Chapter 91. Using Object-Oriented Principles in Test Code
Angie Jones
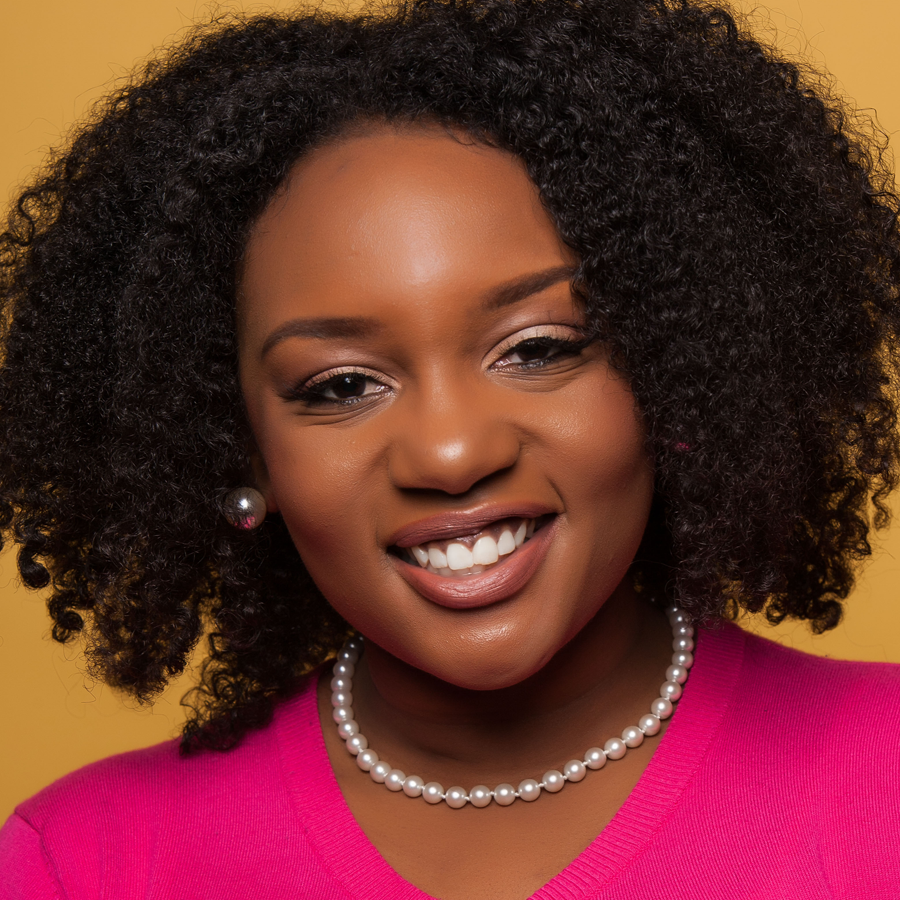
When writing test code, it’s important to exercise the same care that you’d use when developing production code. Here are common ways to use object-oriented (OO) principles when implementing test code.
Encapsulation
The Page Object Model design pattern is commonly used in test automation. This pattern prescribes creating a class to interact with a page of the application under test. Within this class are the locator objects for the elements of the web page and the methods to interact with those elements.
It’s best to properly encapsulate by restricting access to the locators themselves and only exposing their corresponding methods:
public class SearchPage { private WebDriver driver; private By searchButton = By.id("searchButton"); private By queryField = By.id("query"); public SearchPage(WebDriver driver){ this.driver = driver; } public void search(String query) { driver.findElement(queryField).sendKeys(query); driver.findElement(searchButton).click(); } }
Inheritance
While inheritance should not be abused, it can certainly be useful in test code. For example, given there are header and footer components that exist on every page, it’s redundant to create fields and methods for interacting with these components within every Page Object class. Instead, create a base Page
class containing the common members ...
Get 97 Things Every Java Programmer Should Know now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.