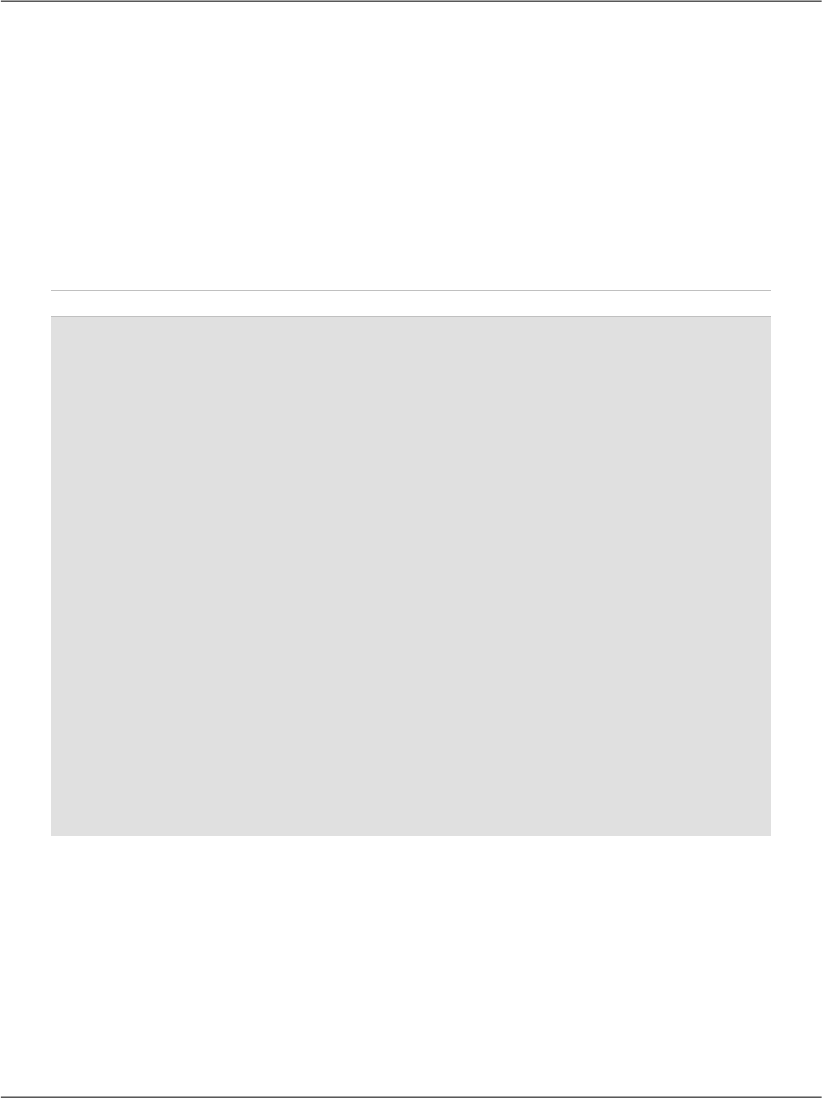
JavaScript or clumsy server-side code to write by hand! Let’s take a look at these
ASP.NET validation controls in detail.
RequiredFieldValidator
The RequiredFieldValidator control is the simplest of the validation controls.
It does exactly what its name suggests: it makes sure that a user enters a value
into a web control. We used the RequiredFieldValidator control in the login
page example presented earlier:
File: Login.aspx (excerpt)
<p>
Username:<br />
<asp:TextBox id="usernameTextBox" runat="server" />
<asp:RequiredFieldValidator id="usernameReq" runat="server"
ControlToValidate="usernameTextBox"
ErrorMessage="Username is required!"
SetFocusOnError="True" Display="Dynamic" />
</p>
<!-- Password -->
<p>
Password and Confirmation:<br />
<asp:TextBox id="passwordTextBox" runat="server"
TextMode="Password" />
<asp:RequiredFieldValidator id="passwordReq" runat="server"
ControlToValidate="passwordTextBox"
ErrorMessage="Password is required!"
SetFocusOnError="True" Display="Dynamic" />
<asp:TextBox id="confirmPasswordTextBox" runat="server"
TextMode="Password" />
<asp:RequiredFieldValidator id="confirmPasswordReq"
runat="server" ControlToValidate="confirmPasswordTextBox"
ErrorMessage="Password confirmation is required!"
SetFocusOnError="True" Display="Dynamic" />
</p>
As you can see, three RequiredFieldValidator controls are used on this page.
The first validates the usernameTextBox control, the second validates the pass-
wordTextBox control, and the third validates the confirmPasswordTextBox
control. These assignments are made using the ControlToValidate property of
the RequiredFieldValidator controls. The ErrorMessage property contains
the error message that will be displayed when the user fails to enter a value into
each control.
230
Chapter 6: Using the Validation Controls