Chapter 1. Software Architecture Demystified: Let’s Get Started!
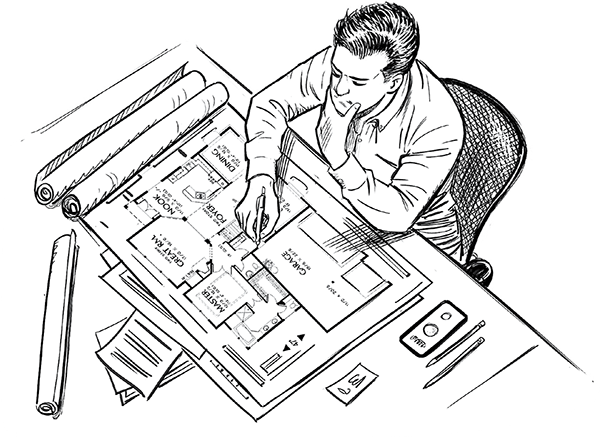
Software architecture is fundamental to the success of your system. This chapter demystifies software architecture. You’ll gain an understanding of architectural dimensions and the differences between architecture and design. Why is this important? Because understanding and applying architectural practices helps you build more effective and correct software systems—systems that not only function better, but also meet the needs and concerns of the business and continue to operate as your business and technical environments undergo constant change. So, without further delay, let’s get started.
Building your understanding of software architecture
To better understand software architecture, think about a typical home in your neighborhood. The structure of the home is its architecture —things like its shape, how many rooms and floors it has, its dimensions, and so on. A house is usually represented through a building plan, which contains all the lines and boxes necessary to know how to build the house. Structural things like those shown below are hard and expensive to change later and are the important stuff about the house.
Note
The building metaphor is a very popular one for understanding software architecture.
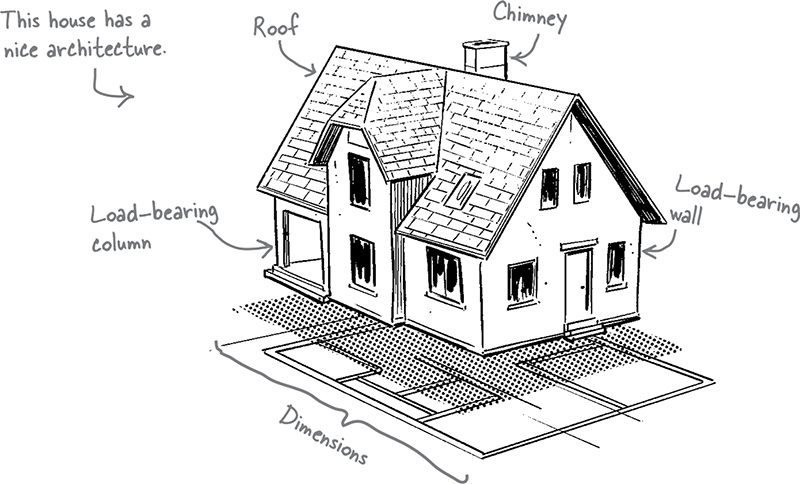
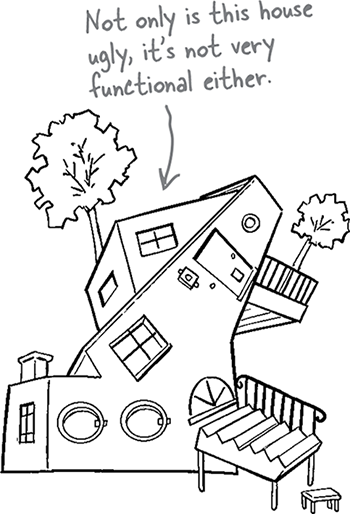
Architecture is essential for building a house. Can you imagine building one without an architecture? It might turn out looking something like the house on the right.
Architecture is also essential for building software systems. Have you ever come across a system that doesn’t scale, or is unreliable or difficult to maintain? It’s likely not enough emphasis was placed on that system’s architecture.
Building plans and software architecture
You might be wondering how the building plans of your home relate to software architecture. Each is a representation of the thing being built. So what does the “building plan” of a software system look like? Lines and boxes, of course.
A building plan specifies the structure of your home—the rooms, walls, stairs, and so on—in the same way a software architecture diagram specifies its structure (user interfaces, services, databases, and communication protocols). Both artifacts provide guidelines and constraints, as well as a vision of the final result.
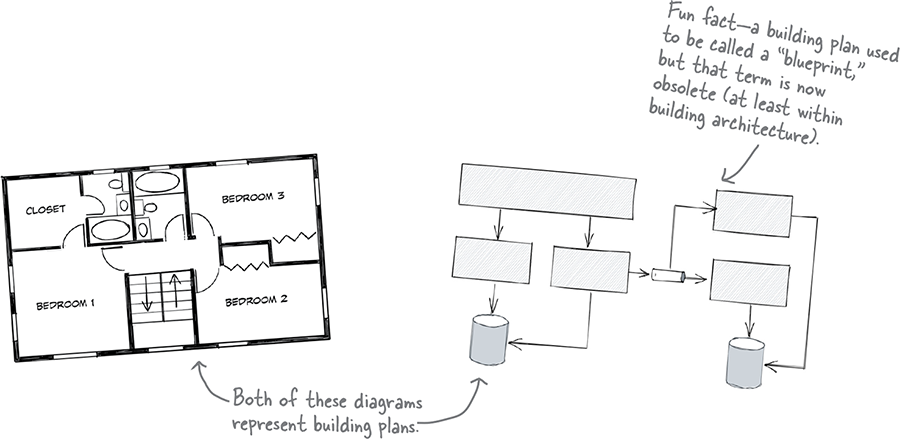
Did you notice that the floor plan for the house above doesn’t specify the details of the rooms—things like the type of flooring (carpet or hardwood), the color of the walls, and where a bed might go in a bedroom? That’s because those things aren’t structural. In other words, they don’t specify something about the architecture of the house, but rather about its design.
Note
Don’t worry—you’ll learn a lot more about this distinction later in this chapter. Right now, just focus on the structure of something—in other words, its architecture.
The dimensions of software architecture
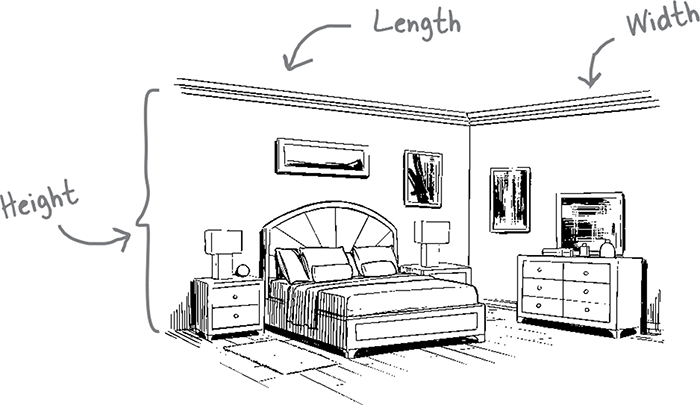
Most things around us are multidimensional. For example, you might describe a particular room in your home by saying it is 5 meters long and 4 meters wide, with a ceiling height of 2.5 meters. Notice that to properly describe the room you needed to specify all three dimensions—its height, length, and width.
You can describe software architecture by its dimensions, too. The difference is that software architecture has four dimensions.
Architectural characteristics
This dimension describes what aspects of the system the architecture needs to support—things like scalability, testability, availability, and so on.
Architectural decisions
This dimension includes important decisions that have long-term or significant implications for the system—for example, the kind of database it uses, the number of services it has, and how those services communicate with each other.
Logical components
This dimension describes the building blocks of the system’s functionality and how they interact with each other. For example, an ecommerce system might have components for inventory management, payment processing, and so on.
Architectural style
This dimension defines the overall physical shape and structure of a software system in the same way a building plan defines the overall shape and structure of your home.
Note
You’ll learn about five of the most common architectural styles later in this book.
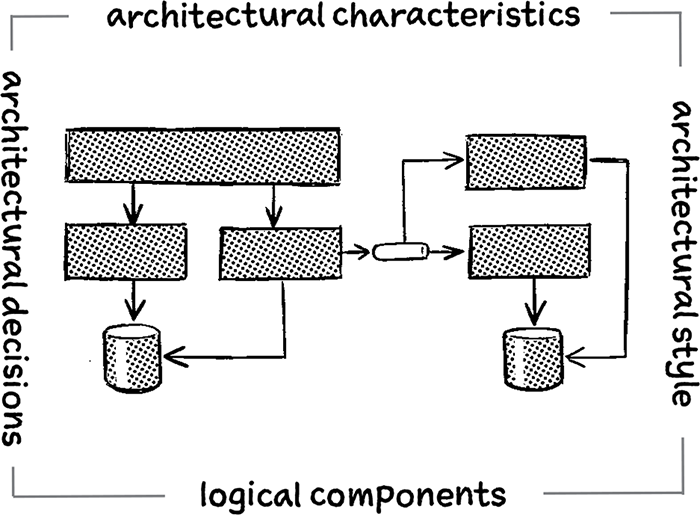
Puzzling out the dimensions
You can think of software architecture as a puzzle, with each dimension representing a separate puzzle piece. While each piece has its own unique shape and properties, they must all fit together and interact to build a complete picture.
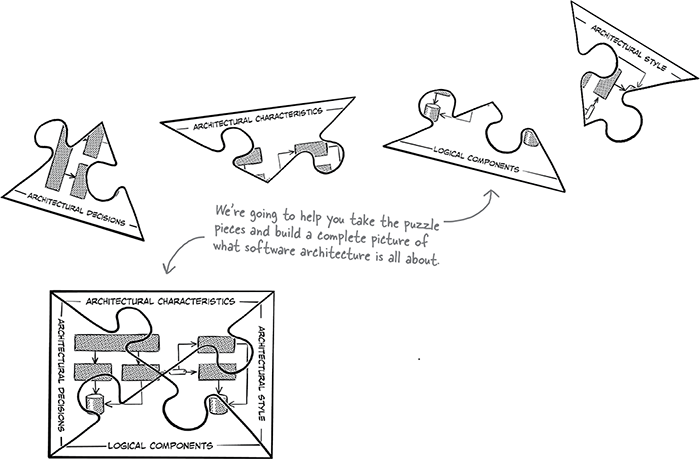
Everything is interconnected.
Did you notice how the pieces of this puzzle are joined in the middle? That’s exactly how software architecture works: each dimension must align.
The architectural style must align with the architectural characteristics you choose as well as the architectural decisions you make. Similarly, the logical components you define must align with the characteristics and the architectural style as well as the decisions you make.
The first dimension: Architectural characteristics
Architectural characteristics form the foundation of the architecture in a software system. Without them, you cannot make architectural decisions or analyze important trade-offs.
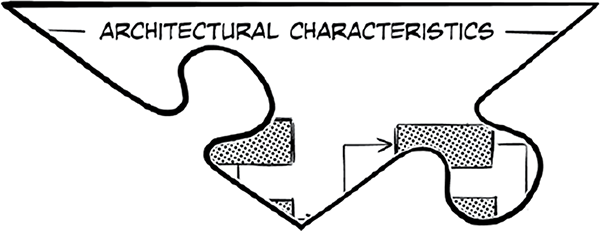
Imagine you’re trying to choose between two homes. One home is roomy but is next to a busy, noisy motorway. The other home is in a nice, quiet neighborhood, but is much smaller. Which characteristic is more important to you—home size or the level of noise and traffic? Without knowing that, you can’t make the right choice.
The same is true with software architecture. Let’s say you need to decide what kind of database to use for your new system. Should it be a relational database, a simple key/value database, or a complex graph database? The answer will be based on what architectural characteristics are critical to you. For example, you might choose a graph database if you need high-speed search capability (we’ll call that performance), whereas a traditional relational database might be better if you need to preserve data relationships (we’ll call that data integrity).
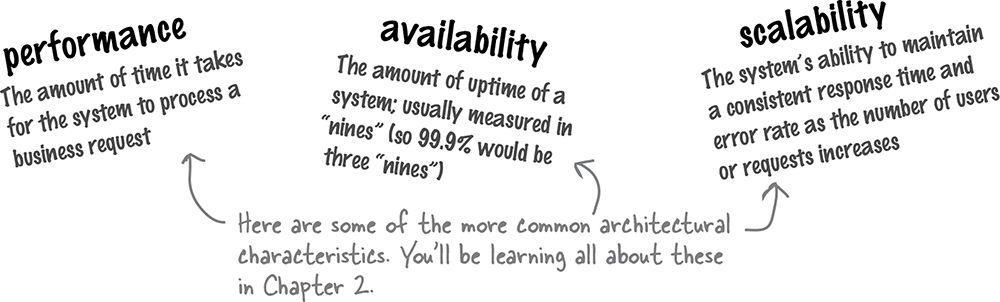
The term architectural characteristics might not be familiar to you, but that doesn’t mean you haven’t heard of them before. Collectively, things like performance, scalability, reliability, and availability are also known as nonfunctional requirements, system quality attributes, and simply “the -ilities” because most end with the suffix -ility. We like the term architectural characteristics because these qualities help define the character of the architecture and what it needs to support.
Note
Architectural characteristics are capabilities that are critical or important to the success of the system.
The second dimension: Architectural decisions
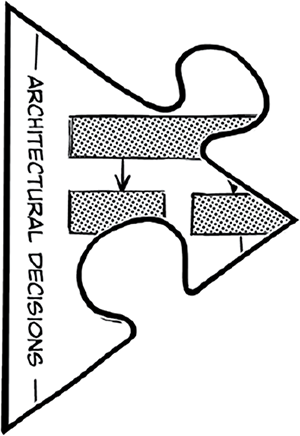
Architectural decisions are choices you make about structural aspects of the system that have long-term or significant implications. As constraints, they’ll guide your development team in planning and building the system.
Should your new home have one floor or two? Should the roof be flat or peaked? Should you build a big, sprawling ranch house? These are good examples of architectural decisions because they involve the structural aspect of your home.
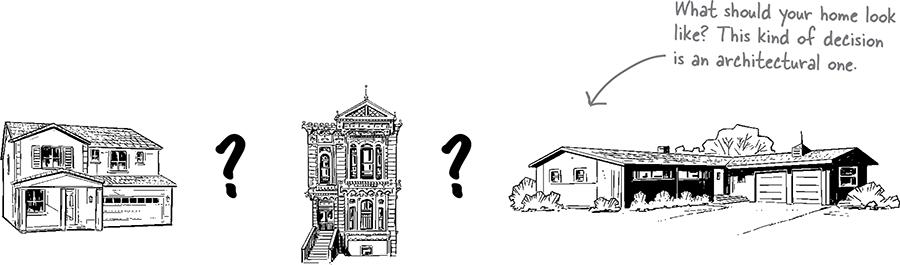
You might decide that your system’s user interface should not communicate directly with the database, but instead must go through the underlying services to retrieve and update data. This architectural decision places a particular constraint on the development of the user interface, and also guides the development team about how other components should access and update data in the database.
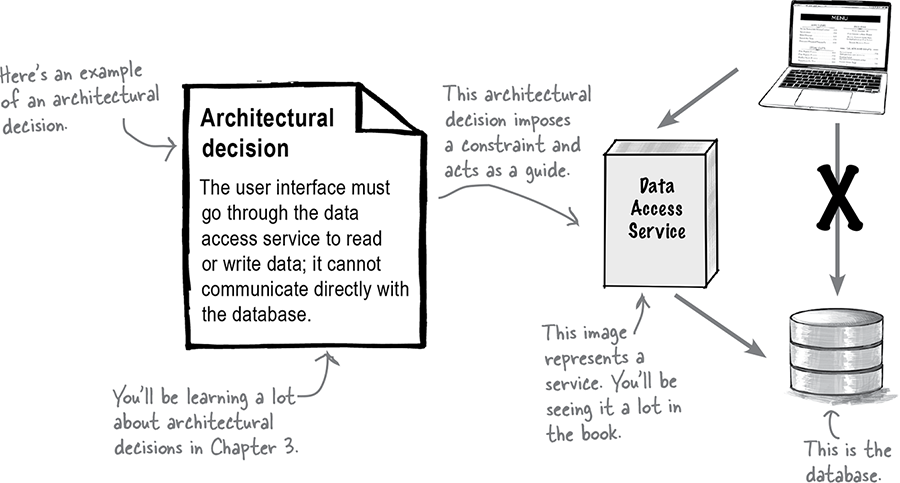
It’s not uncommon to have several dozen or more documented architectural decisions within any system. Generally, the larger and more complicated the system, the more architectural decisions it will have.
The third dimension: Logical components
Logical components are the building blocks of a system, much in the same way rooms are the building blocks of your home. A logical component performs some sort of function, such as processing the payment for an order, managing item inventory, or tracking orders.
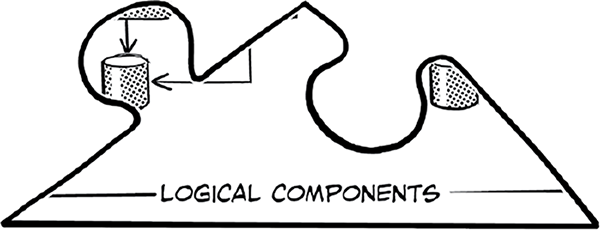
Logical components in a system are usually represented through a directory or namespace. For example, the directory app/order/payment
with the corresponding namespace app.order.payment
identifies a logical component named Payment Processing. The source code that allows users to pay for an order is stored in this directory and uses this namespace.
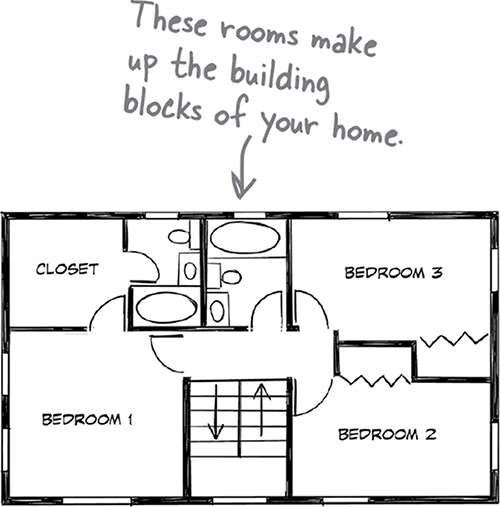
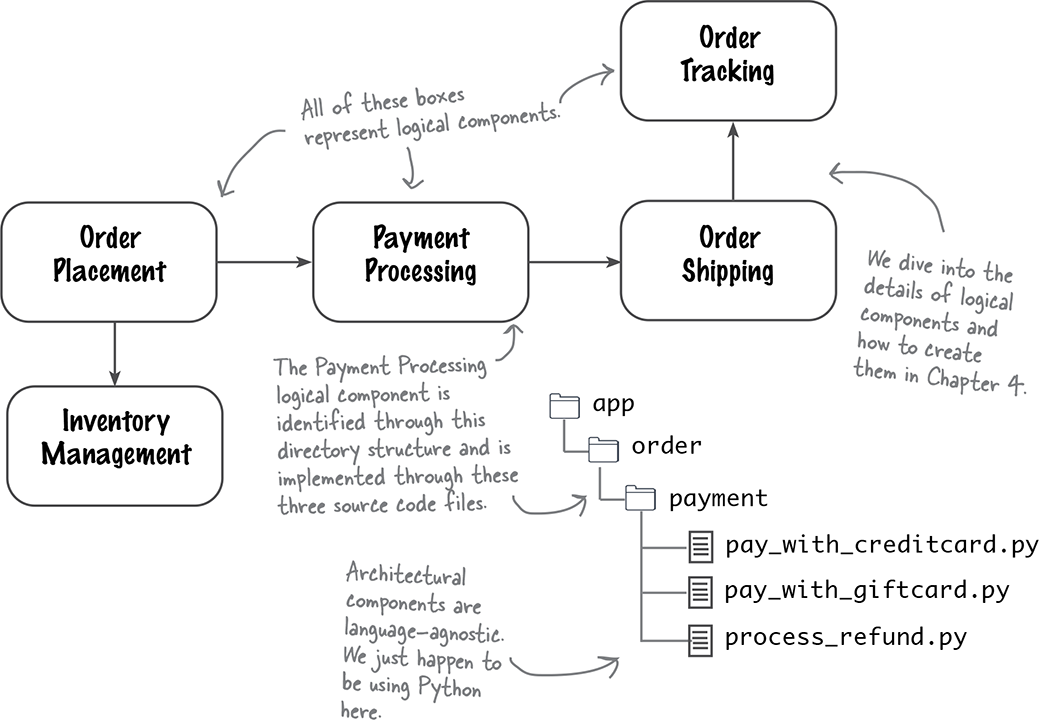
A logical component should always have a well-defined role and responsibility in the system—in other words, a clear definition of what it does.
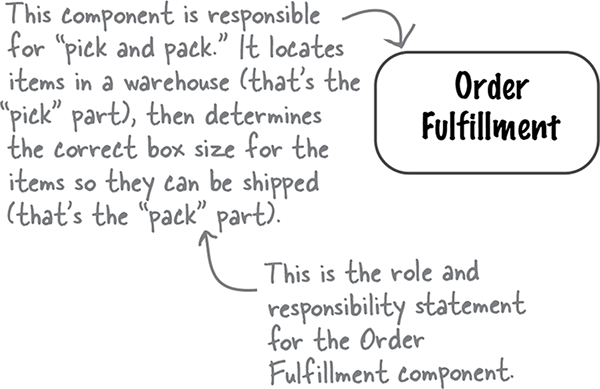
The fourth dimension: Architectural styles
Homes come in all shapes, sizes, and styles. While there are some wild-looking houses out there, most conform to a particular style, such as Victorian, ranch, or Tudor. The style of a home says a lot about its overall structure. For example, ranch homes typically have only one floor; colonial and Tudor homes typically have chimneys; contemporary homes typically have flat roofs.
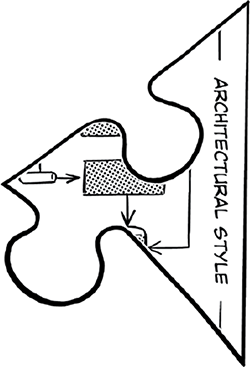
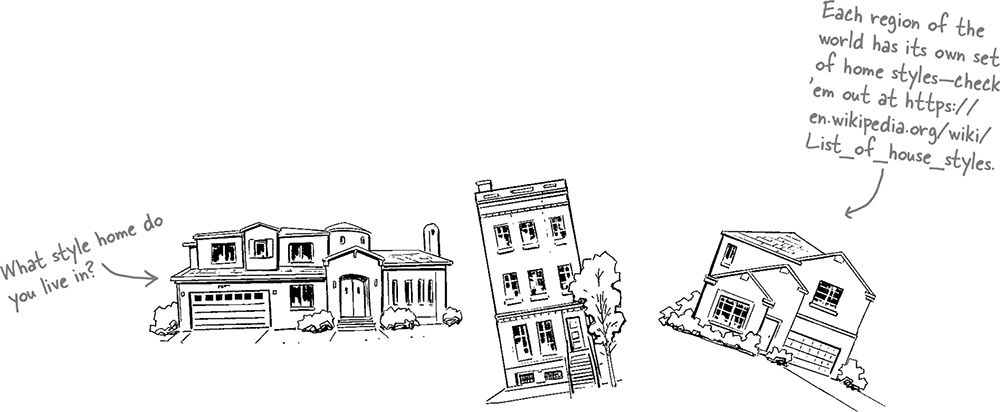
Architectural styles define the overall shape and structure of a software system, each with its own unique set of characteristics. For example, the microservices architectural style scales very well and provides a high level of agility—the ability to respond quickly to change—whereas the layered architectural style is less complex and less costly. The event-driven architectural style provides high levels of scalability and is very fast and responsive.
Note
Don’t worry—you’ll be learning all about these architectural styles later in the book. We’ve devoted chapters to each of them.
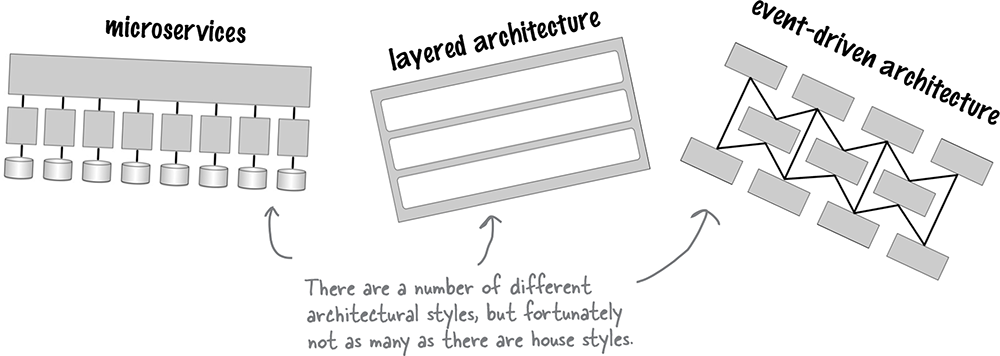
Because the architectural style defines the overall shape and characteristics of the system, it’s important to get it right the first time. Why? Can you imagine starting construction on a one-story ranch home, and in the middle of construction changing your mind and deciding you’re going to build a three-story Victorian house instead? That would be a major undertaking, and likely exceed your budget and affect when you can move into the house.
Software architecture is no different. It’s not easy changing from a monolithic layered architecture to microservices. Like the house example, this would be quite an undertaking.
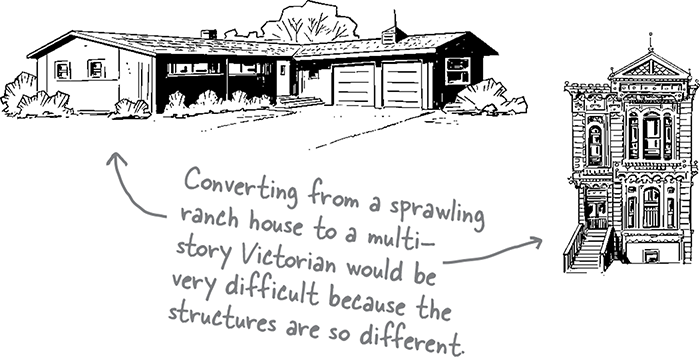
Later in the book, we’ll show you how to properly select an architectural style based on characteristics that are important to you.
Which brings us back to an earlier point—all of the dimensions of software architecture are interconnected. You can’t select an architectural style without knowing what’s important to you.
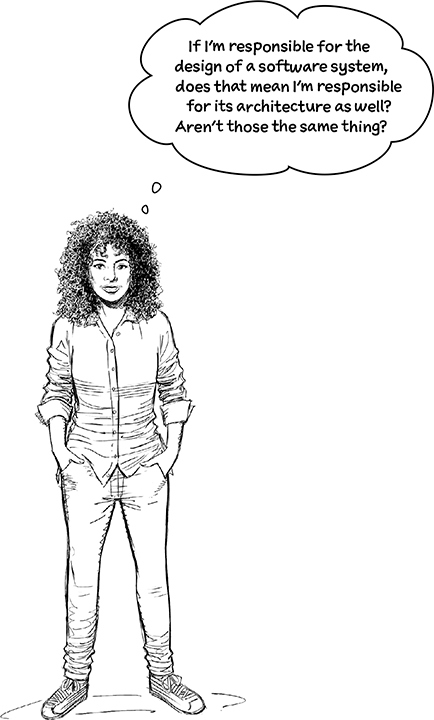
No, architecture and design are different.
You see, architecture is less about appearance and more about structure, while design is less about structure and more about appearance.
The color of a room’s walls, the placement of furniture, and the type of flooring (carpet or wood) are all aspects of design, whereas the physical size of the room and the placement of doors and windows are part of architecture—in other words, the structure of the room.
Think about a typical business application. The architecture, or structure, is all about how the web pages communicate with backend services and databases to retrieve and save data, whereas the design is all about what each page looks like: the colors, the placement of the fields, which design patterns you use, and so on. Again, it becomes a matter of structure versus appearance.
Your question is a good one, because sometimes it gets confusing trying to tell what is considered architecture and what is considered design. Let’s investigate these differences.
A design perspective
Suppose your company wants to replace its outdated order processing system with a new custom-built one that better suits its specific needs. Customers can place orders and can view or cancel orders once they have been placed. They can pay for an order using a credit card, a gift card, or both payment methods.
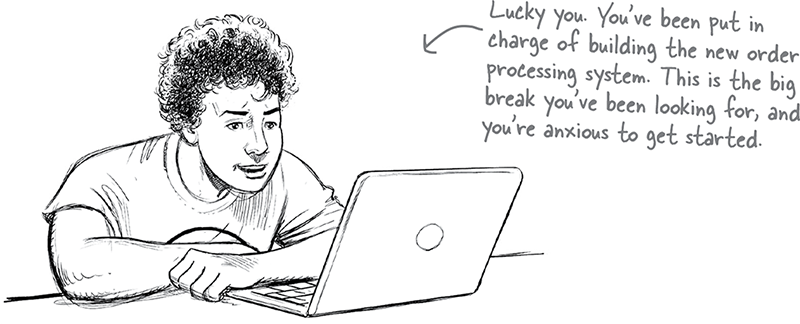
From a design perspective, you might build a Unified Modeling Language (UML) class diagram like the one below to show how the classes interact with each other to implement the payment functionality. While you could write source code to implement these class files, this design says nothing about the physical structure of the source code—in other words, how these class files would be organized and deployed.
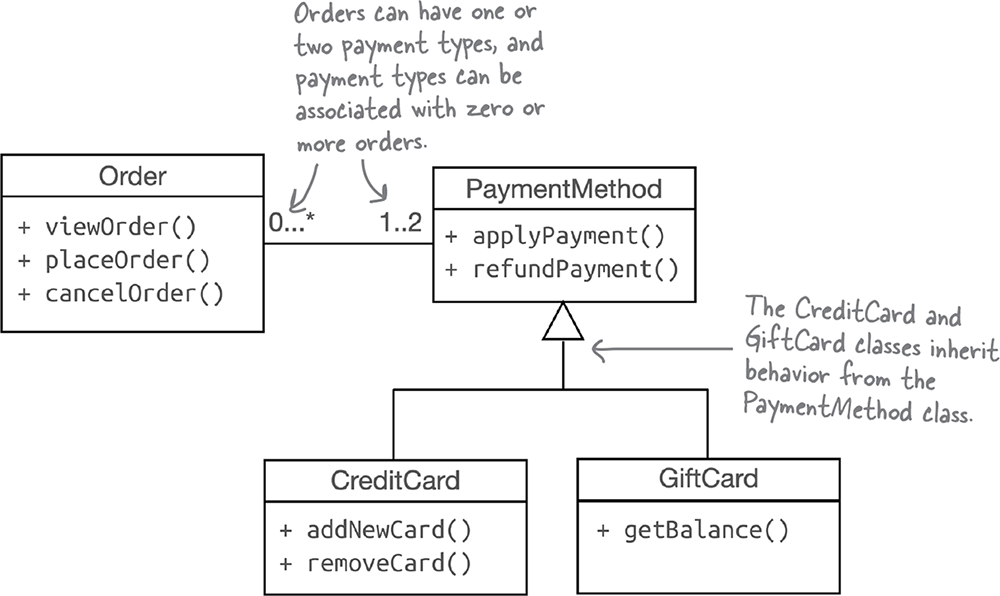
An architectural perspective
Unlike design, architecture is about the structure of the system—things like services, databases, and how services communicate with each other and the user interface.
Let’s think about that new order processing system again. What would the system look like? From an architectural perspective, you might decide to create separate services for each payment type within the order payment process and have an orchestrator service to manage the payment processing part of the system, like in the diagram below.
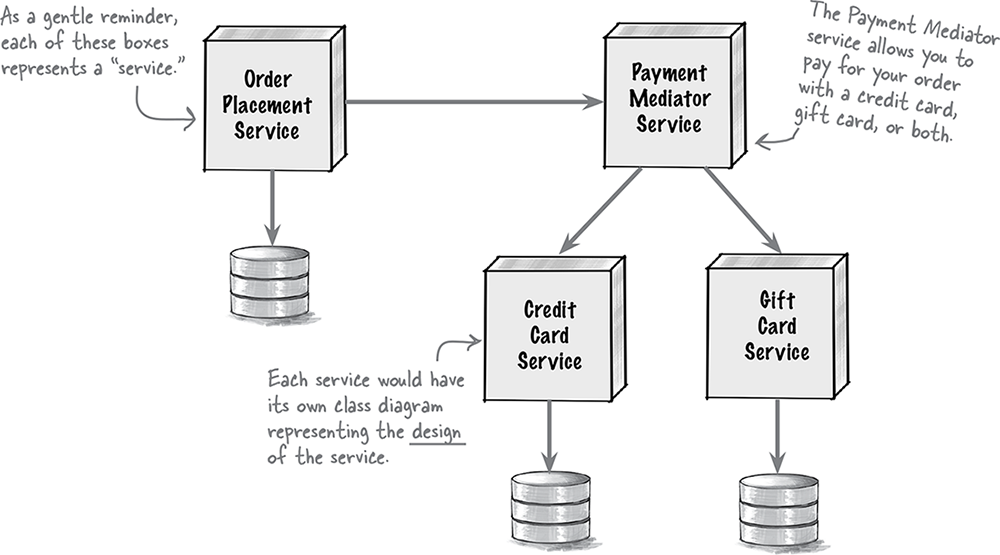
The spectrum between architecture and design
Some decisions are certainly architectural (such as deciding which architectural style to use), and others are clearly design-related (such as changing the position of a field on a screen or changing the type of a field within a class). In reality, most decisions you encounter will fall between these two examples, within a spectrum of architecture and design.
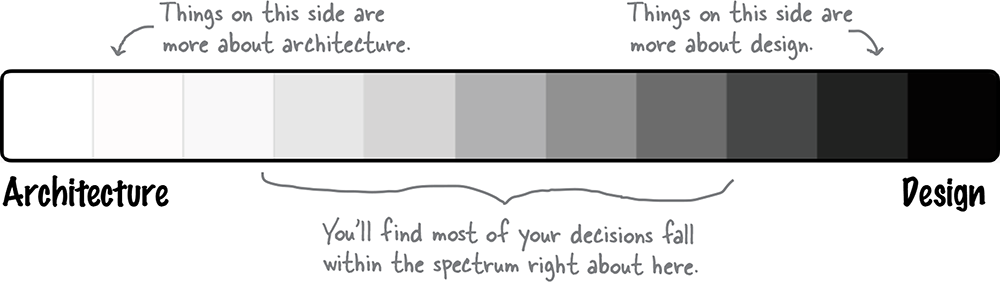
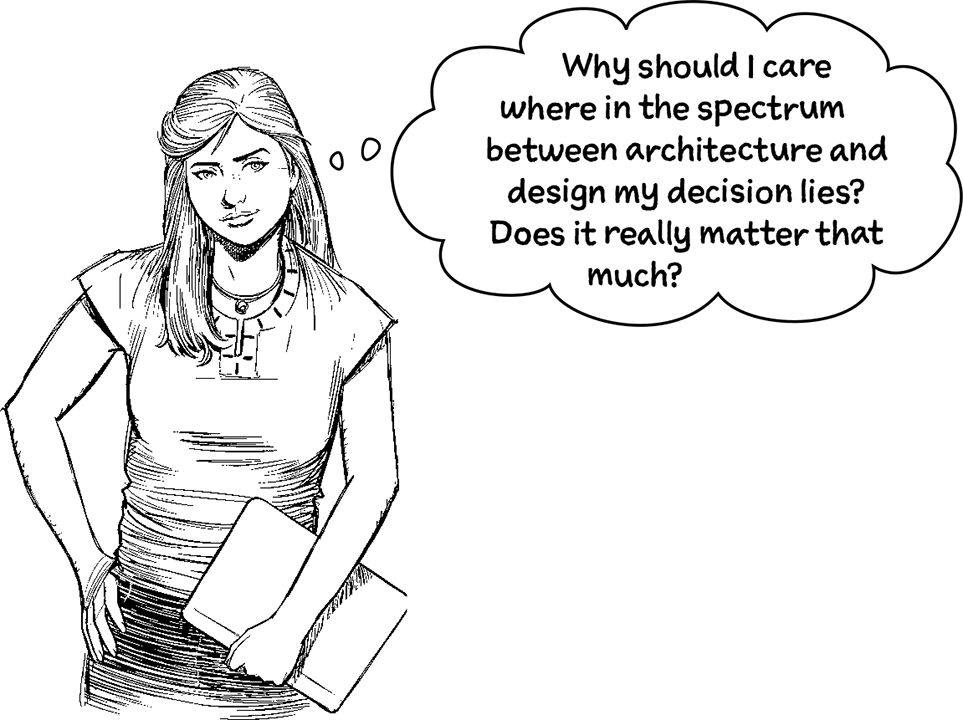
Yes, it matters a lot. You see, knowing where along the spectrum between architecture and design your decision lies helps determine who should be responsible for ultimately making that decision. There are some decisions that the development team should make (such as designing the classes to implement a certain feature), some decisions that an architect should make (such as choosing the most appropriate architectural style for a system), and others that should be made together (such as breaking apart services or putting them back together).
Where along the spectrum does your decision fall?
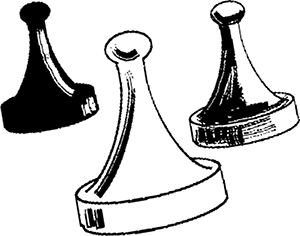
Is it strategic or tactical?
Strategic decisions are long term and influence future actions or decisions. Tactical decisions are short term and generally stand independent of other actions or decisions (but may be made in the context of a particular strategy). For example, deciding how big your new home will be influences the number of rooms and the sizes of those rooms, whereas deciding on a particular lighting fixture won’t affect decisions about the size of your dining room table. The more strategic the decision, the more it sits toward the architecture side of the spectrum.
How much effort will it take to construct or change?
Architectural decisions require more effort to construct or change, while design decisions require relatively less. For example, building an addition to your home generally requires a high level of effort and would therefore be more on the architecture side of the spectrum, whereas adding an area rug to a room requires much less effort and would therefore be more on the design side.
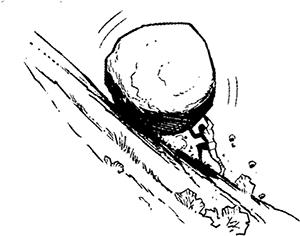
Note
Sometimes waking up in the morning requires a lot of effort—we’ll call those “architecture” mornings.
Does it have significant trade-offs?
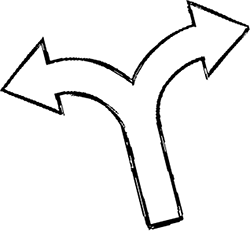
Trade-offs are the pros and cons you evaluate as you are making a decision. Decisions that involve significant trade-offs require much more time and analysis to make and tend to be more architectural in nature. Decisions that have less-significant trade-offs can be made quicker, with less analysis, and therefore tend to be more on the design side.
Note
We’re going to walk you through the details of all three of these factors in the next several pages.
Strategic versus tactical
The more strategic a decision is, the more architectural it becomes. This is an important distinction, because decisions that are strategic require more thought and planning and are generally long term.

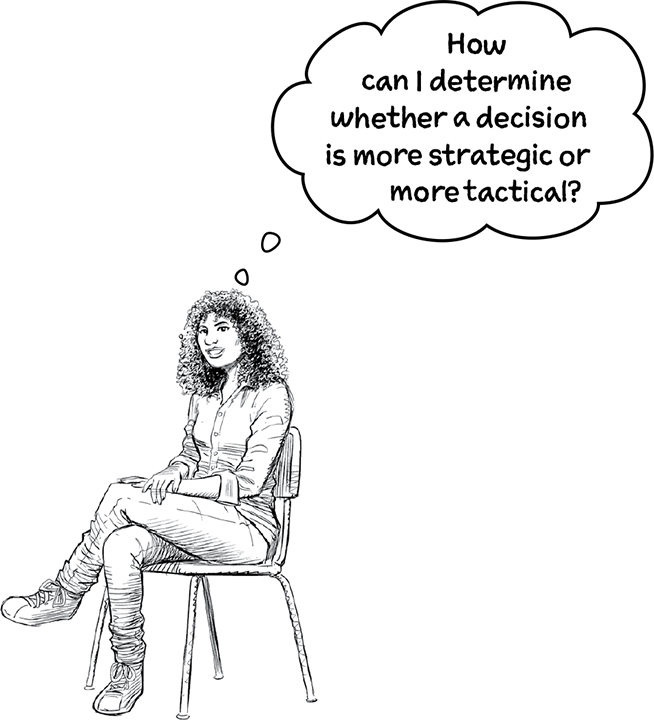
Good question. You can use these three questions to help determine if something is more strategic or tactical. Remember, the more strategic something is, the more it’s about architecture.
How much thought and planning do you need to put into the decision?
If making the decision takes a couple of minutes to an hour, it’s more tactical in nature. If thought and planning require several days or weeks, it’s likely more strategic (hence more architectural).
How many people are involved in the decision?
The more people involved, the more strategic the decision. A decision you can make by yourself or with a colleague is likely to be tactical. A decision that requires many meetings with lots of stakeholders is probably more strategic.
Does your decision involve a long-term vision or a short-term action?
If you are making a quick decision about something that is temporary or likely to change soon, it’s more tactical and hence more about design. Conversely, if this is a decision you’ll be living with for a very long time, it’s more strategic and more about architecture.
High versus low levels of effort
Renowned software architect and author Martin Fowler once wrote that “software architecture is the stuff that’s hard to change.” You can use Martin’s definition to help determine where along the spectrum your decision lies. The harder something is to change later, the further it falls toward the architecture side of the spectrum. Conversely, the easier it is to change later, the more it’s probably related to design.
Note
Martin Fowler’s website (https://martinfowler.com/architecture) has lots of useful stuff about architecture.

Suppose you are planning on moving from one architectural style to another; say, from a traditional n-tiered layered architecture to microservices. This migration effort is rather difficult and will take a lot of time. Because the level of effort is high, this would be on the far end of the architecture side of the spectrum.
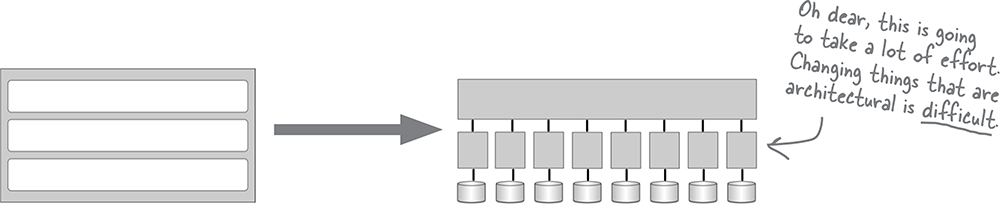
Now suppose you’re rearranging fields on a user interface screen. This task takes relatively less effort, so it resides on the far end of the design side of the spectrum.
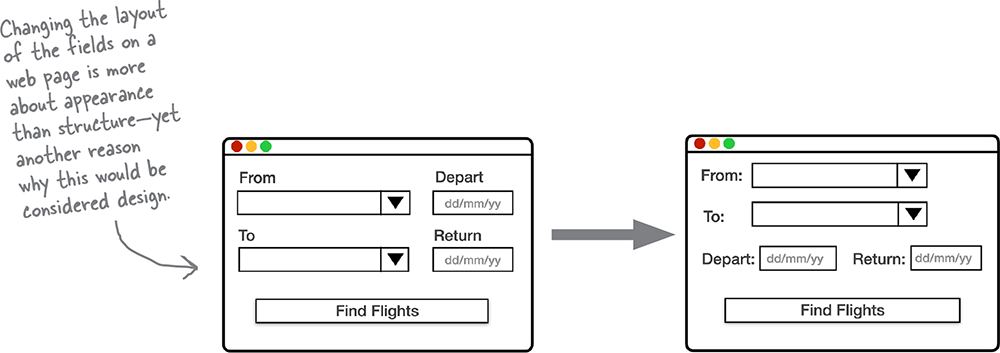
Code Magnets
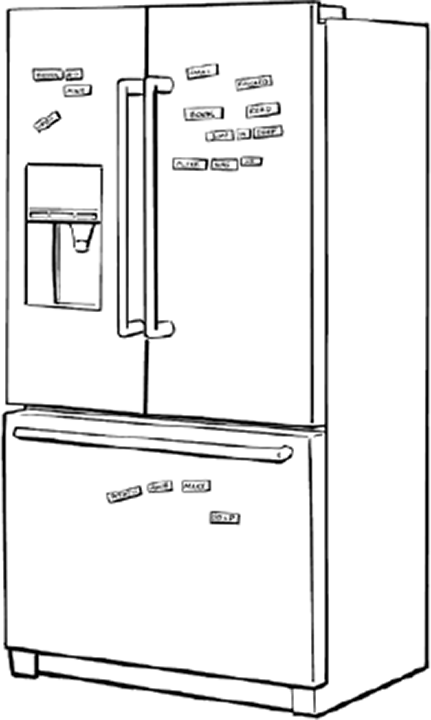
We had all of these magnets from our to-do list arranged from high effort to low effort, and somehow they all fell on the floor and got mixed up. Can you help us put them back in the right order based on the amount of effort it would take to make each change?
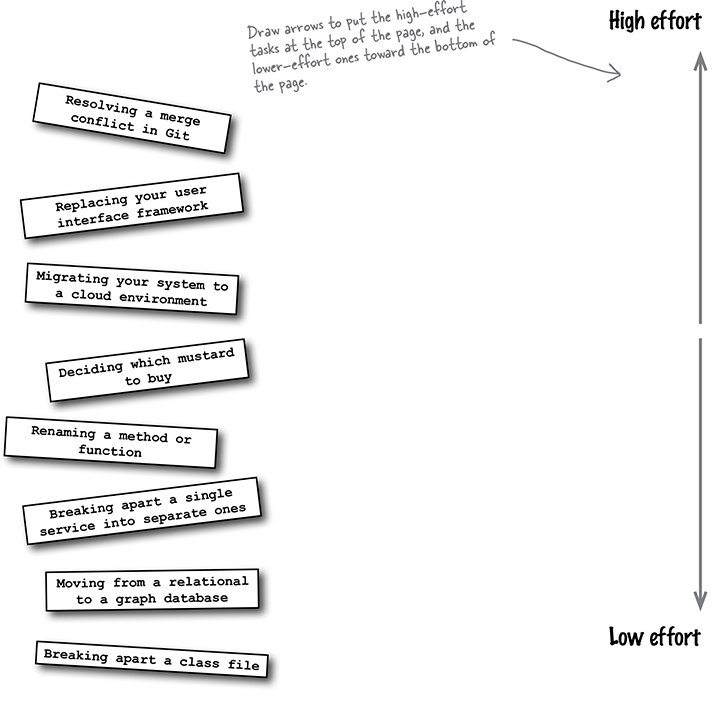
Solution in “Code Magnets Solution”
Significant versus less-significant trade-offs
Some decisions you make might involve significant trade-offs, such as choosing which city to live in. Others might involve less significant trade-offs, like deciding on the color of your living room rug. You can use the level of significance of the trade-offs in a particular decision to help determine whether that decision is more about architecture or design. The more significant the trade-offs, the more it’s about architecture; the less significant the trade-offs, the more it’s about design.

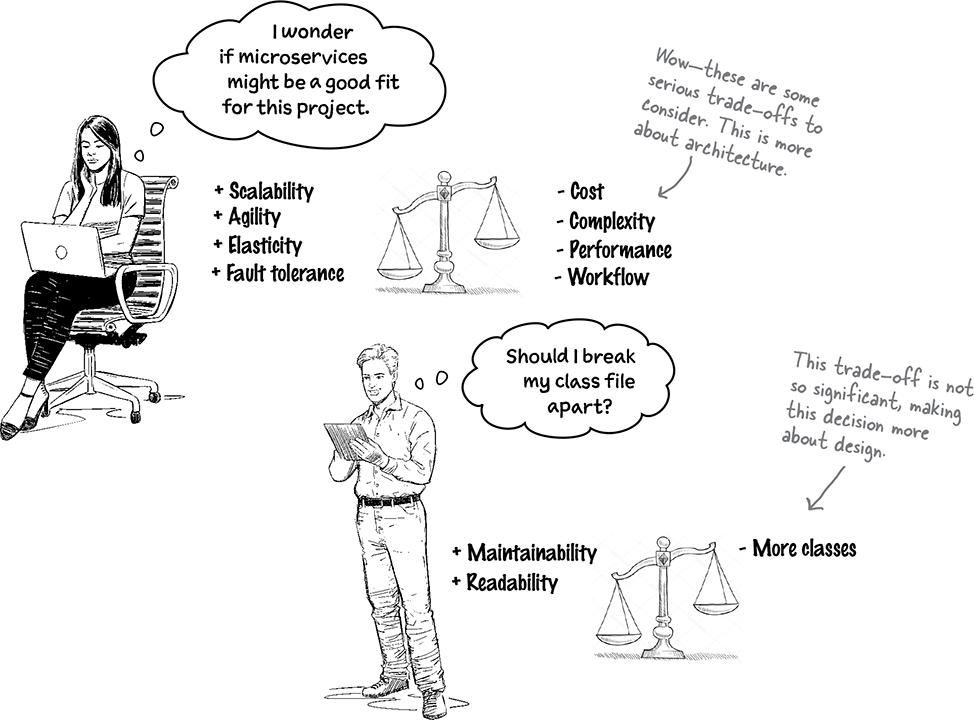
Putting it all together
Now it’s time to put all three of these factors to use to figure out whether a decision is more about architecture or more about design. This tells development teams when to collaborate with an architect and when to make a decision on their own.
Let’s say you decide to use asynchronous messaging between the Order Placement service and the Inventory Management service to increase the system’s responsiveness when customers place orders. After all, why should the customer have to wait for the business to adjust and process inventory? Let’s see if we can determine where in the spectrum this decision lies.
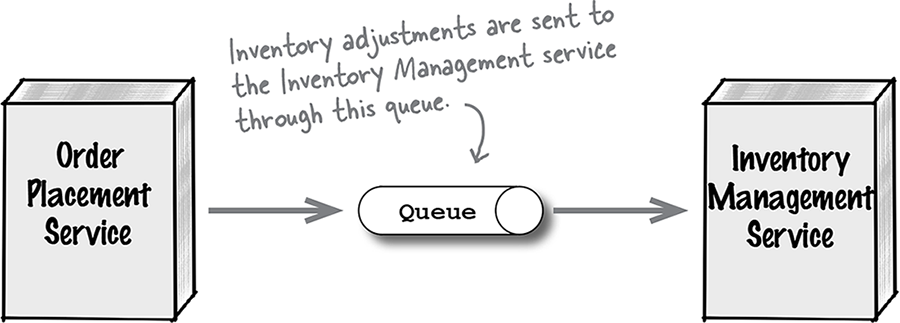
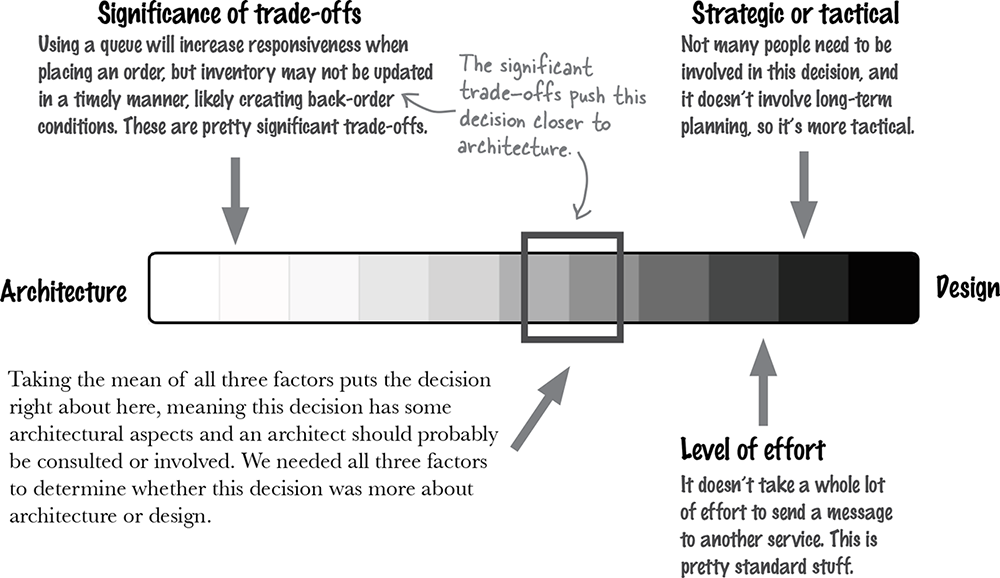
You made it!
Congratulations—you made it through the first part of your journey to understanding software architecture. But before you roll up your sleeves to dig into further chapters, here’s a little quiz for you to test your knowledge so far. For each of the statements below, circle whether it is true or false.
Software Architecture Crossword
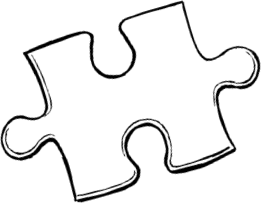
Congratulations! You made it through the first chapter and learned about what software architecture is (and isn’t). Now, why don’t you try architecting the solution to this crossword?
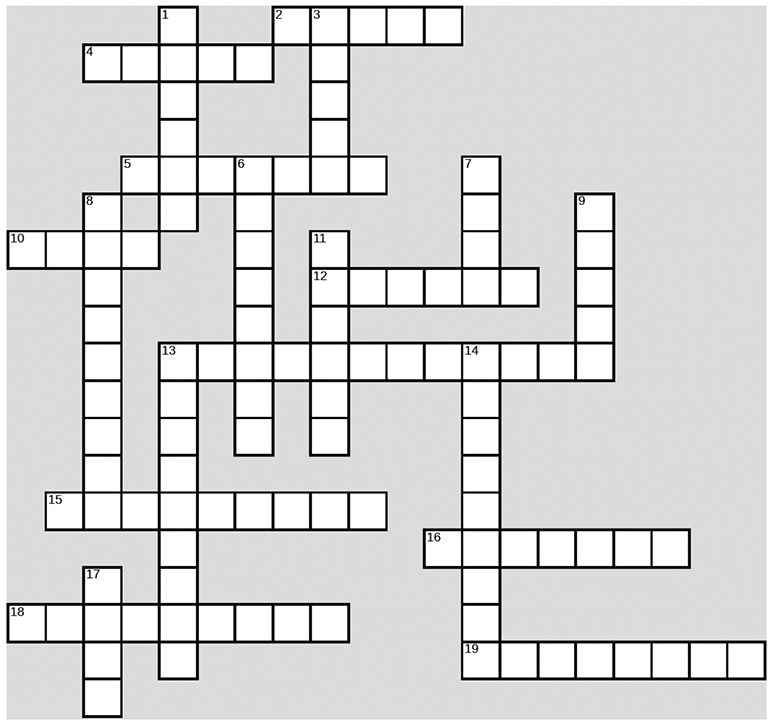
Across
2. An architectural style determings the system’s overall _____
4. _____-driven is an architectural style
5. Architectural characteristics are sometimes called this
10. Architectural decisions are usually _____ term
12. If something takes a lot of _____ to implement, it’s probably architectural
13. You’re learning about software _____
15. You’ll make lots of architectural _____
16. A system’s _____ components are its building blocks
18. The number of rooms in your home is part of its _____
19. Architecture and design exist on a _____
Down
1. Strategic decisions typically involve a lot of these
3. Building this can be a great metaphor
6. Decisions can be strategic of _____
7. How many dimensions it takes to describe a software architecture
8. A website’s user _____ involves lots of design decisions
9. The overall shape of a house or a system, like Victorian or microservices
11. It’s important to know whether a decision is about architecture or this
13. You might want to become one after reading this book
14. You analyze these when making an architectural decision
17. Trade-offs are about the _____ and cons
Solution in “Software Architecture Crossword Solution”
From “Exercise”
From “Exercise”
From “Who Does What?”
From “BE the architect”
From “Who Does What?”
From “Exercise”
From “Code Magnets”
Code Magnets Solution
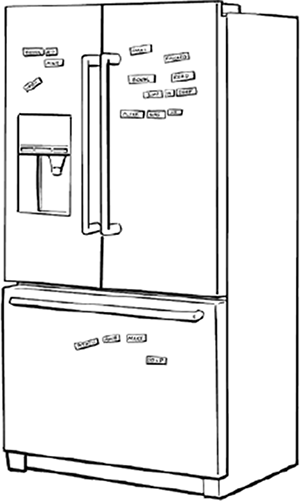
We had all of these magnets from our to-do list arranged from high effort to low effort, and somehow they all fell on the floor and got mixed up. Can you help us put them back in the right order based on the amount of effort it would take to make each change?
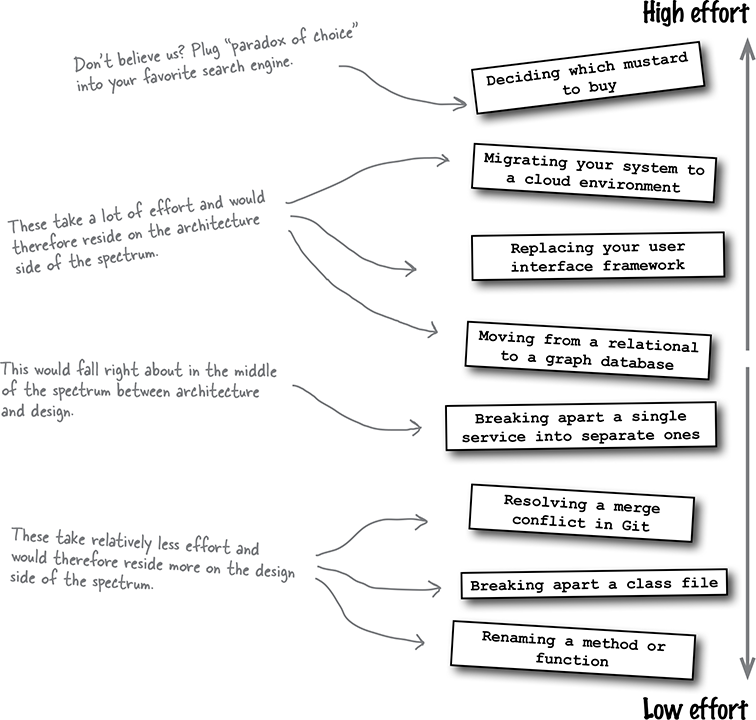
From “Exercise”
From “True or False”
Get Head First Software Architecture now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.