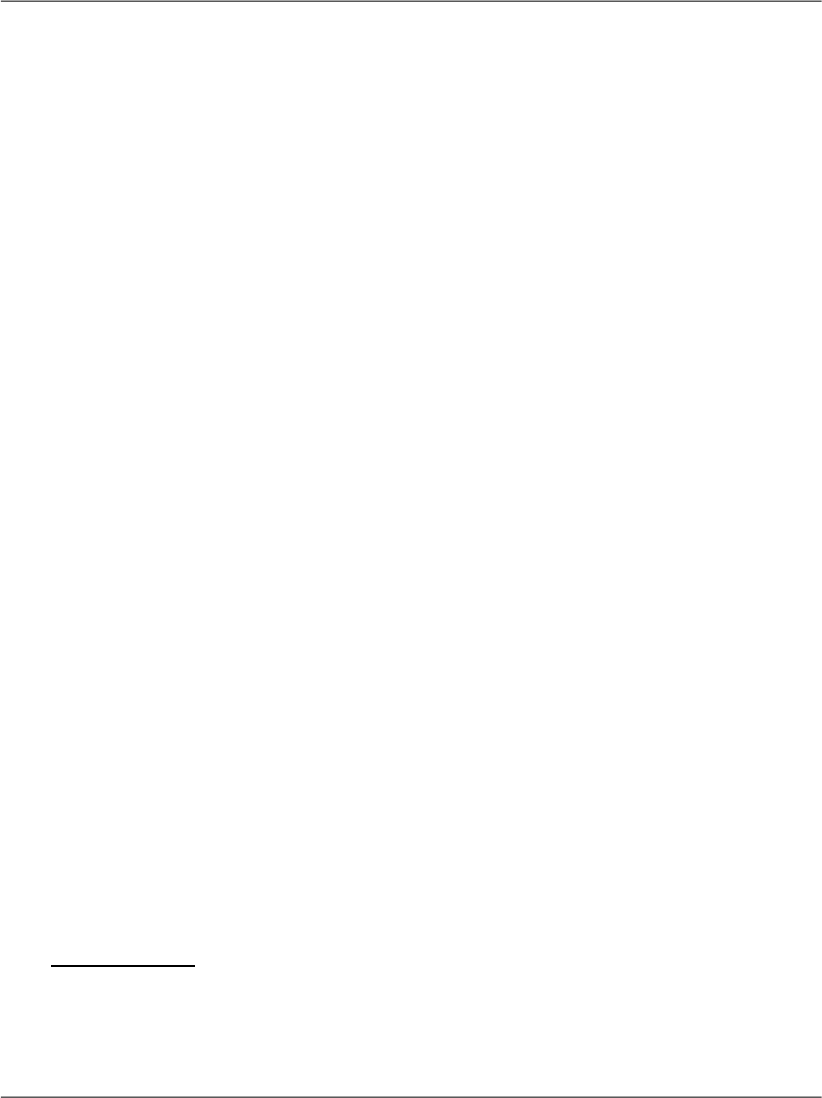
this chapter). We can then use that variable to access features and settings
of the specific control from our subroutine.
e As EventArgs (Visual Basic)
EventArgs e (C#)
This, the second parameter, contains certain information that’s specific to
the event that was raised. Note that, in many cases, you won’t need to use
either of these two parameters, so you don’t need to worry about them too
much at this stage.
As this chapter progresses, you’ll see how subroutines that are associated with
particular events by the appropriate attributes on controls can revolutionize the
way your user interacts with your application.
Page Events
Until now, we’ve considered only events that are raised by controls. However,
there is another type of event: the page event. Technically, a page is simply an-
other type of control, so page events are a particular kind of control event.
The idea is the same as for control events, except that here, it is the page as a
whole that generates the events.
2
You’ve already used one of these events: the
Page_Load event, which is fired when the page loads for the first time. Note that
we don’t need to associate handlers for page events as we did for control events;
instead, we just place our handler code inside a subroutine with a preset name.
The following list outlines the most frequently used page event subroutines:
Page_Init
called when the page is about to be initialized with its basic settings
Page_Load
called once the browser request has been processed, and all of the controls
in the page have their updated values
Page_PreRender
called once all objects have reacted to the browser request and any resulting
events, but before any response has been sent to the browser
2
Strictly speaking, a page is simply another type of control, so page events are actually control events.
But when you’re first learning ASP.NET, it can be helpful to think of page events as being different,
especially since you don’t usually use OnEventName attributes to assign subroutines to handle
them.
56
Chapter 3: VB and C# Programming Basics