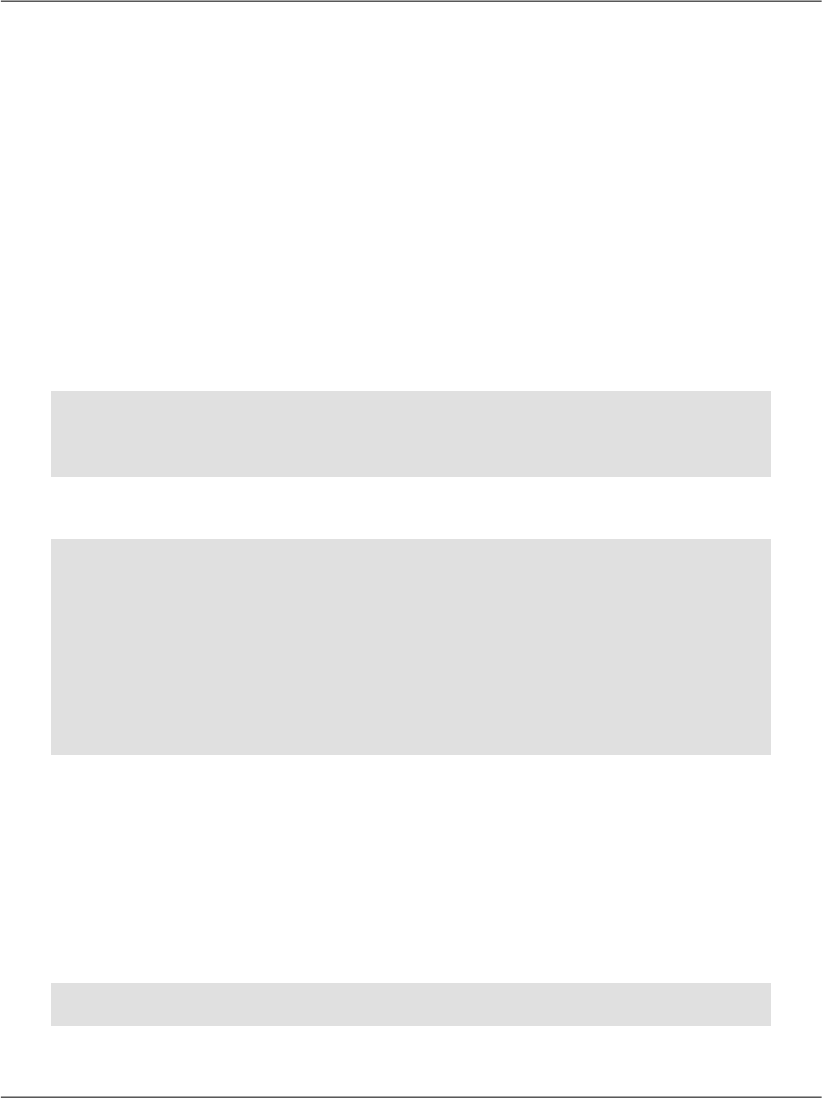
people in your own department on a day-to-day basis. In exactly the same way,
you could always refer to a column in a database using the Table.Column form,
but it’s only necessary when there’s the potential for confusion.
As for the join itself, the code is fairly clear: we’re joining the Departments table
and the Employees table into a single, virtual table by matching the values in the
Departments.DepartmentID column with those in the Employees.DepartmentID
column. From this virtual table, we’re only interested in the names of the employ-
ees whose records match the filter Departments.Department LIKE '%Engineer-
ing'.
By eliminating the WHERE clause and adding the department’s name to the column
list, we could generate a list that contained all the employees and their associated
departments. Try this query:
SELECT Employees.Name, Departments.Department
FROM Departments
INNER JOIN Employees ON Departments.DepartmentID =
Employees.DepartmentID
The results are as you’d expect:
Name Department
-------------------------------- ---------------------------------
Zak Ruvalcaba Executive
Jessica Ruvalcaba Marketing
Ted Lindsey Engineering
Shane Weebe Engineering
David Levinson Marketing
Geoff Kim Accounting
(6 row(s) affected)
Expressions and Operators
In the wonderful world of programming, an expression is any piece of code that,
once evaluated, results in a value. For instance, 1 + 1 is a very simple expression.
In SQL, expressions work in much the same way, though they don’t necessarily
have to be mathematical. For a simple example, let’s create a list that contains
employees and their cities as single strings. Try this query:
SELECT EmployeeID, Name + ', ' + City AS NameAndCity
FROM Employees
310
Chapter 8: Speaking SQL