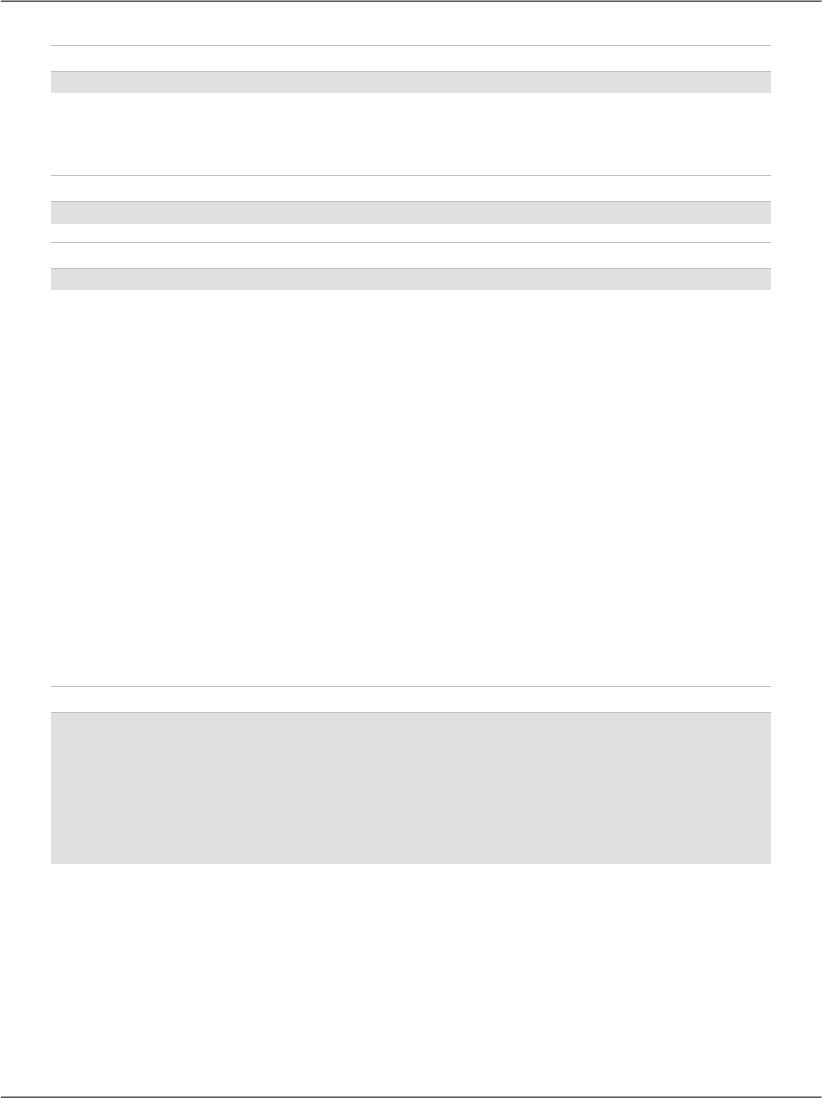
C#
Application.Remove("SiteName");
If you find you have multiple objects and application variables lingering in applic-
ation state, you can remove them all at once using the RemoveAll method:
Visual Basic
Application.RemoveAll()
C#
Application.RemoveAll();
It’s important to be cautious when using application variables. Objects remain
in application state until you remove them using the Remove or RemoveAll
methods, or shut down the application in IIS. If you continue to save objects
into the application state without removing them, you can place a heavy demand
on server resources and dramatically decrease the performance of your applica-
tions.
Let’s take a look at application state in action. Application state is very commonly
used to maintain hit counters, so our first task in this example will be to build
one! Let’s modify the Default.aspx page that Visual Web Developer created
for us. Double-click Default.aspx in Solution Explorer, and add a Label control
inside the form element. You could drag the control from the Toolbox (in either
Design View or Source View) and modify the generated code, or you could simply
enter the new code by hand. We’ll also add a bit of text to the page, and change
the Label’s ID to myLabel, as shown below:
File: Default.aspx (excerpt)
<form id="form1" runat="server">
<div>
The page has been requested
<asp:Label ID="myLabel" runat="server" />
times!
</div>
</form>
In Design View, you should see your label appear inside the text, as shown in
Figure 5.27.
Now, let’s modify the code-behind file to use an application variable that will
keep track of the number of hits our page receives. Double-click in any empty
space on your form; Visual Web Developer will create a Page_Load subroutine
automatically, and display it in the code editor.
174
Chapter 5: Building Web Applications