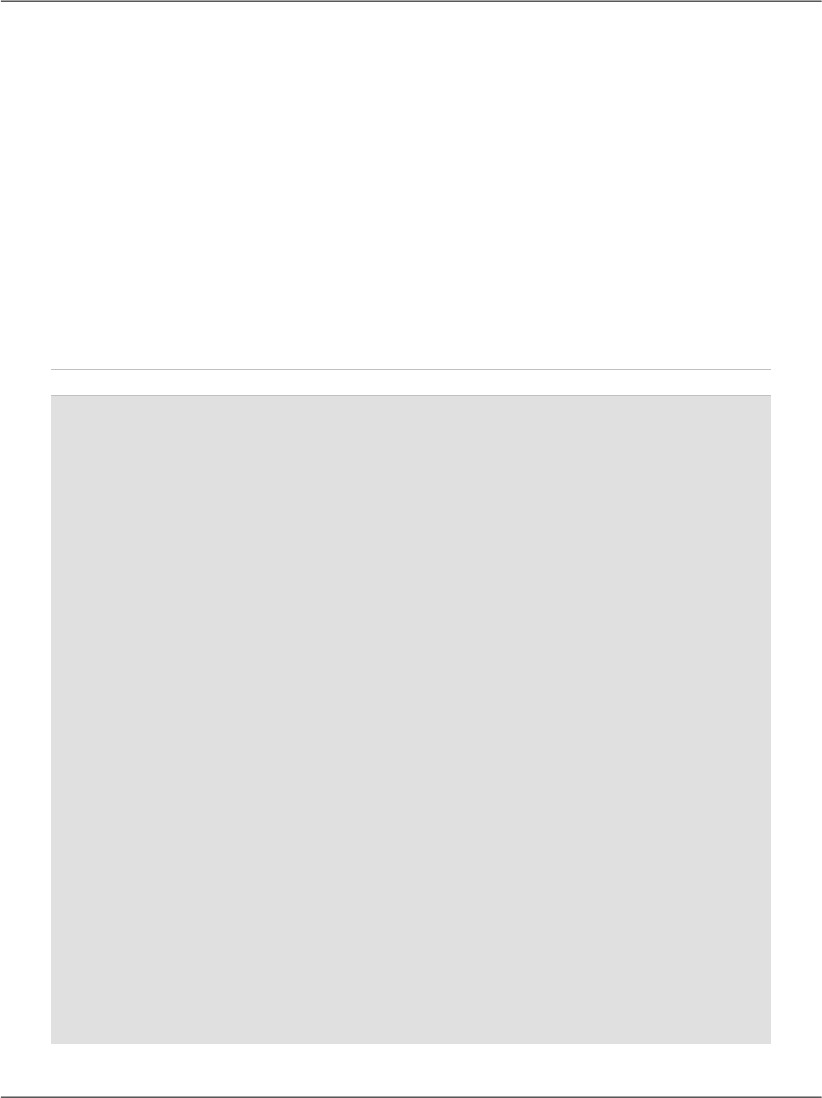
keys, because the GridView supports storing zero, one, or many keys for each
row it displays. In this case, we create an array that contains just one value: Em-
ployeeID. In the code you’ve just written, you can see the syntax that creates
such an array on the fly, without declaring an array first.
After you make this change, you’ll be able to access the EmployeeID value for
any given row through the GridView’s DataKeys property.
With this new data at hand, loading the details of the selected employee into the
DetailsView is a straightforward process. In the GridView’s SelectedIndex-
Changed event handler, we just need to make another database query to read the
details we want to display for the selected employee, then simply feed the results
to the DetailsView object, like this:
Visual Basic File: AddressBook.aspx.vb (excerpt)
Protected Sub grid_SelectedIndexChanged(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles grid.SelectedIndexChanged
BindDetails()
End Sub
Private Sub BindDetails()
' Obtain the index of the selected row
Dim selectedRowIndex As Integer = grid.SelectedIndex
' Read the employee ID
Dim employeeId As Integer = _
grid.DataKeys(selectedRowIndex).Value
' Define data objects
Dim conn As SqlConnection
Dim comm As SqlCommand
Dim reader As SqlDataReader
' Read the connection string from Web.config
Dim connectionString As String = _
ConfigurationManager.ConnectionStrings( _
"Dorknozzle").ConnectionString
' Initialize connection
conn = New SqlConnection(connectionString)
' Create command
comm = New SqlCommand( _
"SELECT EmployeeID, Name, Address, City, State, Zip, " & _
"HomePhone, Extension FROM Employees " & _
"WHERE EmployeeID=@EmployeeID", conn)
' Add the EmployeeID parameter
comm.Parameters.Add("EmployeeID", Data.SqlDbType.Int)
comm.Parameters("EmployeeID").Value = employeeId
' Enclose database code in Try-Catch-Finally
448
Chapter 11: Managing Content Using Grid View and Details View