Chapter 1. 1 thinking computationally: Getting Started
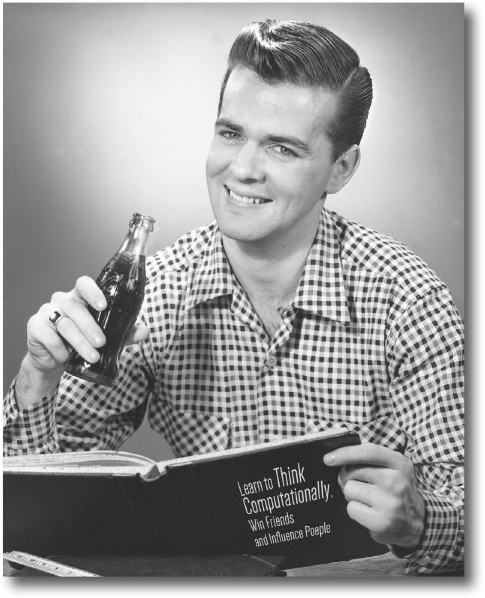
Knowing how to think computationally puts you in control. It’s no secret the world around you is becoming more connected, more configurable, more programmable, and more, well, computational. You can remain a passive participant, or you can learn to code. When you can code, you’re the director, the creator—you’re telling all those computers what they should be doing for you. When you can code, you control your own destiny (or at least you’ll be able to program your internet-connected lawn sprinker system). But how do you learn to code? First, learn to think computationally. Next, you grab a programming language so you can speak the same lingo as your computer, mobile device, or anything with a CPU. What’s in it for you? More time, more power, and more creative possibilities to do the things you really want to do. Come on, let’s get started...
Breaking it down
The first thing that stands between you and writing your first real piece of code is learning the skill of breaking problems down into achievable little actions that a computer can do for you. Of course, you and the computer will also need to be speaking a common language, but we’ll get to that topic in just a bit.
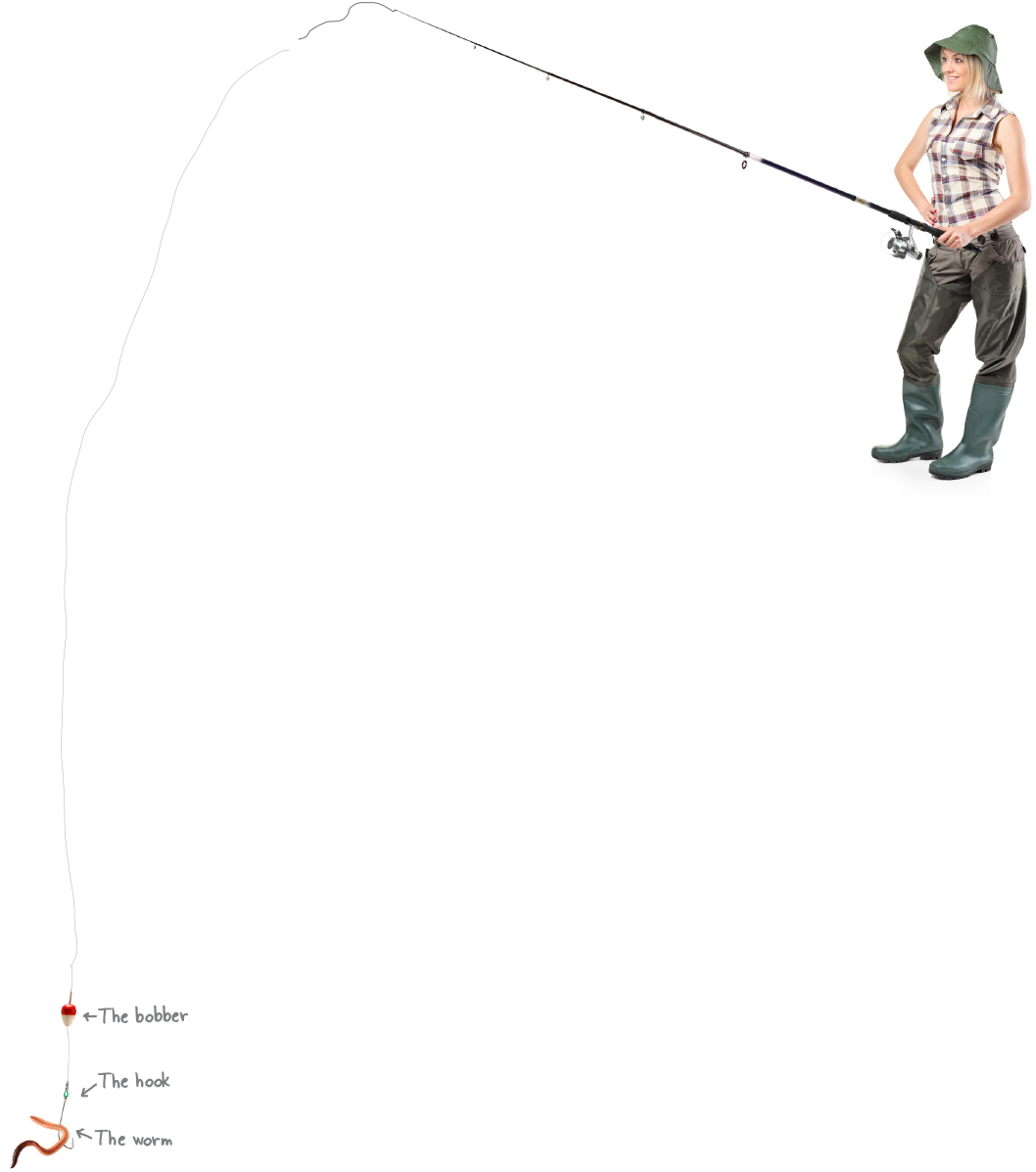
Now breaking problems down into a number of steps may sound like a new skill, but it’s actually something you do every day. Let’s look at a simple example: say you wanted to break the activity of fishing down into a simple set of instructions that you could hand to a robot, who would do your fishing for you. Here’s our first attempt to do that:
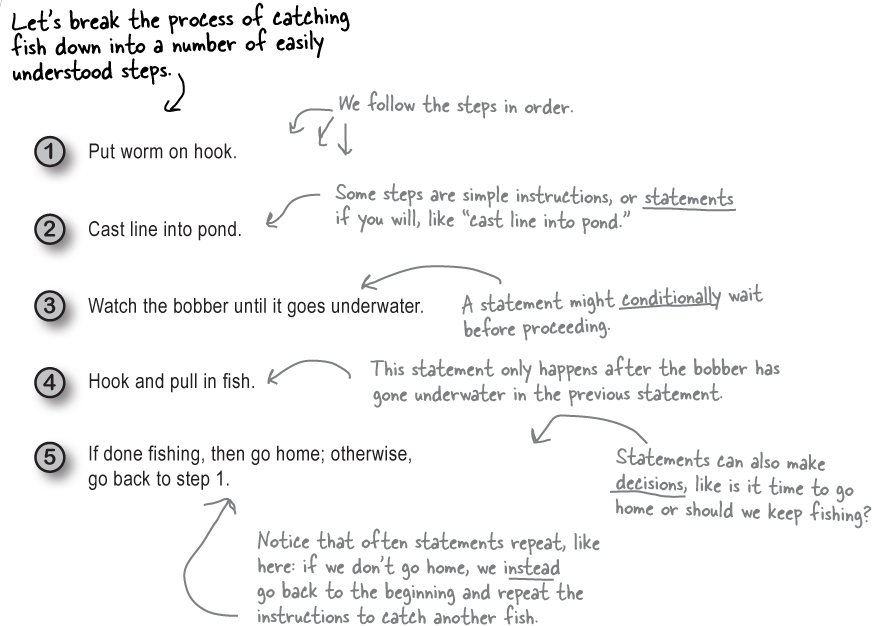
You can think of these statements as a nice recipe for fishing. Like any recipe, this one provides a set of steps that, when followed in order, will produce some result or outcome (in our case, hopefully, catching some fish).
Notice that most steps consist of a simple instruction, like “cast line into pond,” or “pull in the fish.” But also notice that other instructions are a bit different because they depend on a condition, like “is the bobber above or below water?” Instructions might also direct the flow of the recipe, like “if you haven’t finished fishing, then cycle back to the beginning and put another worm on the hook.” Or, how about a condition for stopping, as in “if you’re done, then go home”?
You’re going to find that these simple statements or instructions are the foundation of coding. In fact, every app or software program you’ve ever used has been nothing more than a (sometimes large) set of simple instructions to the computer that tell it what to do.
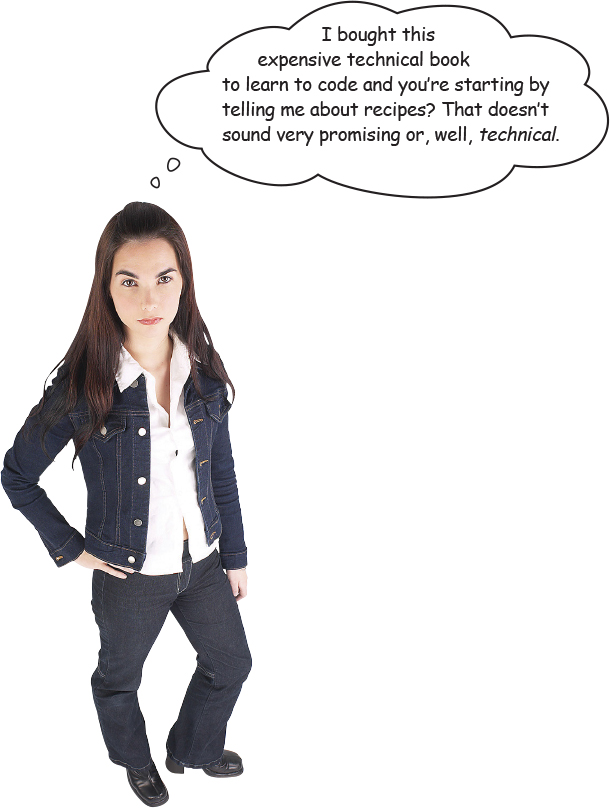
Actually, a recipe is a perfectly good way to describe a set of instructions to a computer. You might even run into that term loosely used here and there in more advanced programming books. Heck, you’ll even find books on common software development techniques that are called cookbooks. That said, if you want to get technical we can—a computer scientist or serious software developer would commonly call a recipe an algorithm. What’s an algorithm? Well, not much more than a recipe—it’s a sequence of instructions that solves some problem. Often you’ll find algorithms are first written in an informal form of code called pseudocode.
Note
More on pseudocode in a bit...
One thing to keep in mind is that, whether you’re talking about a recipe, pseudocode, or an algorithm, the whole point is to work out a high-level description of how to solve a problem before you get into the nitty-gritty details of writing code that the computer can understand and execute.
Note
As you’ll see, this can make the task of coding more straightforward and less error-prone.
In this book, you’ll hear us interchange all these terms, where appropriate—and, oh, in your next job interview you might want to use the term algorithm or even pseudocode to ensure that larger signing bonus (but there’s still nothing wrong with the word recipe).
Note
Just as there are many recipes for the same dish, with algorithms, you’ll find there are many ways to solve the same problem. Some more tasty than others.
How coding works
So you’ve got a task you want the computer to do for you, and you know you’ll need to break that task down into a number of instructions the computer can understand, but how are you going to actually tell the computer to do something? That’s where a programming language comes in—with a programming language you can describe your task in terms that you and the computer both understand. But before we take a deep dive into programming languages, let’s look at the steps you’ll take to actually write code:
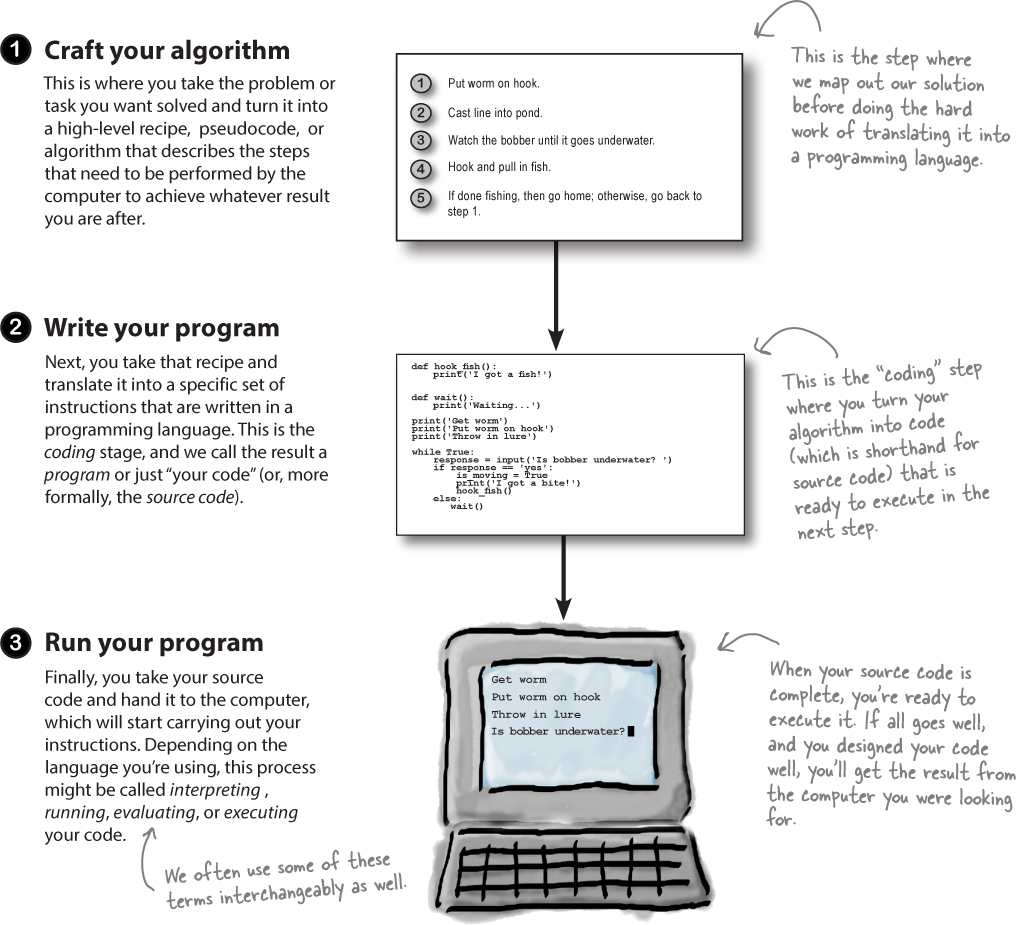
Are we even speaking the same language?
Think of a programming language as a special-purpose language created expressly for specifying tasks to a computer. Programming languages give you a way to describe your recipes in a manner that is clear and precise enough that a computer can understand it.
To learn a programming language there are two things you need to nail down two things: what things can you say using the language, and what do those things mean? A computer scientist would call these the syntax and semantics of the language. Just stash those terms in the back of your brain for now; we’ll be getting you up to speed on both as the book progresses.
Now, as it turns out, just like spoken languages, there are many programming languages, and, as you may already have figured out, in this book we’re going to use the Python programming language. Let’s get a little better feel for languages and Python...
Note
You’ll find that the techniques you’re learning in this book can be applied to just about any programming language you might encounter in the future.
The world of programming languages
If you’re reading this book you may have, in passing, heard about various programming languages. Just walking through the programming section of your local bookstore you might encounter Java, C, C++, LISP, Scheme, Objective-C, Perl, PHP, Swift, Clojure, Haskell, COBOL, Ruby, Fortran, Smalltalk, BASIC, Algol, JavaScript, and of course Python, to name just a few. You might also be wondering where all these names came from. The truth is, programming language names are a lot like the names of rock bands—they’re names that meant something to the people who created the language. Take Java, for instance: it was named, not surprisingly, after coffee (the preferred name Oak was already taken). Haskell was named after a mathematician, and the name C was chosen because C was the successor of the languages A and B at Bell Labs. But why are there so many languages and what are they all about? Let’s see what a few folks have to say about the languages they use:
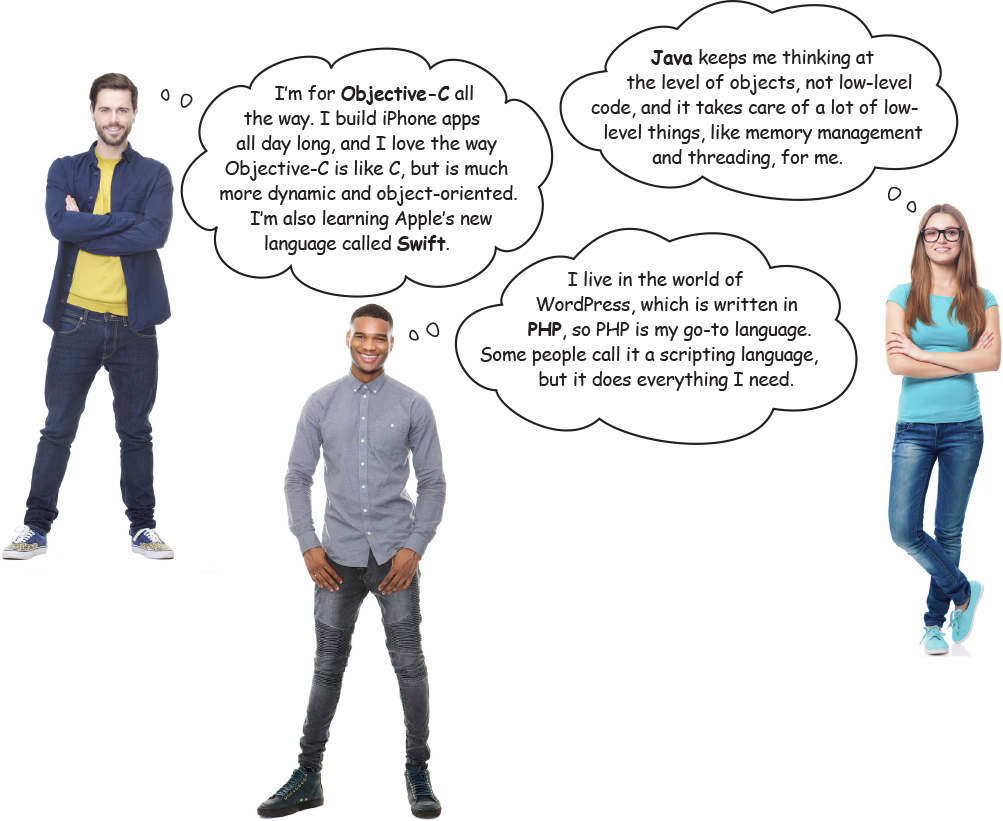
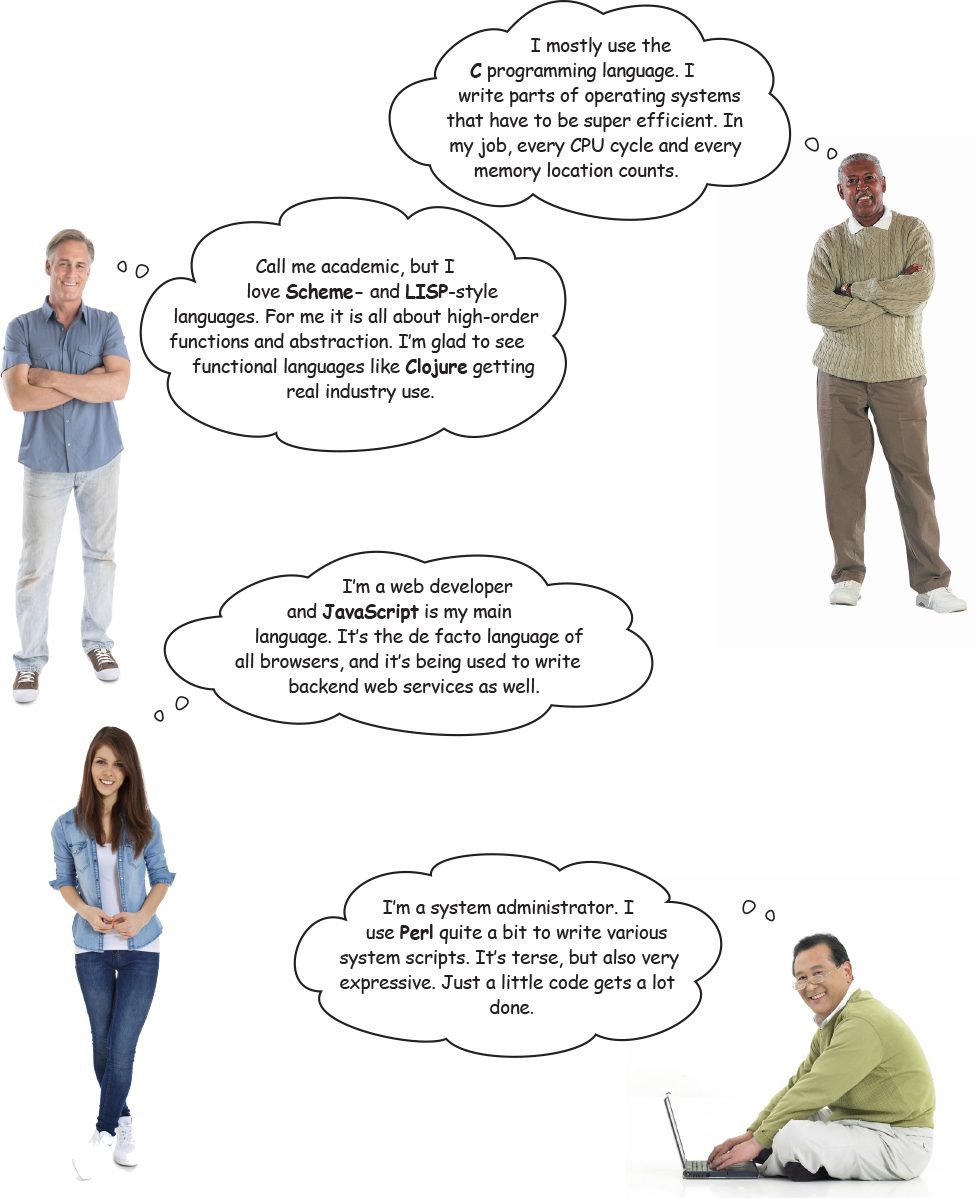
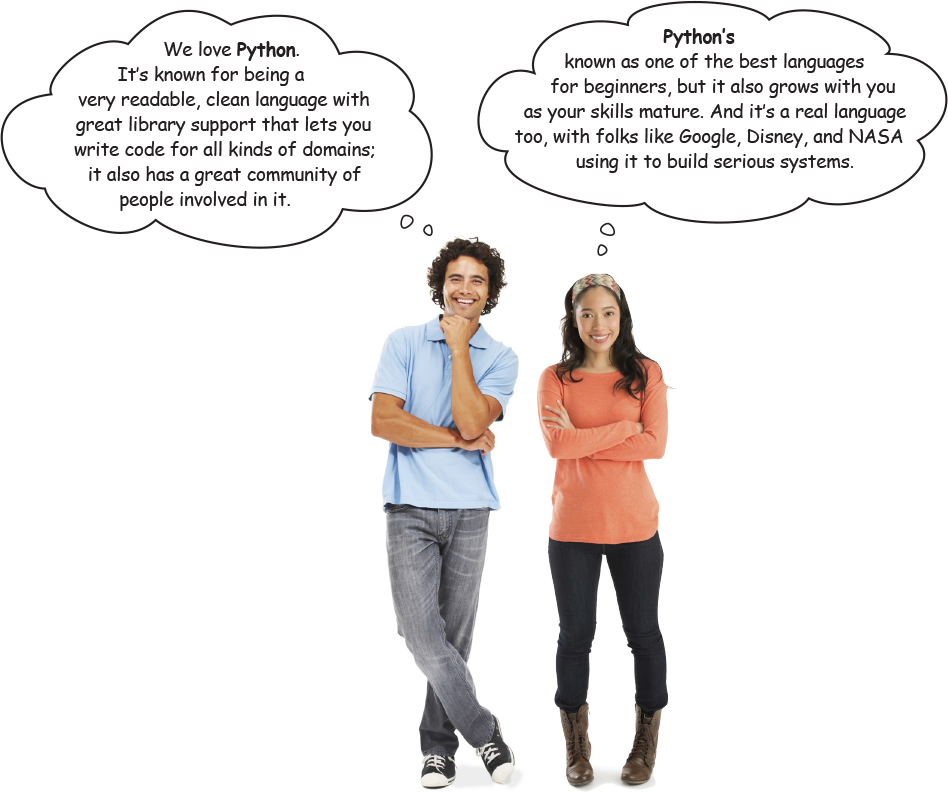
Choices, choices...
As you can see, there are a lot of languages and opinions out there, and we’ve barely scratched the surface of modern languages. You can also see there’s a lot of terminology that comes along with these languages, and, as you progress, those terms are going to make a lot more sense to you. For now, just know there’s a wide variety of languages out there, with more being created every day.
So, in this book, what should we use? Here’s the thing: first and foremost, we want to learn how to think computationally—that way, no matter what language you run across in the future, you’ll be in a good position to learn it. But, that said, we have to start with some language, and, as you already know, we’re going to use Python. Why? Our friends above said it well: it’s considered one of the best languages for beginners because it’s such a readable and consistent language. It’s also a powerful language in that no matter what you want to do with it (now or beyond this book), you can find support in terms of code extensions (we call them modules or libraries) and a supportive community of developers to give you a hand. Finally, some developers will even tell you Python is just more fun than other languages. So how can we go wrong?
How you’ll write and run code with Python
Now that we’ve talked about and even looked at some code, it’s time to start thinking about how you’d actually write and execute some real code. As we mentioned, depending on the language and environment, there are a lot of different models for how you do that. Let’s get a sense for how you’re going to be writing and running your Python code:
Writing your code
First you get your code typed into an editor and saved. You can use any text editor, like Notepad on Windows or TextEdit on the Mac, to write your Python code. That said, most developers use specialized editors known as IDEs (or Integrated Development Environments) to write their code. Why? IDEs are a bit like word processors—they give you lots of nice features like autocompletion of common Python keywords, highlighting of the language syntax (or of errors), as well as built-in testing facilities. Python also conveniently includes an IDE called IDLE, which we’ll be looking at shortly.
Running your code
Running your code is as easy as handing it to the Python interpreter, a program that takes care of everything needed to execute the code you’ve written. We’ll step through the details of this in a bit, but you can access the intepreter through IDLE, or directly from your computer’s command line.
How your code is interpreted
We’ve been describing Python as a language that you and the computer both understand. And, as we’ve learned, an interpreter does the job of reading your code and executing it. To do this, the interpreter actually translates your code behind the scenes into a lower-level machine code that can be directly executed by your computer hardware. You won’t need to worry about how it does this; just know that the interpreter will do the job of executing each statement of your Python code.
there are no Dumb Questions
Q: Why not use English to program computers? Then we wouldn’t need to learn these special-purpose programming languages.
A: Yes, wouldn’t that be nice. As it turns out, English is full of ambiguity, which makes creating such a translator extremely difficult. Reseachers have made small inroads in this area, but we’re a long way from using English, or any other spoken language as a programming language. Also, in the few languages, that have tried to be more English-like, we’ve found that programmers prefer languages that are less like a spoken language and that are more streamlined for coding.
Q: Why isn’t there just one programming language?
A: Technically all modern programming languages are equivalent in that they can all compute the same things, so, in theory, we could use one programming language to serve all our needs. Like spoken languages, however, programming languages differ in their expressive power—that is, you’ll find some types of programming tasks (say, building websites) are easier in some languages than others. Other times the choice of a programming language just comes down to taste, using a particular methodology, or even the need to use the language that your employer has adopted. Count on one thing, though: there will be even more languages, as programming languages continue to evolve.
Q: Is Python just a toy language for beginners? If I wanted to be a software developer, is learning Python going to help me?
A: Python is a serious language and used in many products you probably know and love. In addition, Python’s one of the few professional languages that is also considered an excellent language for beginners. Why? Compared to many existing languages, Python approaches things in a straightforward and consistent way (something you’ll have a better understanding of over time, and as you gain experience with Python and other languages).
Q: What’s the difference between learning to code and thinking computationally? Is the latter just a computer science thing?
A: Computational thinking is a way of thinking about problem solving that grew out of computer science. With computational thinking we learn how to break problems down, to create algorithms to solve them, and to generalize those solutions so we can solve even bigger problems. Often, though, we want to teach a computer to execute those algorithms for us, and that’s where coding comes in. Coding is the means by which we specify an algorithm to a computer (or any computational device, like your smartphone). So the two really go hand in hand—computational thinking gives us a way to create solutions to problems that we want to code, and coding provides a means of specifying our solutions to a computer. That said, computational thinking can be valuable even if you aren’t coding.
A very brief history of Python
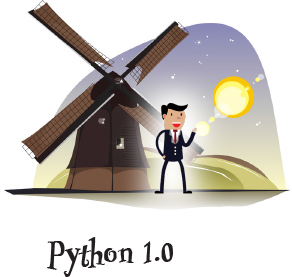
Over in the Netherlands, at the National Research Institute for Mathematics and Computer Science, they had a big problem: their scientists found programming languages difficult to learn. Yes, even to these highly educated, skilled scientists, the most current programming languages were confusing and inconsistent. To the rescue, the Institute developed a new language called “ABC” (you thought we were going to say “Python,” didn’t you?), which was designed to be much easier to learn. While ABC was somewhat successful, an enterprising young developer named Guido van Rossum, after a weekend of binge-watching Monty Python reruns, thought he could take things further—so, using what he’d learned from ABC, Guido created Python. And the rest is history.
Note
Actually, we just made up the part about the binge weekend.
Note
Note from editor: umm, “the rest” is actually in the next few paragraphs.
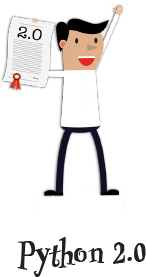
Python came of age with version 2.0 and a whole new set of features aimed at supporting its growing community of developers. For instance, in recognition that Python was truly now a global language, 2.0 was extended to handle character sets from languages far beyond the typical English letters. Python also also improved many technical aspects of the language, like better handling of computer memory as well as better support for common types of data like lists and character strings.
The Python development crew also worked hard to make Python open to a whole community of developers who could help improve the language and implementation.
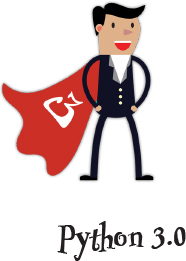
Nobody’s perfect, and there came a time when Python’s creators looked back and saw a few things in Python they wanted to improve. While Python was known for its adherence to keeping things straightforward, experience with the language had revealed a few parts of its design that could be improved, and a few things that hadn’t aged well that needed to be removed.
All these changes meant that some aspects of Python 2 would no longer be supported. That said, Python’s creators made sure there were ways to keep the 2.0 code running. So, if you have code written in Python 2, don’t worry—Python 2 is still alive and well, but know that Python 3 is the future of the language.

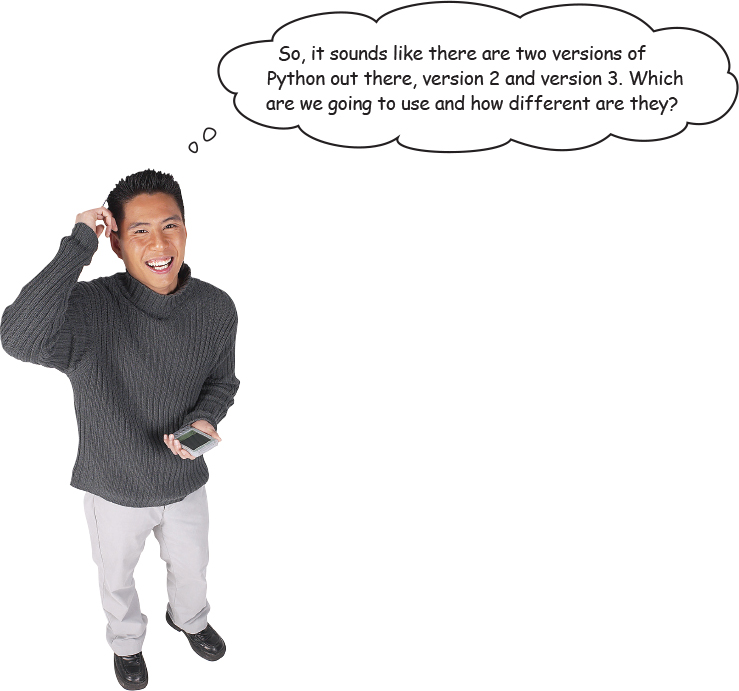
Good question. And you’re right, there are two versions of Python—to be a little more specifc, at the time this book was printed, the current versions are 3.6 and 2.7.
Here’s how we’re going to think about the versions. As it turns out, when you view the two languages, from, say, 10,000 feet, they are remarkably similar, and in fact you might not even be able to see any differences. That said, there are differences, and if you aren’t paying attention to the version you are using, they could trip you up. We’ll be using the newest version of Python in this book—that is, version 3. We’d rather get you started with the version that is going to carry on into the future.
Now there is a lot of code in the world already written in Python 2, and you certainly might encounter it in a module you’ve downloaded online or, if you happen to become a software developer, as part of some old code you become responsible for. After this book you’ll be in a good position to study the small differences between Python 3 and 2, should you need to.
Note
When we say Python 3 or 2, we mean in each case, the latest version of 3 or 2 (at the time of writing, 3.6 and 2.7).
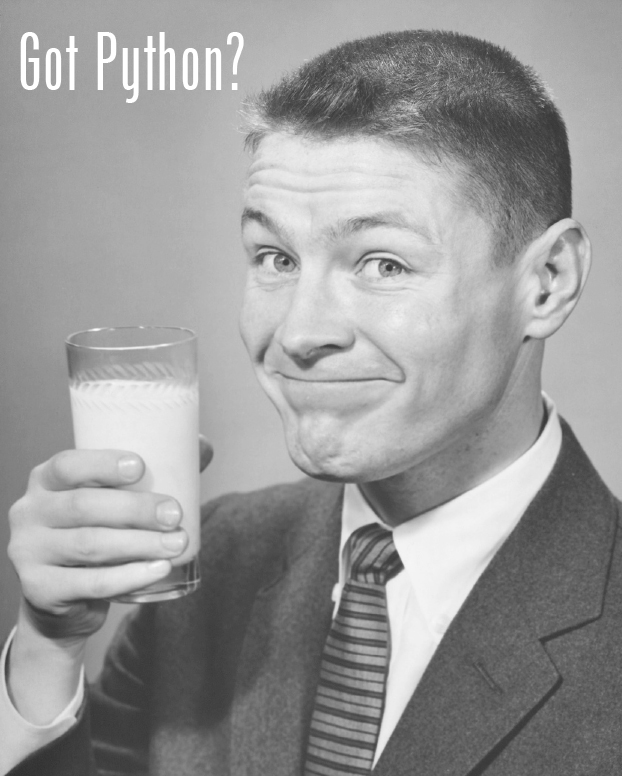
We’re not going to get much further if you haven’t installed Python yet. If you haven’t taken the time to install Python, now is the time. Check out the “You’re going to have to install Python” section in the introduction to get started. Remember, if you’re using a Mac or Linux there’s a good chance you already have Python installed; however, there’s also a good chance you have version 2, not version 3. So whether you’re on a Mac, a Windows, or a Linux machine, at this point you may need to install version 3 of Python.
So, go do that and once you’ve got Python up and running, you’re ready to dive into some real code.
Putting Python through its paces
Now that you’ve got Python installed, let’s put it to work. We’re going to start with a small test program just to make sure everything is up and running. To do that, you need to use an editor to enter your program before you can execute it; that’s where IDLE, the Python editor (or Integrated Development Environment if you prefer), comes in. Go ahead and open IDLE like we did in the introduction. As a reminder, on the Mac you’ll find IDLE under the Applications > Python 3.x
folder. On Windows navigate using the Start button to All Programs, and you’ll find IDLE under the Python 3.x menu option.
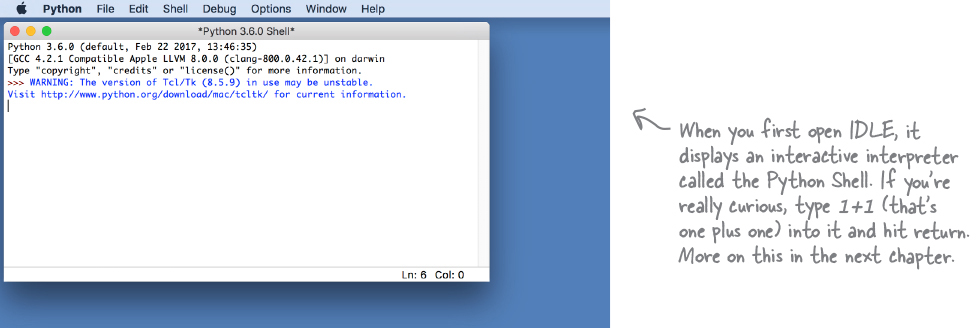
When you first run IDLE it displays an interactive window, called the Python Shell, that you can type Python statements directly into. You can also use an editor to type your code into; to do that, choose File > New File
from the IDLE menu. You’ll see a new, empty editor window appear.
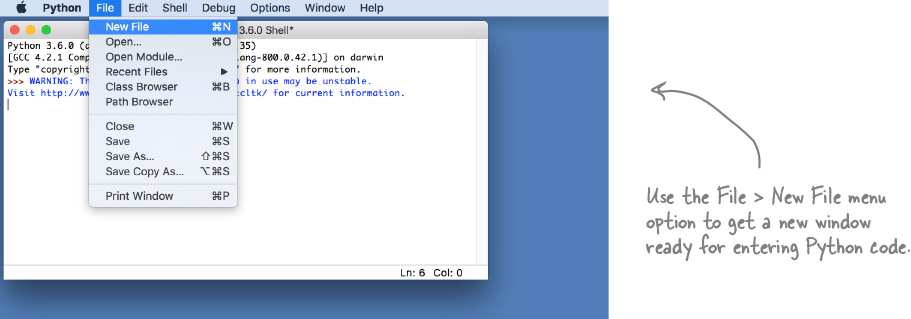
IDLE works just like a word processor, only it knows about Python code and assists you by highlighting Python language keywords, helping you with formatting, and even, when appropriate, autocompleting common Python keywords to make code entry even easier.
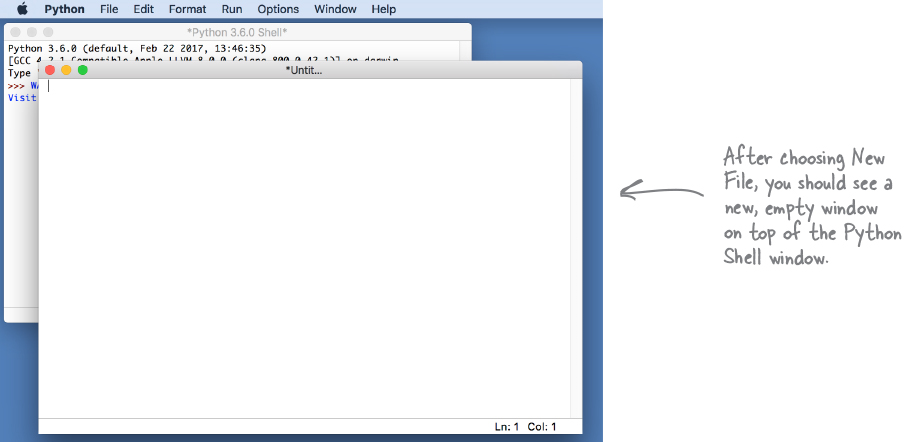
After creating a new file and getting a new blank editing window, you’re going to type in one line of code to test things out; go ahead and enter this code:
print(‘You rock!’)
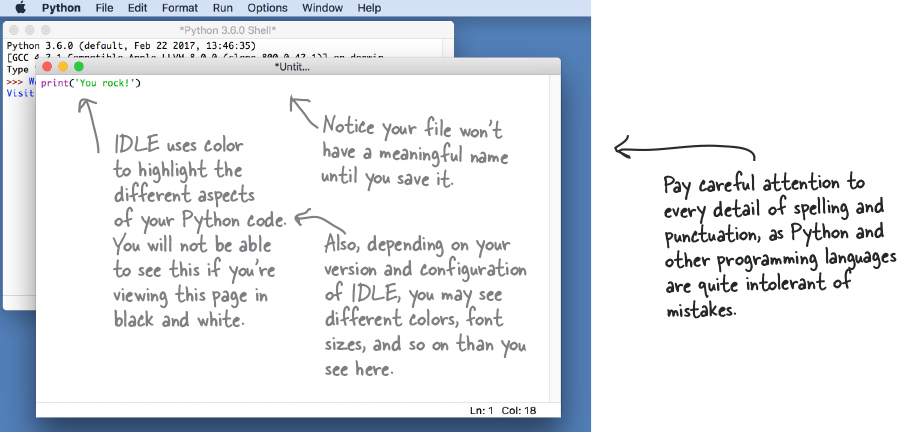
Saving your work
Now that you’ve typed in your first line of code, let’s save it. To do that just choose File > Save
from the IDLE menu:
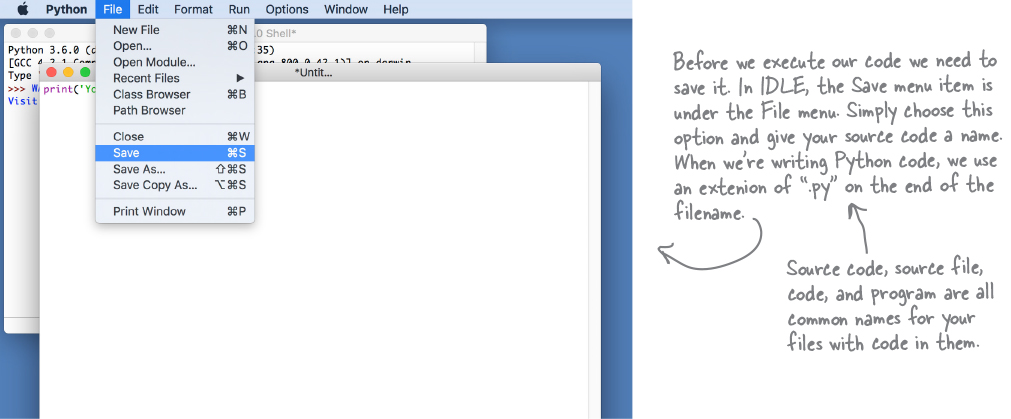
And then give your code a name with a .py extension. We chose the name rock.py. Note that, although we didn’t show it, we also created a folder for Chapter 1 code, called ch1, and we recommend you do too.
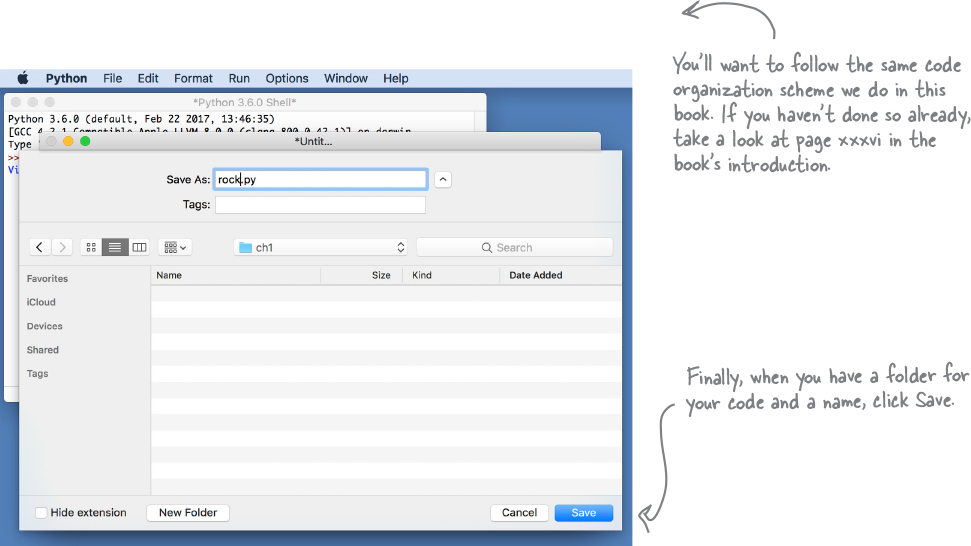
Congrats on coding your first Python program!
You’ve installed Python, you’ve entered a short bit of real Python code using IDLE, and you’ve even executed your first Python program. Now it’s not a very complex bit of code, but you’ve gotta start somewhere. And the good news is, we’re all set up and ready to move on to a serious business application!
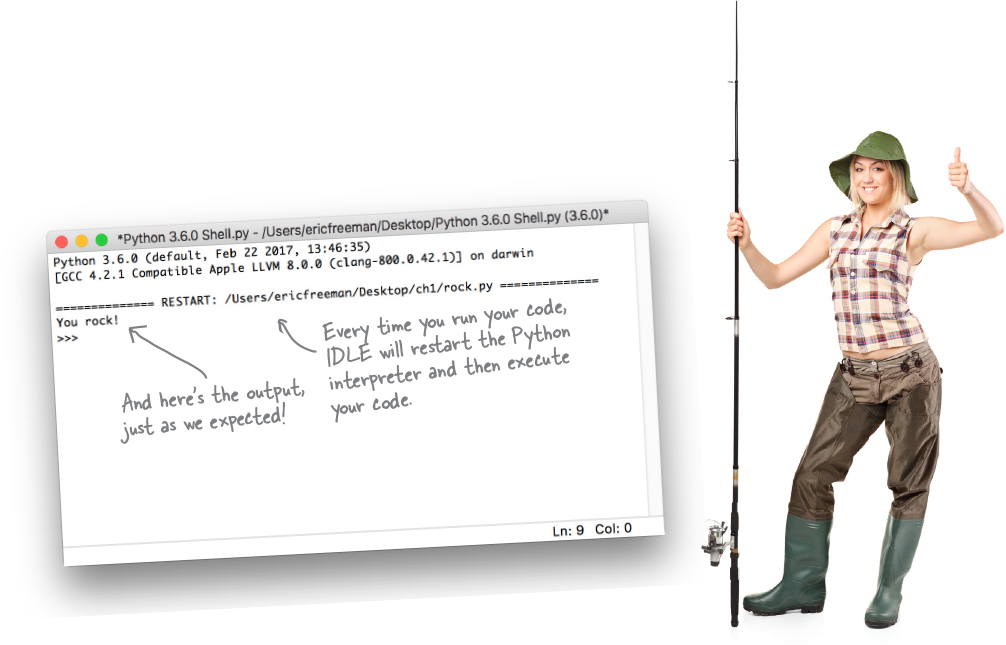
there are no Dumb Questions
Q: Why do we use Run Module to execute our code?
A: Python calls a file of Python code a module. So, all this means is “run all the Python code in my file.” Modules are also a way to further organize code that we’ll get to later.
Q: What exactly do you mean by input and output?
A: Right now we’re just dealing with simple kinds of input and output. Our output is the text that is generated from your program and displayed in the Python Shell window. Likewise, input is any text your program gets from you in the shell window. More generally, all kinds of input and output are possible, such as mouse input, touch input, graphics and sound output, and so on.
Q: I’ve figured out that print gives you a way to print text to the user, but why the name print? When I first saw that, I thought it was something for the printer.
A: Think way, way back to when computers were more likely to output to a printer than a screen. Back in those days, the name print made a lot more sense than it does now. Of course, Python is young enough to not have an excuse for using the name print, but print has traditionally been the way to output to the screen in a lot of languages. So, print outputs to the screen (by way of the Python Shell window), and on a related note, you saw an example earlier of input, which gets user input from the Python Shell.
Q: Is print the only way to output from Python?
A: No, it’s just the most basic way. Computers and programming languages excel at output (and input), and Python is no exception. Using Python (or most languages), you can output to web pages, the network, files on storage devices, graphics devices, audio devices, and many other things.
Q: Okay, well, when I use print, I type something like print(‘hi there’). What exactly is going on there?
A: What you’re doing is using some functionality built into Python to print. More specifically, you’re asking a function called print to take your text in quotes and output it to the Python Shell. Now, we’re going to be getting more into exactly what functions are, what text is, and so on later, but, for now, just know you can use this function anytime you want to print to the shell.
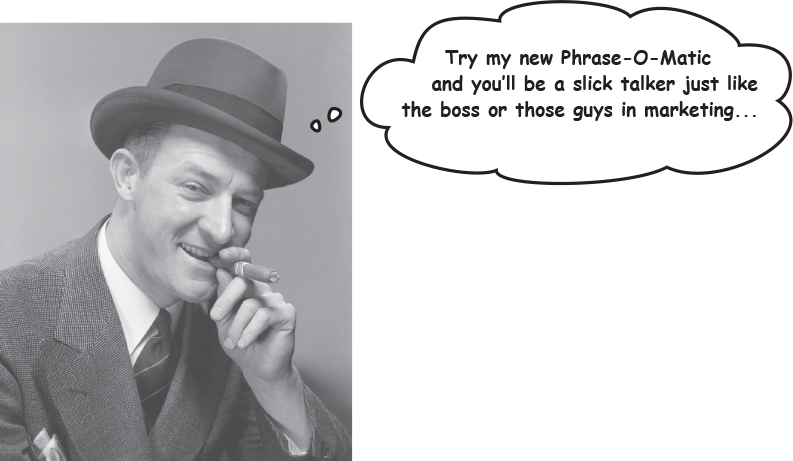
Okay, it’s time to get serious and to write a real-world business application using Python. Check out this Phrase-O-Matic code—you’re going to be impressed.
# let python know we’ll be using some random
# functionality by importing the random module
import random
# make three lists, one of verbs, one of adjectives,
# and one of nouns
verbs = ['Leverage', 'Sync', 'Target', 'Gamify', 'Offline', 'Crowd-sourced', '24/7', 'Lean-in', '30,000 foot'] adjectives = ['A/B Tested', 'Freemium', 'Hyperlocal', 'Siloed', 'B-to-B', 'Oriented', 'Cloud-based', 'API-based'] nouns = ['Early Adopter', 'Low-hanging Fruit', 'Pipeline', 'Splash Page', 'Productivity', 'Process', 'Tipping Point', 'Paradigm']
# choose one verb, adjective, and noun from each list
verb = random.choice(verbs) adjective = random.choice(adjectives) noun = random.choice(nouns)
# now build the phrase by “adding” the words together
phrase = verb + ' ' + adjective + ' ' + noun
# output the phrase
print(phrase)
Phrase-O-Matic
In a nutshell this program takes three lists of words, randomly picks one word from each list, combines the words into a phrase (suitable for your next startup’s slogan), and then prints out the phrase. Don’t worry if you don’t understand every aspect of this program; after all, you’re in “Phrase-O-Matic” of a 600-page book. The point here is just to start to get some familiarity with code:
The
import
statement tells Python we’re going to be using some additional built-in functionality that’s in Python’s random module. Think of this as extending what your code can do—in this case, by adding the ability to randomly choose things. We’ll get into the details of howimport
works later in the book.Next we need to set up three lists. Declaring a list is straightforward—just place each item in the list within quotes and surround it by square brackets, like this:
verbs = ['Leverage', 'Sync', 'Target', 'Gamify', 'Offline', 'Crowd-sourced', '24/7', 'Lean in', '30,000 foot']
Notice we’re assigning each list to a name, like verbs, so we can refer to it later in the code.
Next we need to choose one word randomly from each list. To do that we’re using
random.choice
, which takes a list and randomly chooses one item. We then take that item, and assign it to the corresponding name (verb, adjective, or noun
) so we can refer to it later.Note
random.choice is another built-in function from Python. We’ll learn more about these later in the book.
We then need to create the phrase, and we do this by gluing the three items together (the verb, adjective, and noun)—in Python we can glue these together using the plus sign. Notice also that we have to insert spaces between the words; otherwise, we’d end up with phrases like “Lean-inCloud-basedPipeline.”
Note
Computer scientists like to call gluing text together “concatenation.” It’s a pretty handy word, so you’ll see it more in this book.
Finally, we output the phrase to the Python Shell with the print statement and...voila! We’re in marketing.
Getting the code in the machine
You’ve entered one program into IDLE, but let’s step through it again—we’re going to create a new Python file by choosing File > New File
from the menu. Go ahead and do that, and then type the code from two pages back into the editor.
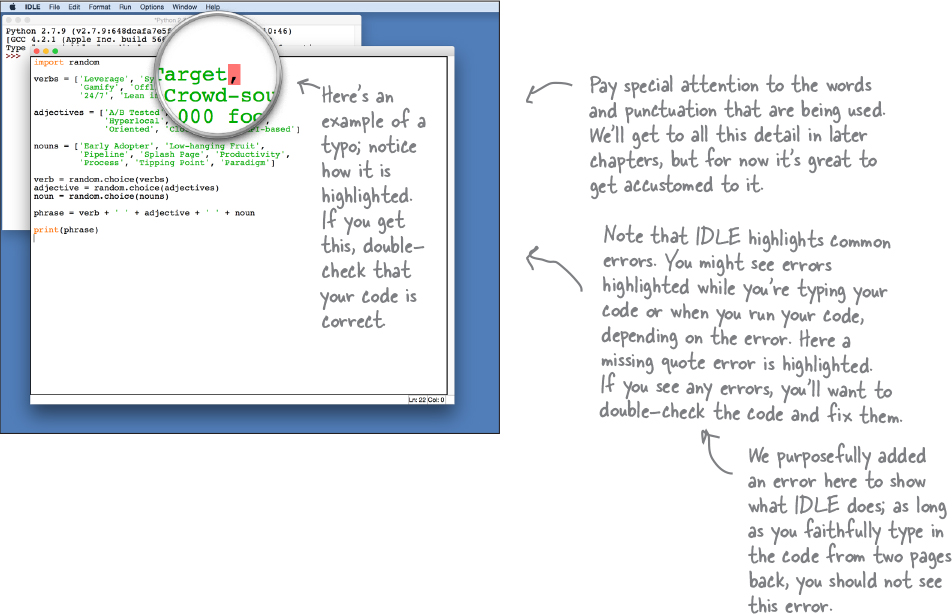
Now that you’ve typed in all the code, let’s save it. Remember, to do that just choose File > Save
from the IDLE menu and then save your code as phraseomatic.py.
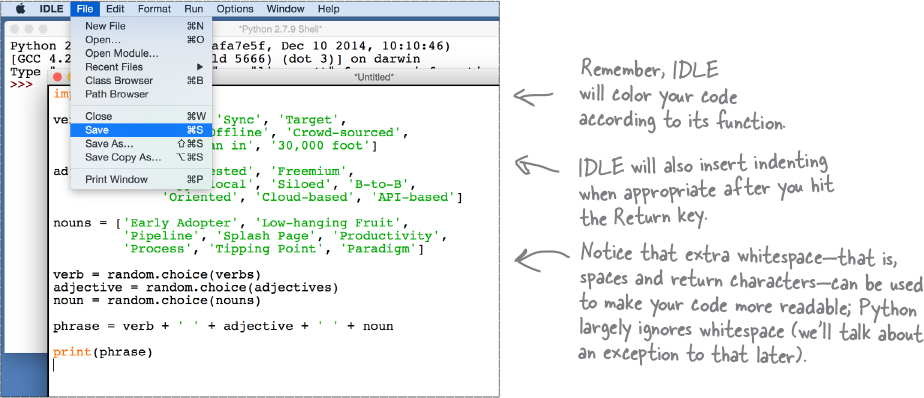
Get Head First Learn to Code now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.