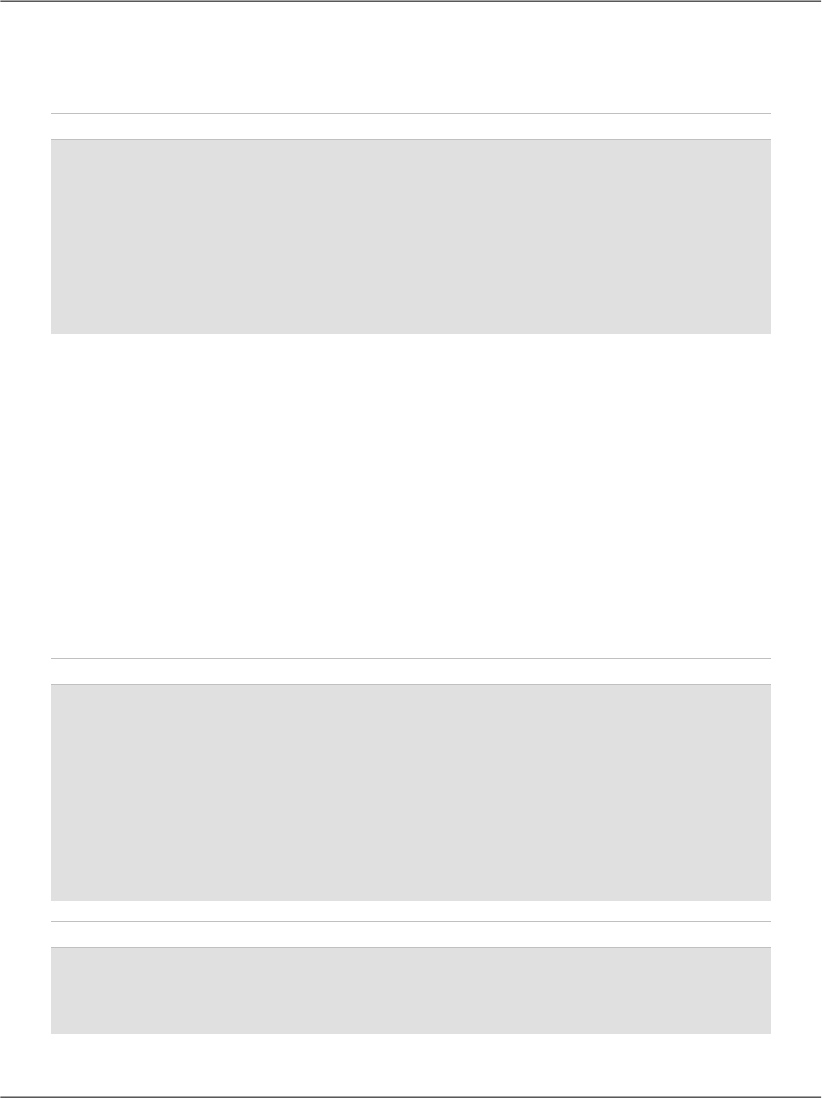
Create a new web form called Departments.aspx, as you have for the other pages
in the Dorknozzle project. Update the generated code like this:
File: Departments.aspx (excerpt)
<%@ Page Language="VB" MasterPageFile="~/DorkNozzle.master"
AutoEventWireup="False" CodeFile="Departments.aspx.vb"
Inherits="Departments" title="Dorknozzle Departments" %>
<asp:Content ID="Content1"
ContentPlaceHolderID="ContentPlaceHolder1" Runat="Server">
<h1>Dorknozzle Departments</h1>
<asp:GridView id="departmentsGrid" runat="server">
</asp:GridView>
</asp:Content>
So far, everything looks familiar. We have a blank page based on
Dorknozzle.master, with an empty GridView control called departmentsGrid.
Our goal through the rest of this chapter is to learn how to use the DataSet and
related objects to give life to the GridView control.
Switch to Design View, and double-click on an empty part of the form to generate
the Page_Load event handler. Add references to the System.Data.SqlClient
namespace (which contains the SqlDataAdapter class), and, if you’re using VB,
the System.Data namespace (which contains classes such as DataSet, DataTable,
and so on) and the System.Configuration namespace (which contains the
ConfigurationManager class, used for reading connection strings from
Web.config).
Visual Basic File: Departments.aspx.vb (excerpt)
Imports System.Data.SqlClient
Imports System.Data
Imports System.Configuration
Partial Class Departments
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles Me.Load
End Sub
End Class
C# File: Departments.aspx.cs (excerpt)
using System;
using System.Data;
using System.Configuration;
using System.Collections;
499
Binding DataSets to Controls