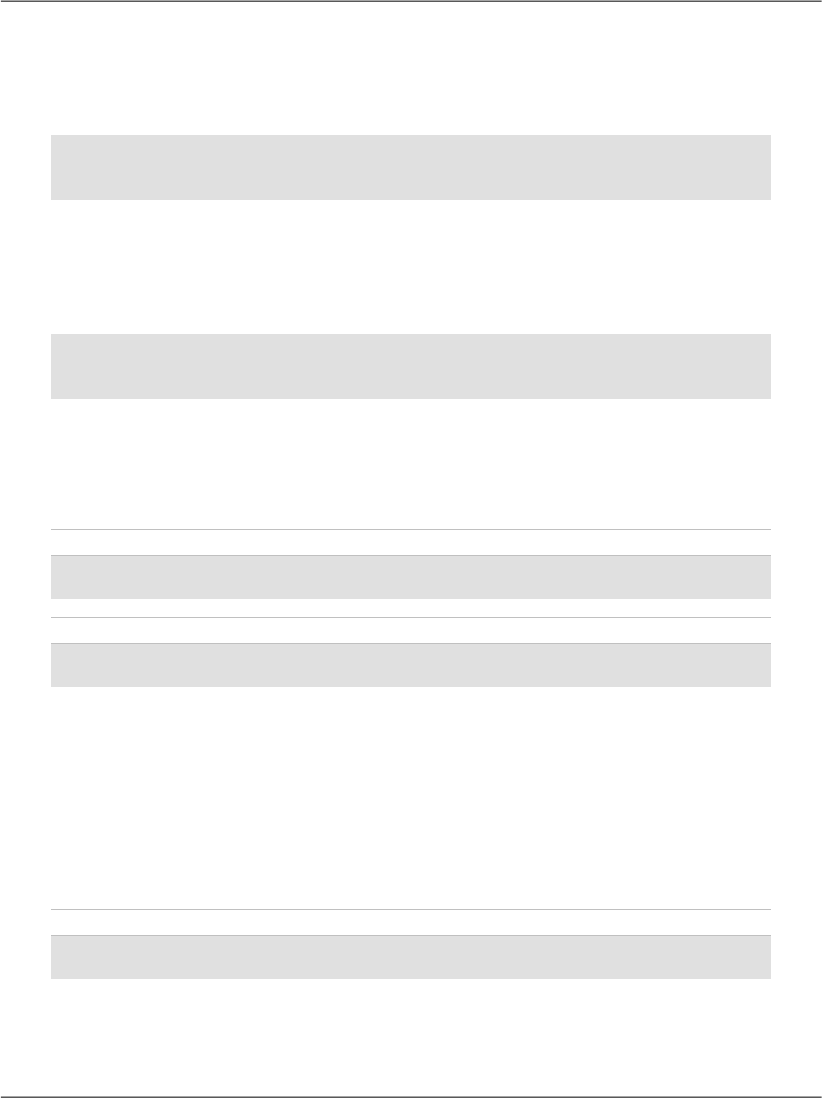
The database would run this query without complaint, and your program would
execute as expected. However, if—as is perhaps more likely—the user entered an
employee’s name, your application would attempt to run the following query:
SELECT EmployeeID, Name, Username, Password
FROM Employees
WHERE EmployeeID = Zac Ruvalcaba
This query would cause an error in the database, which would, in turn, cause an
exception in your web form. As a safeguard against this eventuality, ADO.NET
allows you to define parameters in your query, and to give each of those para-
meters a type. Inserting parameters into your query is a pretty simple task:
comm = New SqlCommand( _
"SELECT EmployeeID, Name, Username, Password " & _
"FROM Employees WHERE EmployeeID = @EmployeeID", conn)
We’ve added a placeholder for our parameter to the query above. To do so, we
add the @ symbol, followed by an identifier for our parameter (in this case, we’ve
used EmployeeID). Next, we need to add this parameter to the SqlCommand object,
and give it a value:
Visual Basic
comm.Parameters.Add("@EmployeeID", System.Data.SqlDbType.Int)
comm.Parameters("@EmployeeID").Value = idTextBox.Text
C#
comm.Parameters.Add("@EmployeeID", System.Data.SqlDbType.Int);
comm.Parameters["@EmployeeID"].Value = idTextBox.Text
Here, we call the Add method of conn.Parameters, passing in the name of the
parameter (EmployeeID) and the parameter’s type; we’ve told ADO.NET that
we’re expecting an int to be passed to the database, but we could specify any of
the SQL Server data types here.
One of the most common SQL Server data types is nvarchar. If your query in-
volved an nvarchar parameter named @Username, for example, you could set its
value with the following code:
Visual Basic
comm.Parameters.Add("@Username", Data.SqlDbType.NVarChar, 50)
comm.Parameters("@Username").Value = username
346
Chapter 9: ADO.NET