Chapter 8. Enums and Collections Organizing your data
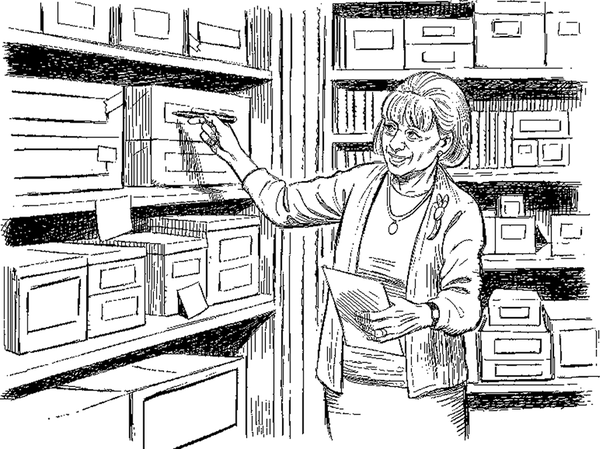
Data isn’t always as neat and tidy as you’d like it to be.
In the real world, you don’t receive your data in tidy little bits and pieces. No, your data’s going to come at you in loads, piles, and bunches. You’ll need some pretty powerful tools to organize all of it—and lucky for us, C# has just the tools we need. Enums are types that let you define valid values to categorize your data. Collections are special objects that store many values, letting you store, sort, and manage all the data that your apps need to pore through. That way, you can spend your time thinking about writing apps to work with your data, and let the collections worry about keeping track of it for you.
If a constructor just sets fields, use a primary constructor instead
Let’s take another look at the FunnyClown class from Chapter 7. It uses a constructor to set a private field and a read-only property that exposes that field to other classes. You added a constructor that takes a string argument and uses it to set a backing field for a read-only property.
class FunnyClown : IClown { public FunnyClown(string funnyThingIHave) { this.funnyThingIHave = funnyThingIHave; } private string funnyThingIHave; public string FunnyThingIHave { get { return funnyThingIHave; } } public void Honk() { Console.WriteLine($"Hi kids! I have {funnyThingIHave}."); } }
Note
Get Head First C#, 5th Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.