, Lambda, Python, IPython, Pip, Boto3, DynamoDB, and Cloud9 on YouTube or O’Reilly.
- How to install AWS CloudShell and AWS Cloud9
-
There is no installation process necessary for AWS CloudShell and AWS Cloud9 because they are already included in the AWS Console experience, as shown in Figure 1-8. Notice that searching for both tools brings them both up in search results, allowing you to “star” (i.e., add them as favorites) or click on them. Finally, the terminal icon can also launch AWS CloudShell. One key advantage of these cloud native terminals is they are fully managed and up-to-date and automatically manage your credentials.
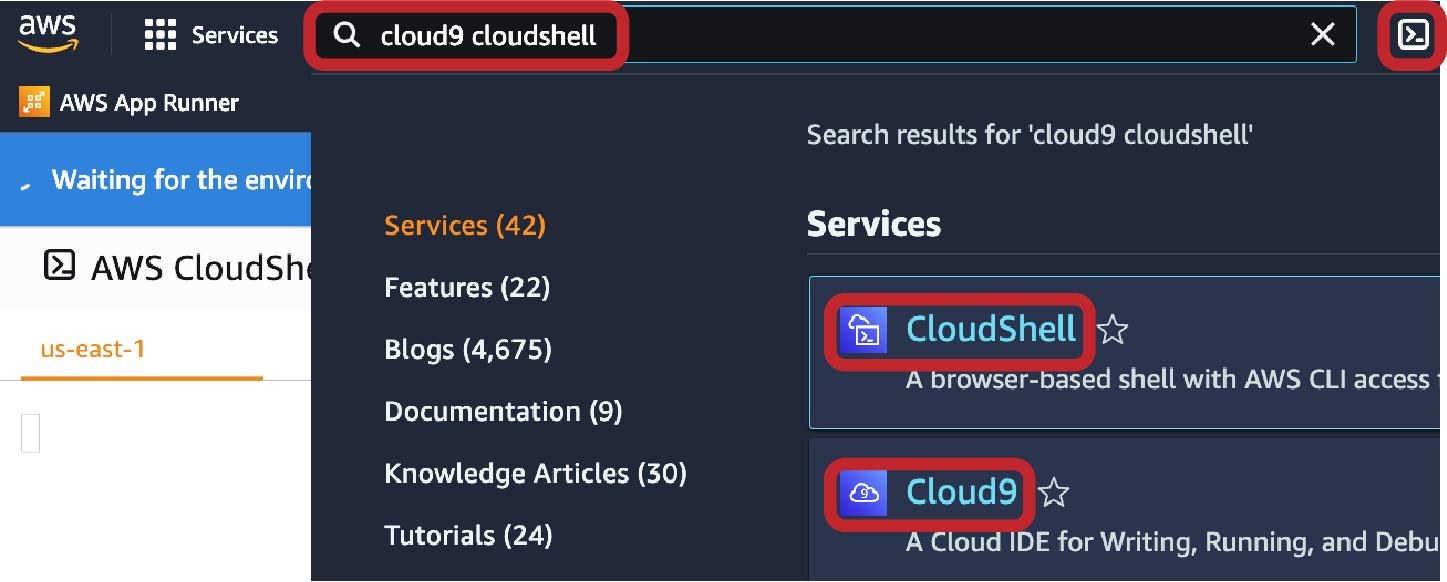
Figure 1-8. Selecting AWS Cloud9 and AWS CloudShell
- How to install the AWS CLI
-
The AWS CLI installation comes with AWS Cloud9 or AWS CloudShell. If you need to install the AWS CLI locally on Windows, a detailed guide is on the AWS website.
- How to install AWSPowerShell.NETCore for AWS
-
If you need to run PowerShell for AWS locally on Windows, Linux, or macOS, you can refer to the PowerShell Gallery to find the latest version of AWSPowerShell.NETCore and installation instructions for Windows, Linux, and macOS. AWSPowerShell.NETCore is the recommended version of PowerShell for working with AWS because it has comprehensive cross-platform support. Generally, though, you can install the following PowerShell command:
Install-Module -Name AWSPowerShell.NetCore
.
An alternative to installing PowerShell on a local operating system is using AWS CloudShell since it comes preinstalled. Next, let’s talk about how this process works.
Using PowerShell with AWS CloudShell
An excellent guide for using AWS Tools for PowerShell lives on the AWS website. Let’s look at a simple example to supplement the official documentation. In the following example, as shown in Figure 1-9, we create an AWS bucket by invoking the New-S3Bucket
cmdlet to create a new Amazon S3 bucket.
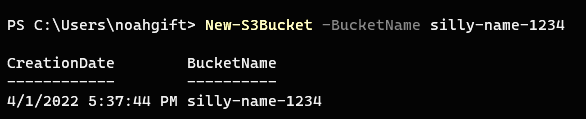
Figure 1-9. Create AWS bucket in AWS PowerShell
Next, we can go to the AWS Console, select S3, and verify it appears, as shown in Figure 1-10.

Figure 1-10. Show AWS bucket in AWS S3 Console
It is worth mentioning that PowerShell for AWS offers a sophisticated and high-level interface to control and interact with services on AWS, including EC2, S3, Lambda, and more. You can further explore those in the AWS documentation.
The AWS CloudShell supports three shells: PowerShell, Bash, and Z shell. To use PowerShell, you type pwsh
. You can explore all AWS CloudShell features using the official documentation. The AWS CloudShell comes with 1 GB of persistent storage in /home/cloudshell-user. The action menu, as shown in Figure 1-11, allows you to perform many practical actions, including downloading and uploading files, restarting the terminal, deleting the data in the home directory, and configuring the tabs layout of the terminal.
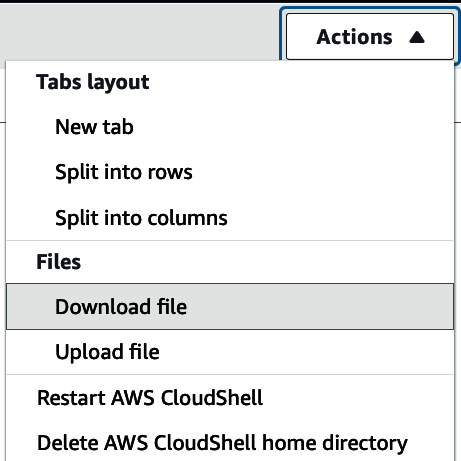
Figure 1-11. AWS CloudShell action menu
Fortunately, the AWS CloudShell PowerShell is straightforward, similar to local PowerShell. First, to use AWSPowerShell.NetCore
, import it as shown in the following sequence, echo content into a file, and write it to the bucket created earlier. Finally, run Get-S3Object
to verify the file’s creation in S3, which you can see in Figure 1-12.
Import-Module
AWSPowerShell
.
NetCore
echo
"This is data"
>
data
.
txt
Write-S3Object
-BucketName
silly-name
-
1234
-File
data
.
txt
Get-S3Object
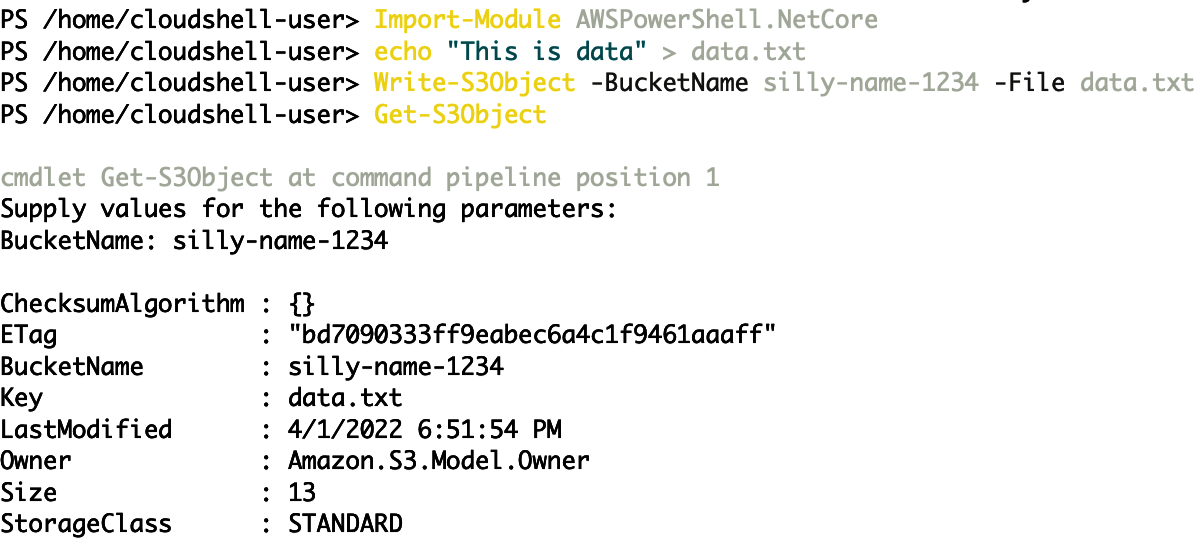
Figure 1-12. AWS CloudShell create file S3
Another feature of the CloudShell and PowerShell environment is invoking C# code. To get started using PowerShell with C#, you can create a C# snippit and embed it in a script called ./hello-ps-c-sharp.ps1. The C# code comes after the $code = @"
block and then terminates at "@
. The general idea is that if you are using PowerShell inside of AWS CloudShell, you can use existing snippets of helpful C# to enhance a PowerShell script without needing a full-fledged editor:
$
code
=
@"
using System;
namespace HelloWorld
{
public class Program
{
public static void Main(){
Console.WriteLine("
Hello
AWS
Cloudshell
!
");
}
}
}
"@
Add
-
Type
-
TypeDefinition
$
code
-
Language
CSharp
iex
"[HelloWorld.Program]::Main()"
Install
-
Module
-
Name
AWS
.
Tools
.
Installer
-
Force
Next, run it in your PowerShell prompt:
PS /home/cloudshell-user> ./hello-ps-c-sharp.ps1 Hello AWS Cloudshell! PS /home/cloudshell-user>
Consider using Bash and PowerShell, depending on which one makes more sense in a given context. If you are doing something with pure .NET capabilities, then PowerShell is an easy win. On the other hand, you may find some documentation for scripting a solution with Bash on the AWS website. Instead of rewriting the example, simply use it and extend in Bash, saving you time.
In the following example, we use a Bash hash to store a list of Cloud9 IDs and then get information about them:
#!/usr/bin/env bash
# Loop through a list of Cloud9 Environments to find out more information
declare
-Acloud9Env
=([
env1]=
"18acd120518340df8a73ccaab641851e"
\
[
env2]=
"2c9eb66bf53b4083b9ab6345bae70dad"
\
[
env3]=
"f104b0141c284a41af0c75fea7890770"
)
## now loop through the above hash to get more information
for
envin
"
${
!cloud9Env[@]
}
"
;
do
echo
"Information for
$env
: "
aws cloud9 describe-environments --environment-id"
${
cloud9Env
[
$env
]
}
"
done
We don’t have to use Bash in this particular situation because there is excellent documentation on how to solve the problem in PowerShell for AWS. PowerShell can do the same thing as Bash and has deep C# integration. Notice the following example shows the PowerShell method of looping through several IDs stored in a hash:
# PowerShell script that loops through Cloud9 to get environment information
$cloud9Env
=
@{
env1
=
"18acd120518340df8a73ccaab641851e"
;
env2
=
"2c9eb66bf53b4083b9ab6345bae70dad"
;
env3
=
"f104b0141c284a41af0c75fea7890770"
}
foreach(
$env
in
$cloud9Env
.GetEnumerator())
{
Write-Host"Information for
$(
$env
.Key)
:"
;
Get-C9EnvironmentData$(
$env
.Value)
}
To summarize, the AWS CloudShell is a great companion for the C# developer, and it is worth having in your toolkit. Now let’s use Visual Studio for AWS, a preferred environment for experienced .NET developers who use AWS.
Using Visual Studio with AWS and AWS Toolkit for Visual Studio
Getting set up with Visual Studio with AWS is a straightforward process. The required components are an AWS account, a machine running a supported version of Windows, Visual Studio Community Edition or higher, and AWS Toolkit for Visual Studio. Please refer to this official AWS documentation for further details on setting up Visual Studio for AWS if this is your first time configuring it.
Note
If you want to use the latest .NET 6 features, you need to use Visual Studio 2022. In practice, your best experience for Visual Studio with deep AWS integration is a Windows environment running the latest version of Visual Studio. For example, a Mac version of Visual Studio exists, but the AWS Toolkit does not work on Mac.
The AWS SDK for .NET has deep integration with the Visual Studio environment, as shown in Figure 1-13. It includes interacting with AWS Core Services, including Amazon S3 and Amazon EC2, through the AWS Explorer integration installed as part of AWS Toolkit for Visual Studio.
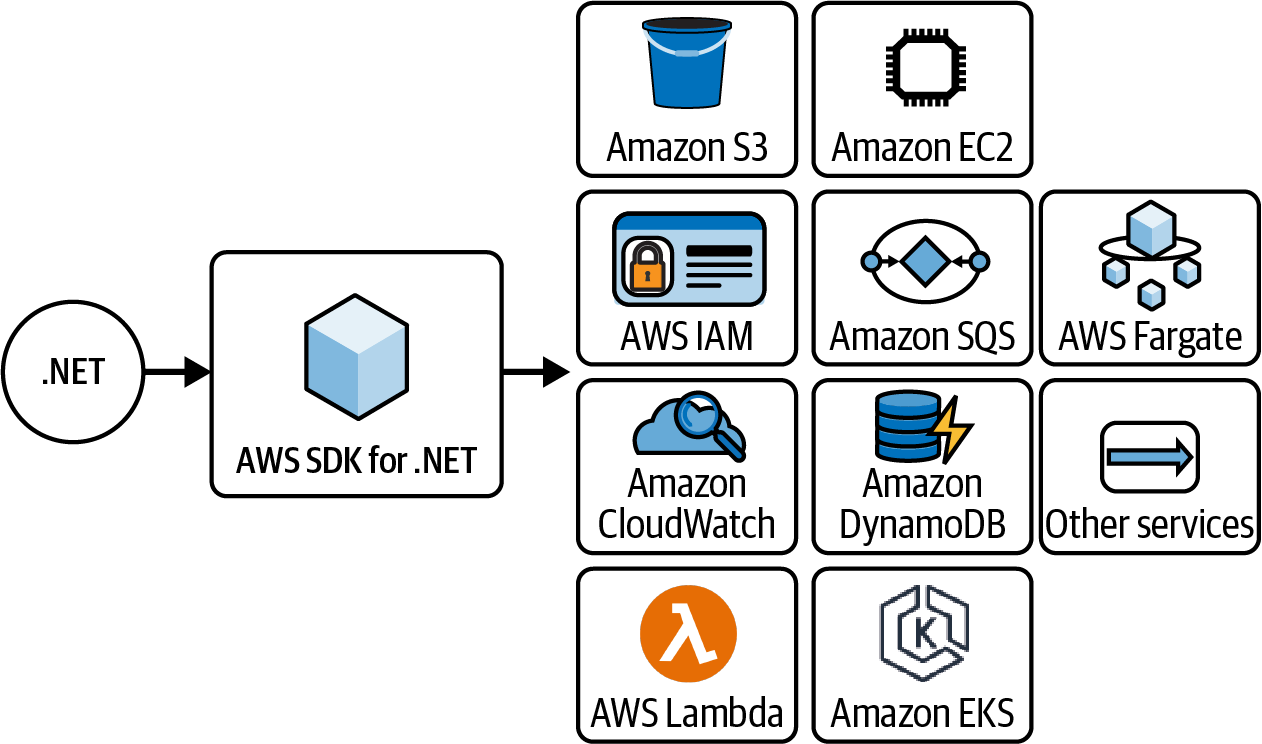
Figure 1-13. AWS SDK for .NET
According to the official AWS documentation, the AWS Toolkit for Visual Studio is an extension for Microsoft Visual Studio running on Microsoft Windows that makes it “easier for developers to develop, debug, and deploy .NET applications using Amazon Web Services.” This integration includes multiple ways to deploy applications and manage services. A component of this integration manifests itself in the Server Explorer Toolbox, or AWS Explorer, as shown in Figure 1-14. The AWS Explorer lets you manage and interact with AWS resources like Amazon S3 or EC2 instances within a Microsoft Windows Visual Studio environment.
Notice how straightforward it is to dive into many popular AWS services with a mouse click.
Visual Studio is a popular development for .NET for two reasons: it is both a fantastic editor and a rich ecosystem for .NET developers. The AWS Toolkit for Visual Studio taps into this ecosystem as an extension for Microsoft Visual Studio on Windows, making it easier for developers to develop, debug, and deploy .NET applications using Amazon Web Services. You can see a complete list of the features available by reviewing the installer’s official documentation.
Note
Note that Visual Studio Code and JetBrains Rider both have AWS Toolkits.
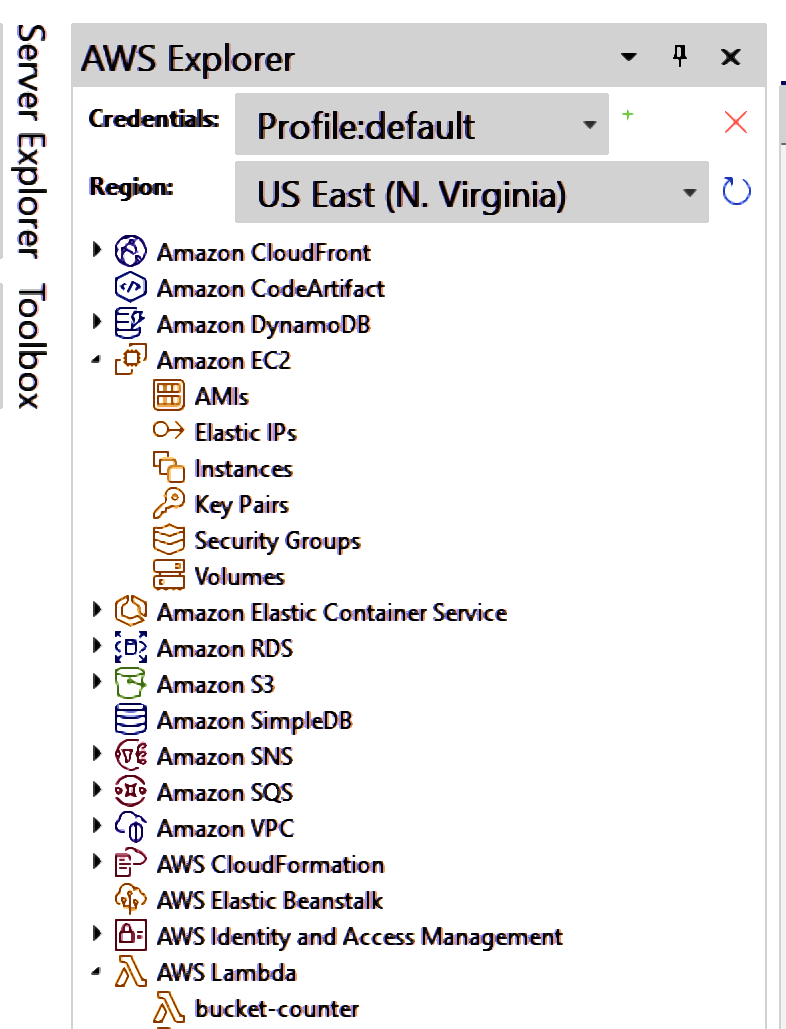
Figure 1-14. AWS Explorer
The AWS Explorer installation does require three key steps:10
-
Install the AWS Toolkit for Visual Studio
Download the AWS Toolkit for Visual Studio 2022 and install the package on your Windows machine that has a local installation of Visual Studio.
-
Create an IAM user and download credentials
Create an IAM user in the AWS Console and apply the principle of least privilege.11 Ensure that you select the access key option, as shown in Figure 1-15, and then download the credentials in CSV format when prompted.
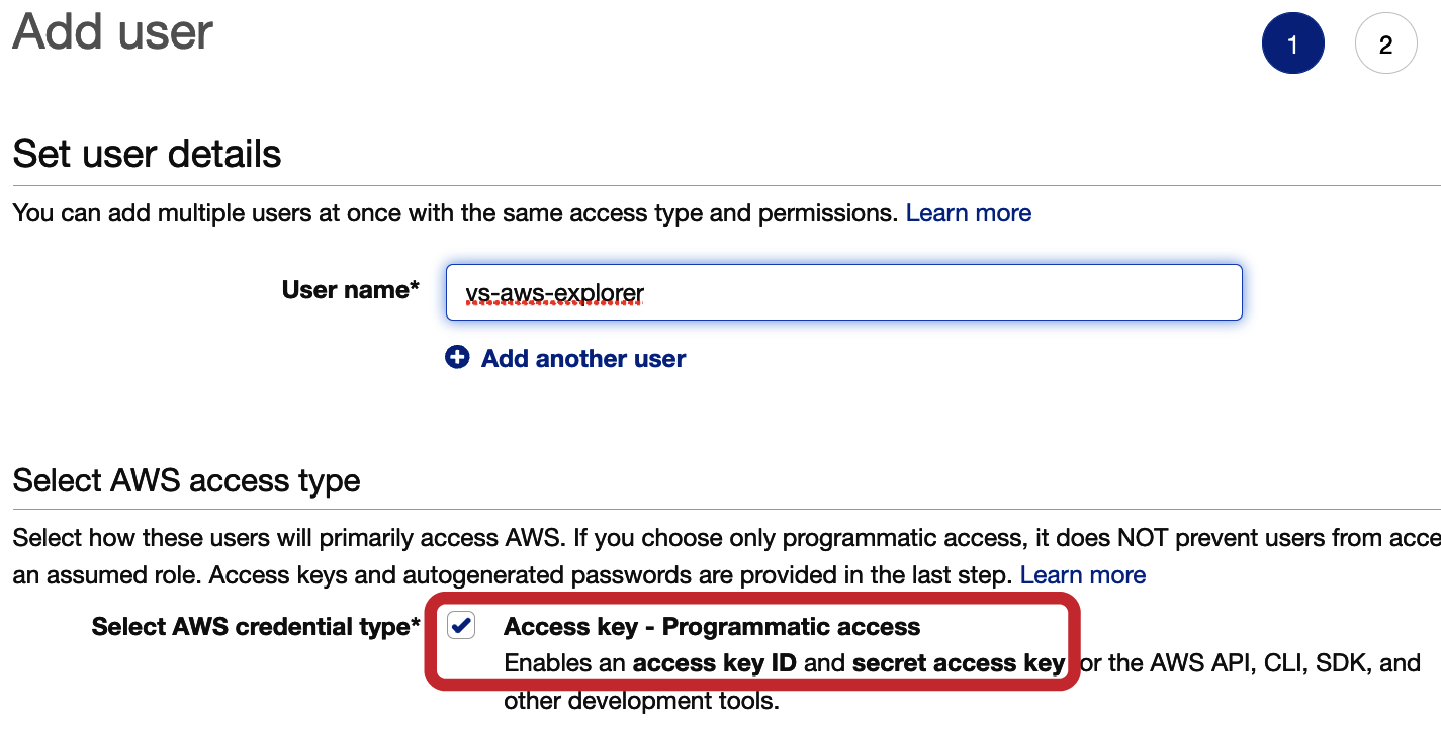
Figure 1-15. Create an IAM user for Visual Studio
-
Add credentials to Visual Studio
Finally, add the credentials you downloaded in CSV form to AWS Explorer, as shown in Figure 1-16.
Note
Extra special consideration is needed in downloading keys from AWS to use on any local development machine because if they are compromised, the privileges of those keys are available to whoever owns them. Using the PLP, a developer should select IAM privileges that correspond only with the functions that are strictly necessary to develop the solutions you create on AWS.
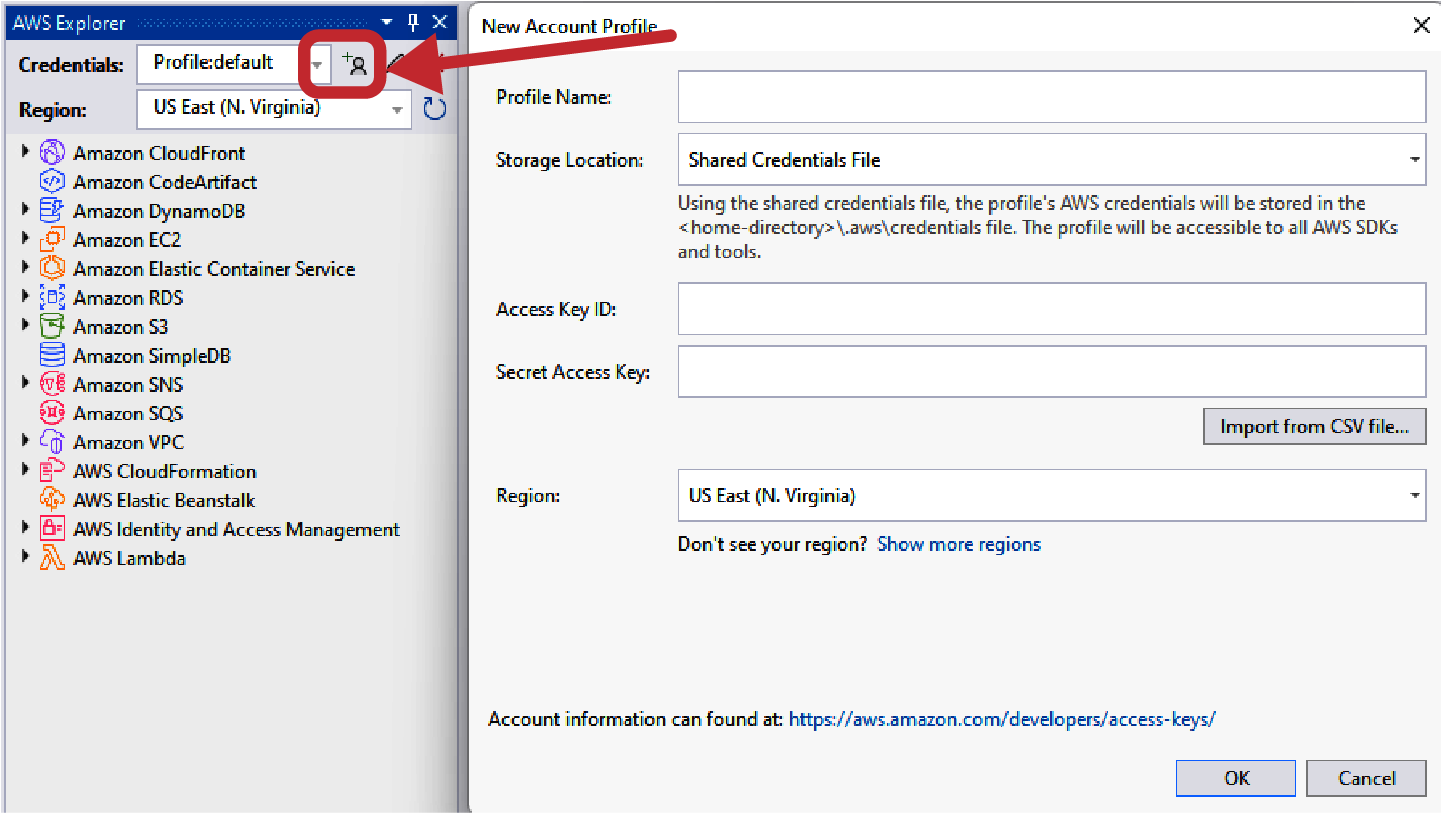
Figure 1-16. Add credentials
Once AWS Toolkit for Visual Studio installs, it is also good to be vigilant about updating the tool shown in Figure 1-17 since it is actively under development with new features from AWS. You will see new updates to the AWS Toolkit for Visual Studio as notifications in Visual Studio.
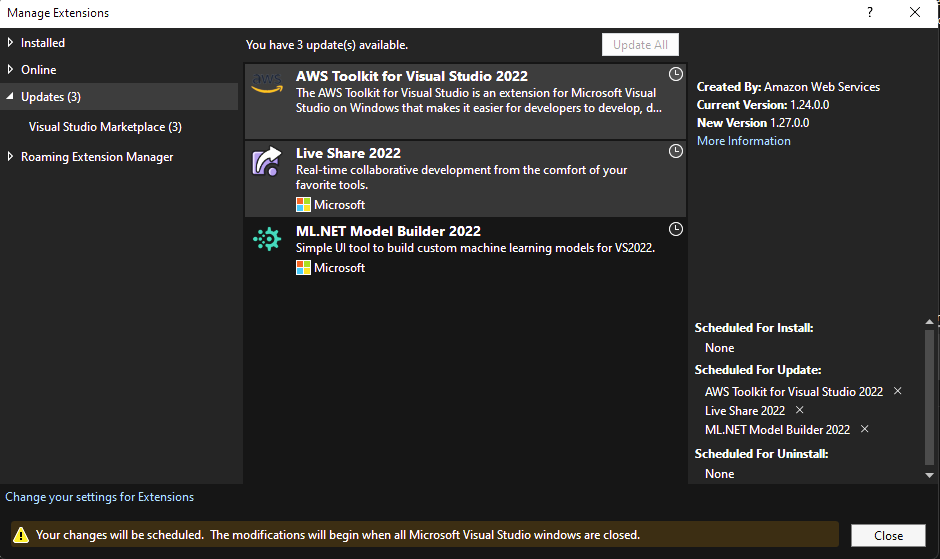
Figure 1-17. Upgrading AWS Toolkit for Visual Studio
Next, let’s look at getting started with the AWS SDK.
Getting Started with the AWS SDK
The best method to learn a new technology is to build something. Let’s get started with the AWS SDK by making a Console App that targets .NET 6 using the AWSSDK.S3 package from NuGet. The steps are as follows:
-
Create a new Console App targeting .NET 6.
Inside Visual Studio, create a new Console Application that serves as the vessel for the AWS S3 tool developed.
-
Install AWSSDK.S3 via NuGet.
With the newly created Console App loaded, choose Tools, then NuGet Package Manager, then Manage NuGet Packages for Solution. Search for AWSSDK.S3 and install it into your Console Project.
-
Create the code.
Build the following Console App by replacing the Program.cs file with the content shown in the following example.
Tip
Another way to install NuGet Packages is to right-click on the project and select Manage NuGet Packages.
using
System
;
using
System.Threading.Tasks
;
// To interact with Amazon S3.
using
Amazon.S3
;
using
Amazon.S3.Model
;
// Create an S3 client object.
var
s3Client
=
new
AmazonS3Client
();
// Display Prompt
Console
.
WriteLine
(
"AWS Bucket Lister"
+
Environment
.
NewLine
);
// Process API Calls Async List AWS Buckets
var
listResponse
=
await
s3Client
.
ListBucketsAsync
();
Console
.
WriteLine
(
$
"Number of buckets: {listResponse.Buckets.Count}"
);
// Loop through the AWS buckets
foreach
(
S3Bucket
b
in
listResponse
.
Buckets
)
{
Console
.
WriteLine
(
b
.
BucketName
);
}
Note
For fans of the command line, it is worth noting that you can also use the dotnet
command-line tool to create a Console Application as in the following snippet: dotnet new console --framework net6.0
.12
The Console App result then details a list of S3 buckets that the AWS user who has run the .NET application had, as shown in Figure 1-18.
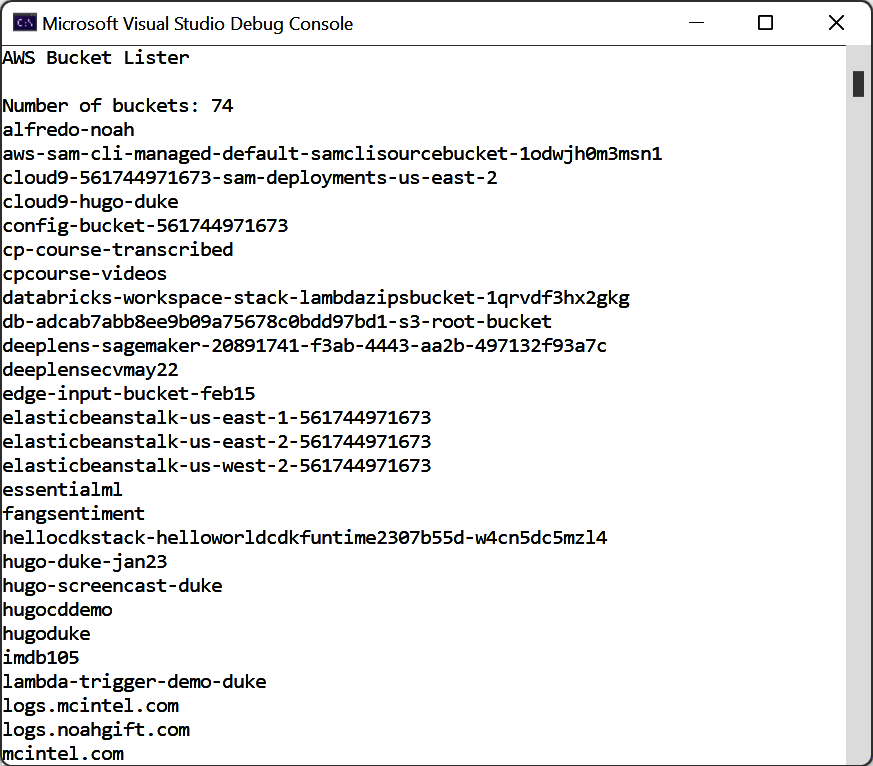
Figure 1-18. Async listing buckets
Note
You can also watch a walk-through of building this S3 Console Application from scratch on O’Reilly or YouTube.
A follow-up step to this Console Application would be to extend the functionality to create buckets and not just list them, as shown in this AWS tutorial for the AWS SDK for .NET.
Next, let’s wrap up everything covered in this chapter.
Conclusion
Cloud computing is a crucial driver of innovation in technology because it opens up more efficient ways to work.13 By leveraging near-infinite resources and cloud native managed services, developers can enhance their productivity by focusing on the problem at hand versus issues unrelated to building a solution, like systems administration. This chapter covered how to start working with Amazon Cloud Services, first using a CloudShell, then moving to a full-fledged Visual Studio code environment.
The Visual Studio environment benefits from the AWS Toolkit, which has advanced features for interacting with the AWS Cloud. The AWS SDK is available directly inside Visual Studio via the NuGet package manager. The AWS SDK allows for straightforward integration into Visual Studio solutions like Console Applications. We used the AWS SDK to build out Console Applications with low-level services like AWS S3 and high-level AI APIs like AWS Comprehend.
A recommended next step for readers is to view the critical thinking discussion questions to reflect on how you could leverage the full power of AWS. You can take these questions and discuss them with your team or a coworker learning AWS. Additionally, several challenge exercise suggestions below serve as good practice for building AWS services that target .NET 6. Some of these services are not covered in detail yet, so you are welcome to skip them until they are covered in later chapters.
Next up, in Chapter 2, we cover AWS Core Services. These include AWS Storage, EC2 Compute, and services like DynamoDB. These services are the foundation for building cloud native applications that leverage the power of the .NET ecosystem.
Critical Thinking Discussion Questions
-
What are the key differences between using Bash versus PowerShell in the AWS Cloudshell?
-
When would it be advantageous to use PowerShell versus Bash and vice versa?
-
How could mastering the command-line
dotnet
tools improve productivity? -
Could the AWS Toolkit for Visual Studio benefit even nondevelopers in your organization, e.g., the operations team? Why or why not?
-
What are the advantages of prototyping C# web services by deploying right from Visual Studio?
Challenge Exercises
-
Build a C# or an F# Console App and deploy to AWS CloudShell. Consider how the unique properties of a cloud-based development environment could help you automate workflows on the AWS Cloud, considering they don’t require API keys and are available without needing to deploy to AWS compute services to run.
-
Refer to the AWS CDK14 in C# documentation and create an S3 bucket via CDK.
-
Build and deploy an Elastic Beanstalk application using Visual Studio and the AWS Toolkit for Visual Studio Code.
-
Install one of the 600+ packages available for AWS with NuGet inside of Visual Studio.
-
Create a hello world AWS Lambda function using the AWS Console in any supported language, then invoke it via VS Code and the AWS Toolkit for Visual Studio Code.
1 You can read more about EFS and spot instances in Chapter 6 in Python for DevOps (O’Reilly).
2 The AWS whitepaper “Developing and Deploying .NET Applications on AWS” explains AWS Lambda’s mechanisms.
3 You can read more detail about how AWS sees cloud computing in their “Overview of Amazon Web Services” whitepaper.
4 The AWS Identity and Access Management guide has a detailed list of https://oreil.ly/x90WL
5 To create an admin IAM user, follow the official AWS guide.
6 See the AWS multifactor authentication guide.
7 You can read more about the AWS Management Console in the in the official documentation.
8 Additionally, there are links to famous .NET developers like Norm Johanson, François Bouteruche, and Steve Roberts.
9 There is a detailed user guide to interacting with the AWS Command Line Interface.
10 There is a detailed set-up guide available for AWS Toolkit for Visual Studio.
11 There is a detailed guide about the concept of principle of least privilege (PLP) in the AWS documentation. The core idea of PLP is only to give out permissions when needed.
12 Other services like AWS Lambda also offer a convenient way to create an application from the command line.
13 In part, Amazon is the leader in cloud computing due to its culture, which describes itself in its leadership principles. These principles include frugality, customer obsession, and invent and simplify, among a few. You can read more about Amazon’s leadership principles.
14 AWS CDK is a library that automates the deployment of resources in AWS.
Get Developing on AWS with C# now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.